21
FebSwitch Statement in JavaScript: Implementation with Examples
Switch Statement in JavaScript
The switch statement is one of JavaScript's most basic decision-making tools. It helps you check multiple conditions in a single block of statements.
In this JavaScript tutorial, we'll explore all the facets of Switch Statements in JavaScript, including syntax, examples, flowcharts, if...else vs. switch statement, etc. If you've just started with JavaScript, you might get confused regarding the application of conditional statements according to the requirements. You'll learn here where to apply switch statements in Javascript. You'll learn here where to apply switch statements in JavaScript. To master these concepts and more, be sure to enroll in our Free Javascript Course for comprehensive learning.
Read More: JavaScript Interview Questions and Answers (Fresher + Experience) |
What is JavaScript switch...case Statement?
The switch...case statement in JavaScript is a type of conditional statement that checks multiple cases for different values of a single expression. It executes one or more blocks of code based on matching cases. The switch statement is similar to a conditional statement containing many else-if blocks, and they can often be used interchangeably.
Flowchart of switch Statement
switch Statement Syntax in JavaScript
switch(expression){
case value1:
//code to be executed;
break; //optional
case value2:
//code to be executed;
break; //optional
......
default:
code block to be executed if any of the cases don't match;
}
The working of the switch statement goes like this:
- It starts with the keyword switch, followed by a set of () containing an expression.
- The expression is evaluated once and compared with the values of each case.
- If any case matches, the corresponding case block executes.
- After the matching case statement is executed, the control exits the switch statement due to the break keyword.
- If the break keyword is not used, the program will check subsequent cases even if a matching case is found.
- If no case matches, the default statement, if present, gets executed.
Remember: It is not necessary to use the default case in JavaScript. It's an optional statement. If the value of the expression doesn't match any case and the default statement is also not present, the entire switch statement is skipped. The program flow goes to the line just after the body of the switch statement.
We'll look at some examples to get a better understanding of the switch statement in JavaScript.
Example illustrating switch...case Statement in JavaScript Compiler
let num = 20;
switch (num) {
case 20:
console.log("It is 20");
break;
case 30:
console.log("It is 30");
break;
case 40:
console.log("It is 40");
break;
default:
console.log("Not 20, 30 or 40");
}
The above code checks if the given number, "num," is 20, 30, or 40. If none of the given numbers exist, the default statement is printed.
Output
It is 20
Multiple Cases
What if various cases in a switch block should have the same output? There may occur some situations where multiple case values must give you the same code block as the result. To accomplish this, we can use more than one case for each block of code.
Example Illustrating Multiple Case Values for a Single Block
let age = 25;
switch (age) {
case 13:
case 14:
case 15:
case 16:
case 17:
case 18:
case 19:
console.log("Adolescent as per WHO")
break;
case 20:
case 21:
case 22:
case 23:
case 24:
console.log("Young Adult");
break;
case 25:
case 26:
case 27:
case 28:
case 29:
case 30:
console.log("Mature Adult");
break;
// when age is none of the above
default:
console.log("Other Age Group");
}
In the above program, we have grouped multiple cases, which allows us to use a single block of code for all the instances in the group. These groups are
- Cases 13, 14, 15, 16, 17, 18, 19: Displays Adolescent on the output screen.
- Cases 20, 21, 22, 23, 24: Displays Young Adult on the output screen.
- Cases 25, 26, 27, 28, 29, 30: Displays Mature Adult on the output screen.
Since 25 matches the first case in the third group (case 25), Mature Adult is printed on the screen.
Output
Mature Adult
Javascript nested switch Statement
The nested switch statement in JavaScript is similar to the nested if statement. It is used to match values with multiple cases.
Example of nested switch Statement in JavaScript
let x = 1, y = 6;
switch (x) {
case 1:
switch (y) {
case 2:
console.log("x = 1, y = 2");
break;
case 6:
console.log("x = 1, y = 6");
break;
default:
console.log("x = 1, y did not match");
}
break;
case 2:
console.log("x = 2");
break;
default:
console.log("x did not match");
}
In the above code:
- The value of x is 1, and hence, the program enters the first switch case.
- Inside case 1, we have another switch block for statement y.
- The value of the y variable is 6, and hence, case 6 of the inner switch is executed.
Output
x = 1, y = 6
Strict Comparison in Switch Statement in JavaScript
JavaScript switch...case statement performs strict type checking of the case values with the value of the expression. The values must be of the same type to match.
let x = 58;
switch (x) {
case "58":
x = "one (string type)";
break;
case 58:
x = "one (number type)";
break;
case 23:
x = "two (number type)";
break;
default:
x = "not found";
}
console.log(`The value of x is ${x}.`);
In the above code, we have assigned an integer value to the variable x.
- case "58" checks if x is the string "58". It is evaluated as false because x is not of string type.
- case 58 checks if x is the integer 58. It evaluates to true since both the type and the value of a match against case 58, thus assigning "one (number type)" to x.
Output
The value of x is one (number type).
Comparison between switch statement and if...else statement
Both are decision-making or conditional or selection statements.
switch Statement | if...else statement |
The expression inside the switch statement determines which case should be executed. | The expression inside an if statement determines whether the sentences in the if block or the else block are executed. |
switch statement looks for equality. | The if-else statement examines both equality and logical expression. |
switch statement evaluates only character or numeric data types. | if...else statement evaluates integer, character, pointer, floating-point, and boolean types |
If there's no break statement after each case, the phrase in the switch statement determines which case to execute by the end of the switch expression. | The statement under the if block will execute first, followed by the statements beneath the otherwise block statement. |
If the expression within the switch statement isn't satisfied, the default code is executed. | If the phrase inside the if block is not executed, the statement inside the else block will be run. |
The switch statement is used when there are multiple cases to match, and the cases are not mutually exclusive | if...else statement is used when there are numerous cases to match. |
Summary
The switch statement in JavaScript is a significant programming construct in the journey of learning JavaScript. We saw the switch statement in complete detail. You need to practice the concepts you learned from this tutorial.
To deepen your understanding and practice the concepts you learned from this tutorial, you can enroll in a Free Online JavaScript Course and enhance your skills further!
You can also read: |
FAQs
- Readability: Clearly organizes multiple conditional branches, improving code readability.
- Maintainability: Easier to maintain and modify when dealing with many conditions.
- Avoids Deep Nesting: Prevents deep nesting of conditional statements, making the code cleaner.
- Direct Matching: Ideal for direct value comparisons, providing a straightforward approach to handle various cases.
Take our Javascript skill challenge to evaluate yourself!
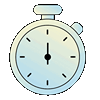
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.