05
JanGetting Started with jQuery UI
jQuery UI will provide you wide range of widget which makes our application more powerful and user friendly. The jQuery UI plugin give you lot more flexibility to play over the widget through the script. Will see the majorly used jQuery UI widget in this article. We can easily add the jQuery UI plugin in our application using the CDN https://code.jquery.com/ui/1.12.0/jquery-ui.min.js
Accordion
Accordion is a widget which is used to expand/ collapse the content. jQuery UI provides different options, events and methods to customize the accordion widget based on the requirement.
HTML
<!DOCTYPE html> <html lang="en" xmlns="http://www.w3.org/1999/xhtml"> <head> <meta charset="utf-8" /> <title></title> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script> <script src="https://code.jquery.com/ui/1.12.0/jquery-ui.min.js" integrity="sha256-eGE6blurk5sHj+rmkfsGYeKyZx3M4bG+ZlFyA7Kns7E=" crossorigin="anonymous"></script> </head> <body> <div id="accordion"> <h3>First header</h3> <div>First content panel</div> <h3>Second header</h3> <div>Second content panel</div> </div> </body> </html> <script type="text/javascript"> $("#accordion").accordion(); </script>
From the above code you can notice I have included Jquery-UI JS next to jquery JS
$("#accordion").accordion() - > will initialize the accordion widget to the div with id “accordion”.
Result
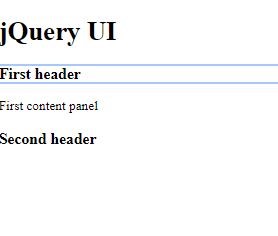
Let’s add the jQuery UI CSS to apply a default theme for the widget. Add below CSS link to the header of the HTML to apply a theme to the widget
<link rel="stylesheet" href="http://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
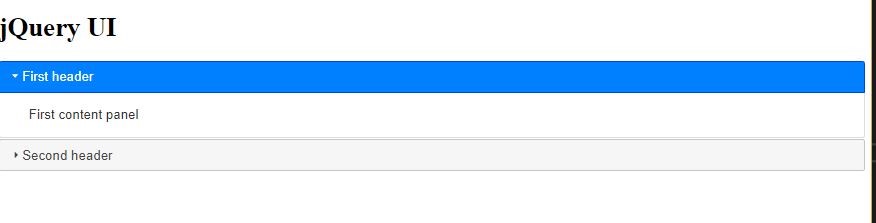
The above result shows the accordion which is applied for the element using jQuery UI.
Options : Mostly used options in accordionRead More - Advanced jQuery Interview Questions and Answers
active
This option is used to define which panel should be opened by default, =>If active –> false, all the panel will be collapsed. By default, first panel will be opened always
HTML
<div id="accordion"> <h3>First header</h3> <div>First content panel</div> <h3>Second header</h3> <div>Second content panel</div> </div>
JavaScript
$( "#accordion" ).accordion({ active: 1 });
The active value is based on the index of the panel which starts from 0, so according to the script the 2nd panel which means index 1 will be opened by default.
Classes
Classes option is used to add CSS class for the widget.
HTML
<html lang="en" xmlns="http://www.w3.org/1999/xhtml"> <head> <meta charset="utf-8" /> <title></title> <link rel="stylesheet" href="jquery-ui.css" /> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script> <script src="https://code.jquery.com/ui/1.12.0/jquery-ui.min.js" integrity="sha256-eGE6blurk5sHj+rmkfsGYeKyZx3M4bG+ZlFyA7Kns7E=" crossorigin="anonymous"></script> </head> <body> <h1>jQuery UI</h1> <div id="accordion"> <h3>First header</h3> <div>First content panel</div> <h3>Second header</h3> <div>Second content panel</div> </div> </body> </html> <script type="text/javascript"> $( "#accordion" ).accordion({ active: 1, classes: { "ui-accordion": "highlight" } });
Result
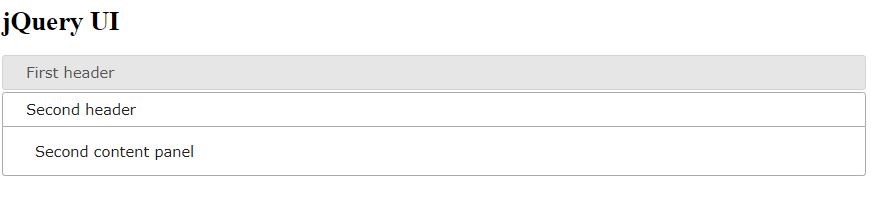
Icons
Icons options is used to add the icons to the accordion
JavaScript
$( "#accordion" ).accordion({ active: 1, icons: { "header": "ui-icon-plus", "activeHeader": "ui-icon-minus" } });
Result
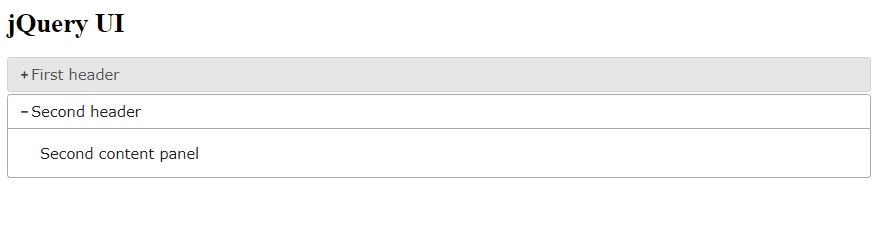
As per the code the + icon is added to the inactive header and – icon is added to the active header
Events
Auto Complete
It will help the user to quickly find and select item from the pre-populated list of suggested values. So basically, the widget will search, and filter the data from the pre-defined list based on what user types in the input field. This widget comes with lot of options, methods and events.
The most commonly used optionsHTML
<div class="ui-widget"> <input id="autoComplete" type="text" /> </div>
JavaScript
$("#autoComplete").autocomplete({ source: ["apple", "orange", "grapes"], autoFocus: true, delay: 500, });
autoFocus
it will focus the first item in the list
Result
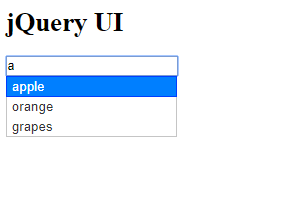
var source = $("#autoComplete").autocomplete("option", "source");
The above code is used to get the value of source
$("#autoComplete").autocomplete("option", "source", ["c++", "java","javascript", "ASP"]);
The above code is used to set the value for source, Like this you can get and set the value for all the options which is available in widget.
Frequently used Autocomplete methodsDatePicker
The datepicker widget in jQuery UI can be enabled using datepicker() function, which is used to display the calendar from where the user can pick the date.
HTML
<div class="ui-widget"> <input type="text" id="datepicker"> </div>
JavaScript
$(function () { $("#datepicker").datepicker(); });
Result
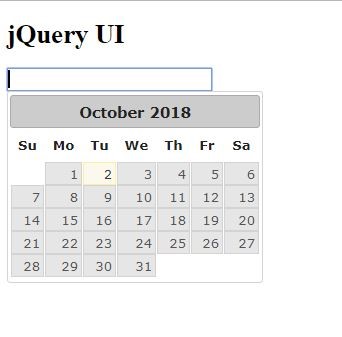
By default, the current date will be selected. jQuery UI provides different option, method and events to the date picker widget will see some of them. There are many options available for datepicker,
the most frequently used options are listed belowHTML
<div class="ui-widget"> <input type="text" id="datepicker"> </div>
JavaScript
$("#datepicker").datepicker({ changeMonth: true, changeYear: true, dateFormat: "yy-mm-dd", duration:"slow" });
Result
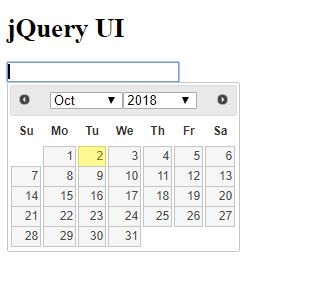
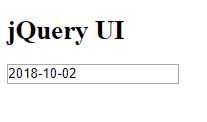
You can notice the month and year dropdown, and when user select the date it will be formatted to the format which is defined in datapicker().
Frequently used Methods in DatePickerNote : There is no event for the datepicker.
Tabs
It is a single content area with multiple panels.
HTML
<div id="tabs"> <ul> <li><a href="#tabs-1">Tab 1</a></li> <li><a href="#tabs-2">Tab 2</a></li> <li><a href="#tabs-3">Tab 3</a></li> </ul> <div id="tabs-1"> <p>Table 1 content.</p> </div> <div id="tabs-2"> <p>Tab 2 content.</p> </div> <div id="tabs-3"> <p>Tab 3 content.</p> </div> </div>
JavaScript
$(function () { $("#tabs").tabs(); });
Result
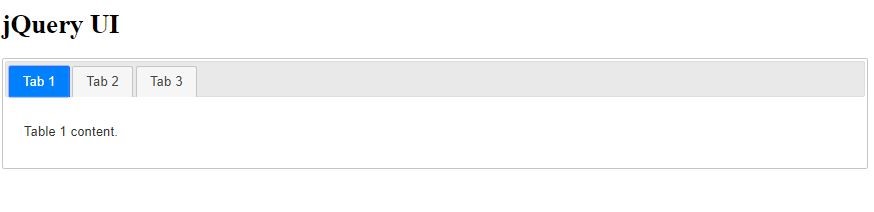
HTML
<!DOCTYPE html> <html lang="en" xmlns="http://www.w3.org/1999/xhtml"> <head> <meta charset="utf-8" /> <title></title> <link rel="stylesheet" href="jquery-ui.css" /> <link rel="stylesheet" href="http://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script> <script src="https://code.jquery.com/ui/1.12.0/jquery-ui.min.js" integrity="sha256-eGE6blurk5sHj+rmkfsGYeKyZx3M4bG+ZlFyA7Kns7E=" crossorigin="anonymous"></script> </head> <h1>jQuery UI</h1> <div id="tabs"> <ul> <li><a href="#tabs-1">Tab 1</a></li> <li><a href="#tabs-2">Tab 2</a></li> <li><a href="#tabs-3">Tab 3</a></li> </ul> <div id="tabs-1"> <p>Table 1 content.</p> </div> <div id="tabs-2"> <p>Tab 2 content.</p> </div> <div id="tabs-3"> <p>Tab 3 content.</p> </div> </div> </html>
JavaScript
$("#tabs").tabs({ active: 1, disabled: [2], event:"mouseover" });
Result
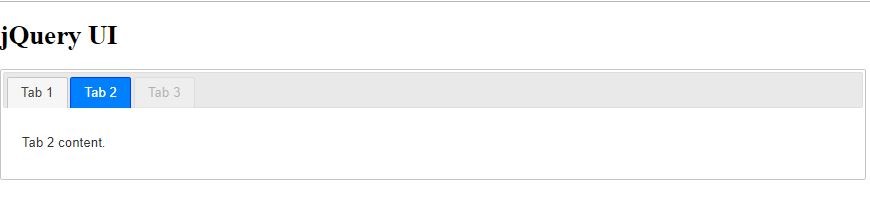
As per the code the 2nd tab is selected by default and the third tab is disabled.
most frequently used methods in Tabs is listed belowdisable()
JavaScript
$("#tabs").tabs({ active: 1, event:"mouseover" }); $("#tabs").tabs("disable");
Result
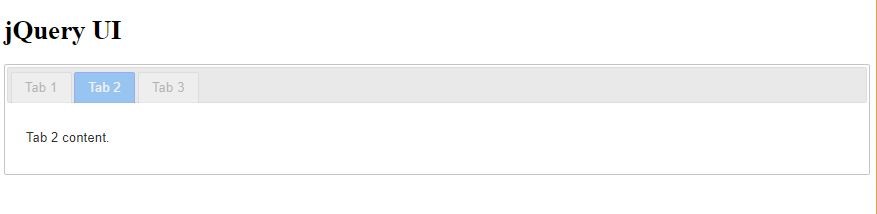
As per the code you can notice all the tab menu are disabled.
The most frequently used events in tabs are list belowTooltip
This tooltip is customizable which can replace the native tooltip. When user mouse over the element the tooltip will be displayed.
HTML
<div class="ui-widget"> <label>First Name: </label> <input type="text" id="txtFirstName" title="Please Enter First Name"> </div>
JavaScript
$(function () { $(document).tooltip(); });
It will initialize the jQuery UI tooltip() for the document
Result
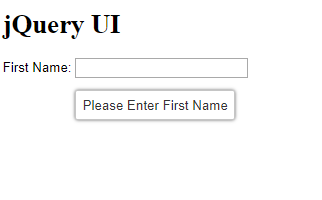
HTML
<div class="ui-widget"> <label>First Name: </label> <input type="text" id="txtFirstName" title="" > </div>
JavaScript
$(document).ready(function () { $("#txtFirstName").tooltip({ content: "Please Enter First Name", position: { at: "right" }, hide: { effect: "explode", duration: 1000 }, }); })
Result
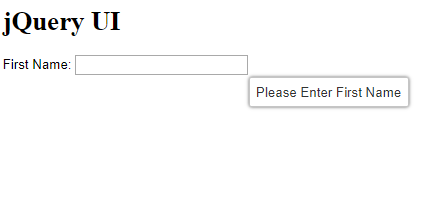
As per the code the tooltip position is aligned to right and the content is loaded from tooltip function
Frequently used methods in jQuery UI TooltipHTML
<div class="ui-widget"> <label>First Name: </label> <input type="text" id="txtFirstName" title="" > </div>
JavaScript
$(document).ready(function () { $("#txtFirstName").tooltip({ content: "Please Enter First Name", position: { at: "right" }, hide: { effect: "explode", duration: 1000 }, close: function (event, ui) { console.log("close event fired") }, open: function (event, ui) { console.log("open event fired") console.log(ui); }, }); })
Result
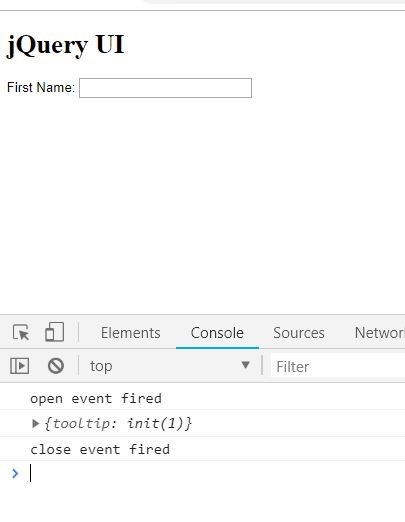
From the above result you can notice the open and close event is fired when we mouse over the field where the tooltip is initialized.
Summary
We have seen how to implement the accordion, datapicker, auto complete, tabs and tooltip widget using the jQuery UI plugin, and different kind of options, methods and events available for each widget which makes it more powerful.