20
DecTernary Operator in JavaScript: Simplifying Conditional Logic
Ternary Operator in JavaScript
The ternary operator in JavaScript is a concise way to perform conditional operations. It's a shorthand for the traditional if-else statement in JavaScript and helps write cleaner, more readable code. Understanding and using the ternary operator in JavaScript is vital because it allows you to make decisions in a single line, enhancing code efficiency and simplicity. Mastering the ternary operator in JavaScript is a great skill for developers looking to improve their coding workflow.
In the JavaScript tutorial, let's explore the Ternary operator in Javascript, including syntax of ternary operator in JavaScript, components of the ternary operator, use cases of the ternary operator in JavaScript, when to use the ternary operator, ternary operator vs. If-else statement in JavaScript, and a lot more.
What is the Ternary Operator in JavaScript
The ternary operator is a JavaScript operator that acts as a shorthand for the if-else statement. It takes three operands, hence the name "ternary."
Syntax
condition ? expressionIfTrue : expressionIfFalse;
- condition: The condition that is evaluated (a boolean expression).
- expressionIfTrue: The result is returned if the condition is evaluated as true.
- expressionIfFalse: The result is returned if the condition is evaluated as false.
Components of the Ternary Operator
The ternary operator in JavaScript consists of three components that enable it to evaluate a condition and return one of two values.
- Conditional Expression
- True (if) Statement
- False (else) Statement
1. Conditional Expression
This is the logical or boolean condition that gets evaluated. If the condition is evaluated as true, the first expression (after ?) is executed. If it evaluates to false, the second expression (after :
) is executed.
Example
let age = 18;
let isAdult = age >= 18 ? "Yes" : "No";
console.log(isAdult);
Output
Yes
In this example, the conditional expression is age >= 18.
2. True (if) Statement
This is the expression that gets executed or returned if the conditional expression evaluates to true.
Example
let age = 18;
let status = age >= 18 ? "Adult" : "Minor";
console.log(status);
Output
Adult
Here, "Adult" is the true statement that executes when the condition age >= 18 is true.
3. False (else) Statement
This is the expression that gets executed or returned if the conditional expression evaluates to false.
Example
let age = 16;
let status = age >= 18 ? "Adult" : "Minor";
console.log(status);
Output
Minor
Here, "Minor" is the false statement that executes when the condition age >= 18 is false.
Use Cases of Ternary Operator in JavaScript
1. Assigning values based on condition
The ternary operator is often used for assigning values based on a condition. This helps in writing concise and readable code.
Example
let age = 18;
let status = age >= 18 ? "Adult" : "Minor";
console.log(status);
Output
Adult
2. Inline conditional rendering of Components
In frameworks like React, the ternary operator is commonly used for inline conditional rendering of components.
let isLoggedIn = true;
let button = isLoggedIn ? Logout : Login;
3. Simplifying if-else statements
Instead of writing multiple lines with an if-else block, the ternary operatorcan perform the same logic in one line.
let score = 85;
let result = score >= 50 ? "Pass" : "Fail";
console.log(result); // Output: "Pass"
Output
Pass
Some Other Examples of Ternary Operators in JavaScript
1. Basic example
let number = 10;
let result = number % 2 === 0 ? "Even" : "Odd";
console.log(result); // Output: "Even"
Output
Even
2. Nested ternary operator
let age = 25;
let category = age < 13 ? "Child" : age < 20 ? "Teen" : "Adult";
console.log(category); // Output: "Adult"
Output
Adult
3. Practical example in real-world scenarios
let userRole = "admin";
let accessLevel = userRole === "admin" ? "Full Access" : "Limited Access";
console.log(accessLevel); // Output: "Full Access"
Output
Full Access
When to use the ternary operator
You should use the ternary operator in some cases where:
- For Simple Conditions: The ternary operator is perfect for handling straightforward, single-condition checks. It keeps your code clean and concise.
- To Make Your Code Concise: The ternary operator is a great choice if you want to reduce the number of lines in your code compared to using a traditional if-else statement.
- When Assigning Values: It's especially useful when you need to decide the value of a variable based on a condition. This makes your code shorter and more efficient.
- For Inline Conditional Rendering: If you're working in frameworks like React, the ternary operator is handy for conditionally rendering components or elements directly within JSX.
- To Improve Readability in Simple Cases: When your condition and expressions are easy to understand, the ternary operator can actually make your code more readable and elegant.
Best Practices of Ternary Operator in JavaScript
1. Readability concerns
While the ternary operator simplifies code, excessive use or nesting can make the code hard to read. Avoid using it when it sacrifices clarity.
2. Avoiding Overuse
It’s important not to overuse the ternary operator in your code. When nested or used for complex logic, it can quickly reduce the readability of your code. Stick to using it for straightforward, simple conditions.
Ternary Operator Vs. If-else Statement in JavaScript
Let's understand the key differences between the Ternary operator and the if-else statement in JavaScript:
Factor | Ternary Operator | If-else Statement |
Syntax | condition ? valueIfTrue : valueIfFalse; | if (condition) { codeIfTrue; } else { codeIfFalse; } |
Conciseness | More concise and fits in a single line. | Requires multiple lines, making it more verbose. |
Readability | Good for simple conditions but can become unreadable if nested. | Suitable for more complex or multi-step logic. |
Use Case | Best for short and straightforward conditional logic. | Both have similar performance in terms of execution speed. |
Execution Speed | Both have similar performance in terms of execution speed. | Easier to handle and understand nested logic. |
Usage in Assignments | Commonly used for assigning values directly. | Requires more lines of code for value assignments. |
Pros and Cons of using Ternary Operator in JavaScript
Pros
- Concise Code: It allows you to write conditional logic in a single line, making your code shorter and cleaner.
- Quick Value Assignment: Ideal for assigning a value to a variable based on a condition without needing multiple lines of if-else.
- Inline Usage: Perfect for frameworks like React, where you can use it directly in JSX for conditional rendering.
- Improved Readability for Simple Conditions: For straightforward logic, it makes the code easier to understand at a glance.
Cons
- Readability Issues: When the ternary operator is used for complex conditions or nested multiple times, it can make the code difficult to read and understand.
- Limited Use for Complex Logic: It’s not well-suited for handling multi-step or intricate logic, where an if-else statement would be clearer and easier to maintain.
Common Mistakes and How to Avoid Them
1. Misuse of nested ternary operators: Nested ternary operators can be difficult to read and understand. When the logic is too complex, it's better to use multiple if-else statements.
2. Lack of clarity in expressions: If the expressions in the ternary operator are complex, the code can be harder to follow. Always prioritize clarity and readability over compactness.
Conclusion
The ternary operator in JavaScript is a powerful tool that simplifies conditional operations, making your code more concise and efficient. However, the ternary operator in JavaScript is important to use judiciously to maintain readability. By understanding its components and best practices, you can enhance your coding skills and use the ternary operator effectively in your JavaScript projects.
Dear learners, if you are interested in certification courses to enhance your skills and knowledge, Scholarhat provides you with the Frontend Developer Certification Training. join our ScholarHat Tech Trendy Masterclasses to clear your doubts and knowledge.
FAQs
Take our Javascript skill challenge to evaluate yourself!
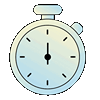
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.