22
DecUnderstanding Local and Global Variables in JavaScript
Local and Global Variables in JavaScript
Local and global variables in JavaScript are important concepts because they provide the knowledge for controlling where data can be used in a program. Knowing how local and global variables in JavaScript work helps developers keep their code clean and organized. This understanding is key for avoiding mistakes and making code work better across different parts of a program.
In this JavaScript Tutorial, prepare yourself to clear your doubts about the 'Variable in JavaScript', 'What is Scope in JavaScript?', 'Global Variables in JavaScript', 'Local Variables in JavaScript', 'Understanding var, let, and const in Scope', and a lot more.
Variables in JavaScript
Like other programming languages, Variable in JavaScript is like a container for storing data values that may be utilized and updated in the code. Variables are defined with keywords like var, let, or const to specify how and where they may be accessed.
- 'var': Declares a variable that is function-scoped (works within the function where it’s defined) and can be updated.
- 'let': Declares a variable that is block-scoped (works within the curly braces {} it’s defined in) and can also be updated.
- 'const': Declares a block-scoped variable that cannot be transferred, which means its value remains constant once set.
Example
let name = "Aman"; // Declares a variable named 'name' with the value "Aman"
const age = 24; // Declares a constant variable 'age' with the value 24
var city = "Paris"; // Declares a variable 'city' with the value "Lucknow"
What is Scope in JavaScript?
Scope in JavaScript refers to the section of code where a variable may be accessed. It specifies whether you may use the variable across the whole program (global scope) or only within a specified function or block (local scope).
How Scope Defines the Visibility and Accessibility of Variables
- Scope in JavaScript controls where a variable can be used in the code.
- Global Scope: Variables declared outside any function/block are accessible everywhere in the program.
- Local Scope: Variables declared inside a function or block are only accessible within that specific area.
- Function Scope (var): Variables declared with var inside a function are only accessible within that function.
- Block Scope (let, const): Variables declared with let or const inside {} are only accessible within that block.
- Scope prevents variables from interfering with each other by keeping them limited to certain parts of the code.
Global Variables in JavaScript
Global variables in JavaScript are those that are declared outside of any block or function and are thus available to the program as a whole. Anywhere in the code, both inside and outside of functions, these variables can be used. However, excessive use of global variables can result in errors and make code more difficult to manage.
Example
let name = "Aman"; // Global variable
function greet() {
console.log("Hello, " + name); // Accessing global variable inside a function
}
greet(); // Output: Hello, Aman
Providing the above example, Thenameis a global variable because it is declared outside of any function. Therefore, it may also be accessed within the greet function.
Output
Hello, Aman
How to Create Global Variables
Global variables in JavaScript are declared outside of any function or block with var, let, or const. When you declare a variable this way, it may be accessed from anywhere in the code, including functions and other blocks.
1. Using var Keyword
Any var variable specified outside of a function becomes a global variable. However, it may mistakenly overwrite other global var variables with the same name.
var color = "blue"; // Global variable using var
2. Using let Keyword
A let variable declared outside a function or block is also global, but it has stricter scoping rules that prevent some common errors.
let theme = "dark"; // Global variable using let
3. Using the const Keyword
A const variable declared outside a function or block is global and cannot be reassigned to a new value. However, if it holds an object or array, its properties can still be modified.
const appName = "MyApp"; // Global constant
Pros of Using Global Variables
- Easily accessible: Can be reached from anywhere in the code.
- Useful for constants: Handy for values that must be consistent throughout the program.
- Convenient for Shared Data: Ideal for data that needs to be shared across many functions.
Cons for Using Global Variable
- Increases the Risk of Errors: If utilized in several locations, it can result in unintended alterations.
- Harder to Debug: Debugging is more difficult since variable changes are more difficult to follow.
- Risk of Name Conflicts: Reduced code modularity can make it more difficult to reuse and maintain.
- Risk of Name Conflicts: Other variables with the same name may be mistakenly overwritten.
Examples of Global variables in JavaScript.
Let's understand global variables with some examples.
Example 1: Global Variable for a Shared Value
let userName = "Aman"; // Global variable
function greetUser() {
console.log("Hello, " + userName + "!");
}
function farewellUser() {
console.log("Goodbye, " + userName + "!");
}
greetUser();
farewellUser();
The variable userName is declared globally outside any function. This makes it accessible to both greetUser and farewellUser functions, which can use the same userName value without needing to pass it as an argument.
Output
Hello, Aman!
Goodbye, Aman!
Example 2: Global Variable for App Configuration
const appName = "MyCoolApp"; // Global constant
function displayAppName() {
console.log("Welcome to " + appName);
}
displayAppName();
Here, appName is a global constant, meaning it can’t be reassigned. It’s accessible throughout the code, and the displayAppName function uses it to display the app's name without needing any arguments.
Output
Welcome to MyCoolApp
Local Variables in JavaScript
A local variable in JavaScript is one that can only be accessed within the function or block of code where it was created. This means you can't access it from outside of that function or block, which helps to keep your code tidy and eliminates accidental variable modifications from other sections of the program.
- If you want to create a local variable in JavaScript, you normally use let or const (or var if it's in a function), and it only exists while the function or block is running.
- Local variables are useful because they prevent conflicts with other variables in your code and make it easy to follow what happens in each function.
Example
function greet() {
let name = "Aman"; // Local variable, only accessible in this function
console.log("Hello, " + name);
}
greet();
console.log(name);
In this code, the name is a local variable inside the greet function, so it can only be accessed and used within that function.
Output
Hello, Alice
Error: name is not defined
How to Create Local Variables in JavaScript
Local variables in JavaScript are declared using let, const, or var inside a function or block (e.g., {} for loops, if statements, functions).
1. Inside Functions
Any variable declared with var, let, or const within a function is specific to that function. This indicates that it can only be utilized within that function and disappears after it is finished.
Example
function greet() {
let message = "Hello"; // Local variable in a function
console.log(message);
}
greet();
console.log(message);
In the above code, the message is a local variable within the greet function, meaning it’s only accessible inside that function and will give an error if accessed outside.
Example
Hello
Error: message is not defined
2. Inside Blocks
Variables declared with let or const within a block (such as an if statement or for loop) are also restricted to that block. However, because var does not create block-scoped variables, it can be accessed outside of the block.
Example
if (true) {
let blockVar = "I’m inside an if block"; // Local to this block
console.log(blockVar);
console.log(blockVar); }
In the above code, blockVar is a local variable within the if block, so it’s accessible only within that block and will cause an error if accessed outside.
Example
I’m inside an if block
Error: blockVar is not defined
Examples of Local variables in JavaScript.
Let's understand local variables with some examples.
Example 1: Function-Scoped Variable
function sayHello() {
let greeting = "Hello, world!"; // Function-scoped variable
console.log(greeting); // Output: Hello, world!
}
sayHello(); // Works inside the function
console.log(greeting);
greeting is a local variable within the sayHello function, so it can only be used inside that function. Trying to access it outside the function will cause an error.
Output
Works inside the function
Error: greeting is not defined
Example 2: Block-Scoped Variable
if (true) {
let message = "Inside the block"; // Block-scoped variable
console.log(message);
}
console.log(message);
message is a local variable within the if block, so it can only be accessed inside that block. Trying to access it outside the block will result in an error.
Output
Inside the block
Error: message is not defined
Understanding var, let, and const in Scope.
In JavaScript, var, let, and const are used to declare variables, but they behave differently when it comes to scope (the area where the variable is accessible).
1. var - Function Scoped
- var is function-scoped, meaning it is accessible within the function where it is declared, and not outside.
- If declared outside of a function, it becomes a global variable.
- var is hoisted to the top of the scope (meaning it is available throughout the function, but it’s initialized as undefined until the declaration line is executed).
Example
function exampleVar() {
var x = 10;
console.log(x); // Output: 10
}
exampleVar();
console.log(x);
Here, x is accessible only inside exampleVar because it is declared using var.
Output
10
Error: x is not defined (function-scoped)
2. let - Block Scoped
- let is block-scoped, meaning it is only accessible within the block (a pair of {}) where it is declared, like inside loops or if statements.
- let is not hoisted like var it stays in the "temporal dead zone" until its declaration is reached.
Example
if (true) {
let y = 20;
console.log(y); // Output: 20
}
console.log(y);
y is only accessible inside the if block, and trying to access it outside that block will result in an error.
Output
20
Error: y is not defined (block-scoped)
3. const - Block Scoped & Constant Value
- const is also block-scoped, just like let, but the difference is that a variable declared with const cannot be reassigned after its initial value is set.
- const is also not hoisted and follows the same hoisting rules as let.
Example
if (true) {
const z = 30;
console.log(z); // Output: 30
}
console.log(z);
z is only accessible inside the block where it is declared, just like let. However, if you try to reassign z, you will get an error.
Output
30
Error: z is not defined (block-scoped)
Note:
If you try to reassign a value to a const variable, it will throw an error.
Example of Reassignment Error
const pi = 3.14;
pi = 3.1415; // Error: Assignment to constant variable.
The value of pi cannot be reassigned after initialization.
output
Error: Assignment to constant variable.
Key Differences
- var: Function-scoped, hoisted, can be re-declared and updated.
- let: Block-scoped, not hoisted, can be updated but not re-declared in the same scope.
- const: Block-scoped, not hoisted, cannot be updated or re-declared (value is constant after initialization).
How Local and Global Variables Interact in JavaScript
In JavaScript, local variables and global variables can interact in various ways depending on where and how they are declared. Here's how they work:
- Global Variables are declared outside any function or block and are accessible anywhere in the code.
- Local Variables are declared inside a function or block and are only accessible within that function/block.
- A local variable can access a global variable, but a global variable cannot directly access a local variable outside the scope of its function/block.
Example 1: Global Variable Accessed by Local Variables
var globalVar = "I'm a global variable"; // Global variable
function example() {
var localVar = "I'm a local variable"; // Local variable
console.log(globalVar); // Output: I'm a global variable
console.log(localVar); // Output: I'm a local variable
}
example();
console.log(globalVar);
Explanation
The global variable globalVar is accessible both inside and outside the function. The local variable localVar is only accessible inside the function example().
Output
I'm a global variable
I'm a local variable
I'm a global variable
Example 2: Local Variable Cannot Access Global Variables Outside Scope
var globalVar = "I am global"; // Global variable
function example() {
var localVar = "I am local"; // Local variable
console.log(globalVar); // Output: I am global
}
example();
console.log(localVar);
Explanation
Inside the example() function, the local variable localVar cannot be accessed outside the function because it is local to that function, while the global variable globalVar is accessible both inside and outside the function.
Output
I am global
Error: localVar is not defined
Example 3: Local Variables Can Override Global Variables
var x = 5; // Global variable
function example() {
var x = 10; // Local variable with the same name
console.log(x); // Output: 10 (local variable overrides global one)
}
example();
console.log(x);
Explanation
The local variable x inside the example() function does not affect the global x. Inside the function, the local x takes precedence, but once outside the function, the global x remains unchanged.
Output
10
5
Key Takeaways:
- Global variables can be accessed anywhere in the code.
- Local variables can only be accessed within the function or block they are declared in.
- Local variables can shadow or override global variables if they have the same name.
Read More: JavaScript Interview Questions and Answers for Fresher and Experienced Candidates
Conclusion
To summarize, local and global variables in JavaScript play an important role in controlling data accessibility and scope within a program. Local variables are restricted to the function or block in which they are declared, whereas global variables are accessible throughout the script. The proper use of both types of variables helps to avoid errors and improve code clarity. Understanding their behavior is critical for developing efficient and bug-free JavaScript programming.
Further Read |
Strings in JavaScript |
Switch Statement in JavaScript |
for Loop in JavaScript |
Map in Javascript |
FAQs
Take our Javascript skill challenge to evaluate yourself!
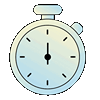
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.