Understanding OOPs Concepts in .NET with Examples
OOPs Concepts in .NET
OOP stands for Object Oriented Programming. It means a kind of programming where everything is represented by an object. Understanding the OOP concepts can greatly enhance your coding skills and make your applications more robust and maintainable.
Read More: Top 50 ASP.NET Core Interview Questions and Answers for 2025 |
What is OOP?
Object Oriented Programming or OOP is a popular way of programming where objects are used to create the programs and applications. In OOP, an object can be anything like a car, person, or even a customer order. The ultimate goal of OOP is to elevate the flexibility and easy refactoring of the code by abstracting real-life entities.
What are Classes and Objects?
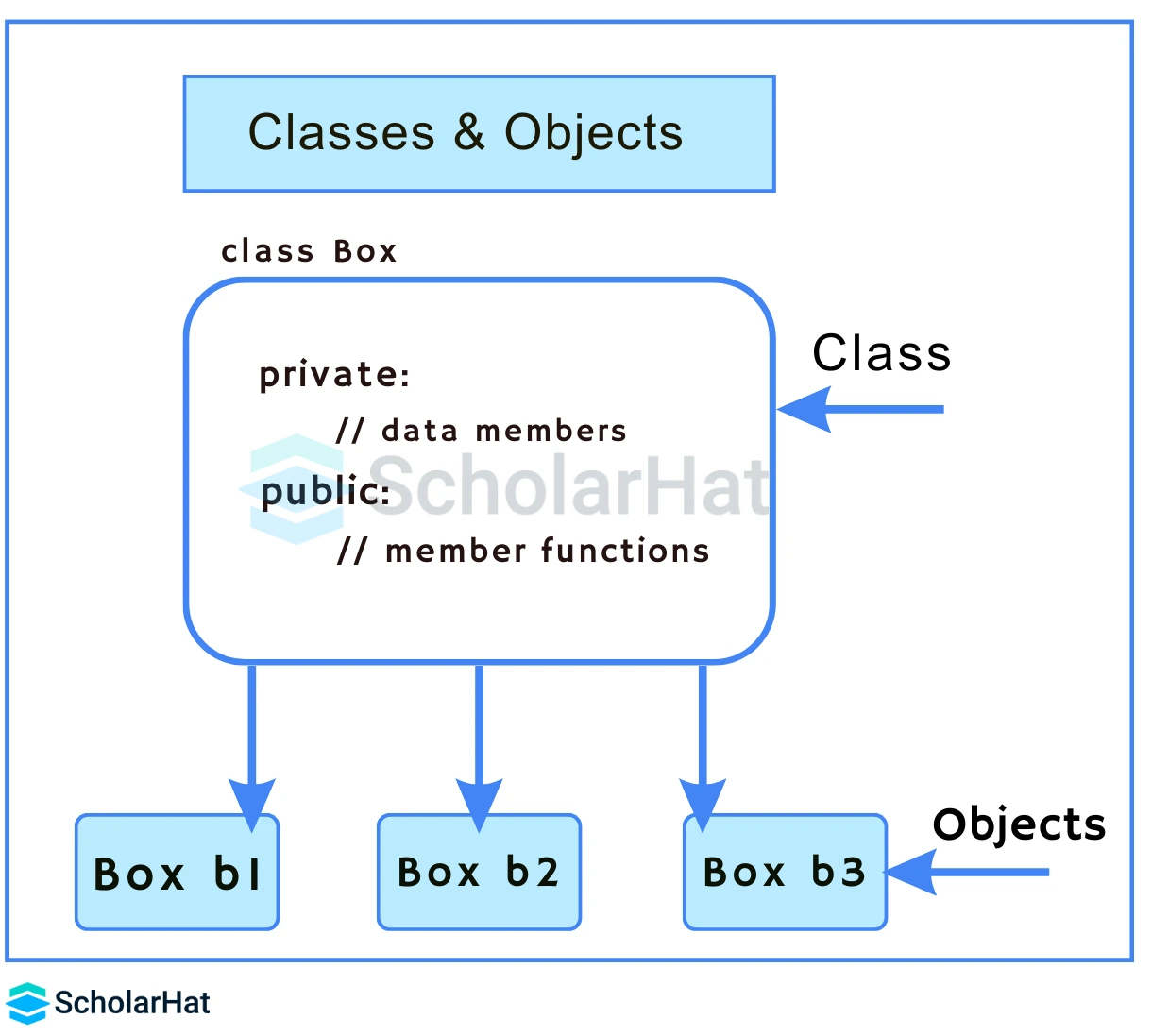
In OOP, a class is the equivalent of an architectural plan to build an object. An object is any empty framework characterized by some properties and behaviors. For example, consider the class Car. It is very probable that this car’s constituting parts could be things such as color or model; it would also include functions such as initiating movement or stopping movement respectively.
An object, however, is an instance of a class. Consider the Car class example: you can instantiate several car objects with different values for the attributes.
public class Car
{
public string Color { get; set; }
public string Model { get; set; }
public void StartEngine()
{
Console.WriteLine("Engine started");
}
public void StopEngine()
{
Console.WriteLine("Engine stopped");
}
}
// Creating an object
Car myCar = new Car();
myCar.Color = "Red";
myCar.Model = "Toyota";
myCar.StartEngine();
The 4 Basic Principles of OOP
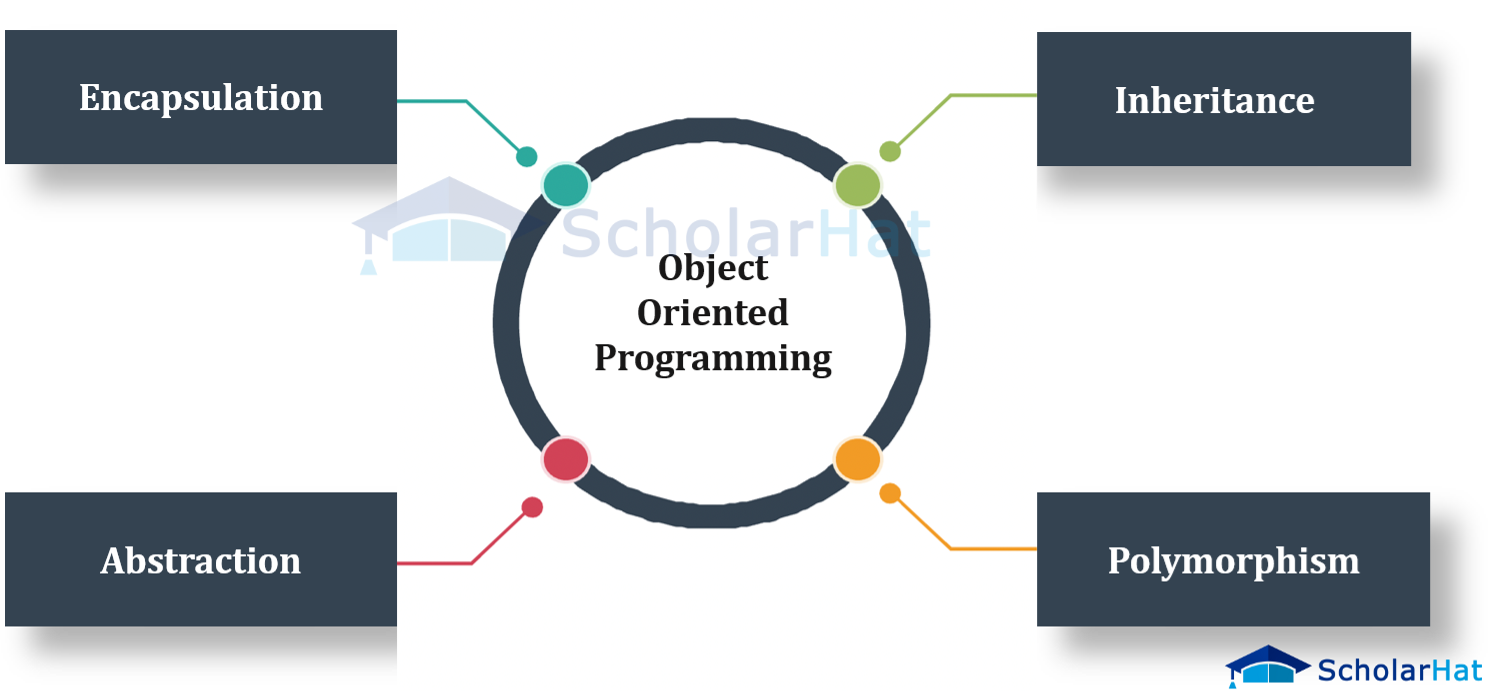
1. Abstraction
public abstract class Animal
{
public abstract void MakeSound();
}
public class Dog : Animal
{
public override void MakeSound()
{
Console.WriteLine("Woof!");
}
}
2. Encapsulation
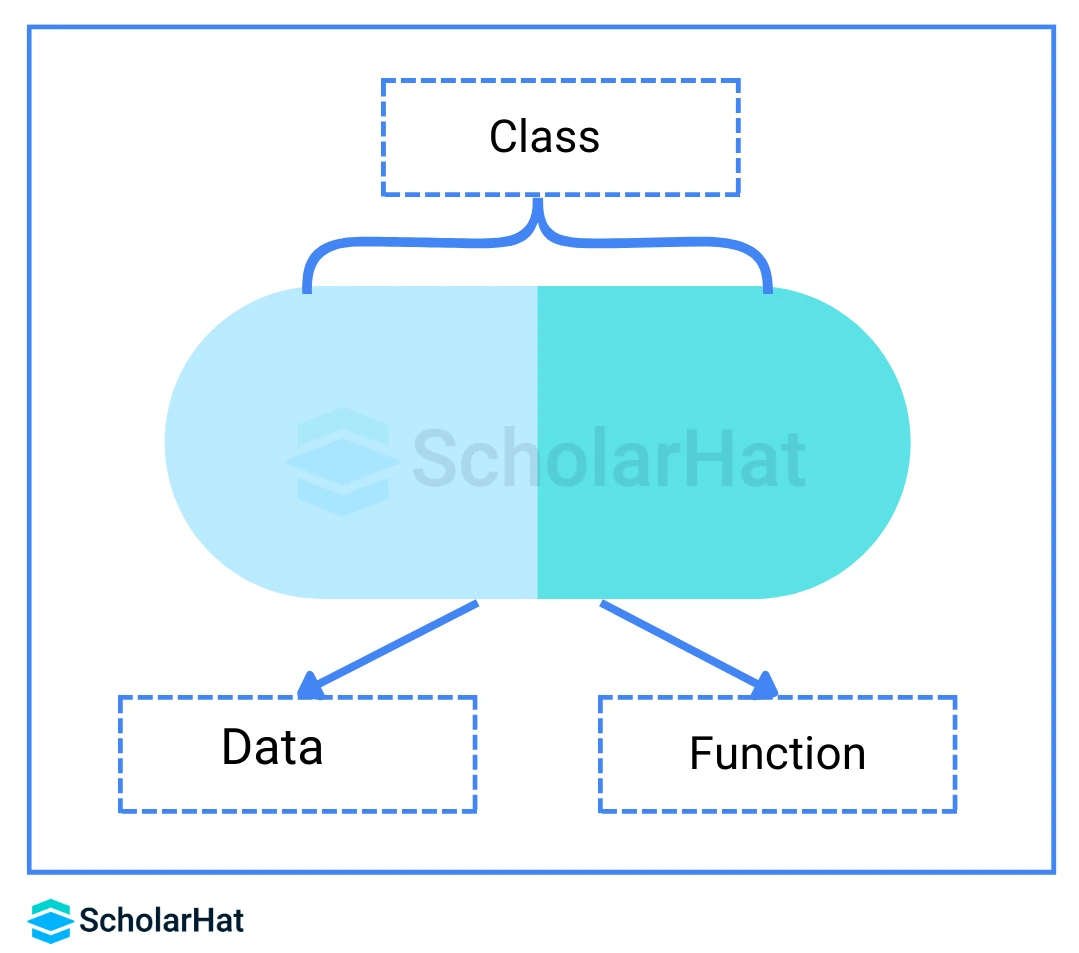
public class BankAccount
{
private decimal balance;
public void Deposit(decimal amount)
{
if (amount > 0)
{
balance += amount;
}
}
public decimal GetBalance()
{
return balance;
}
}
3. Inheritance
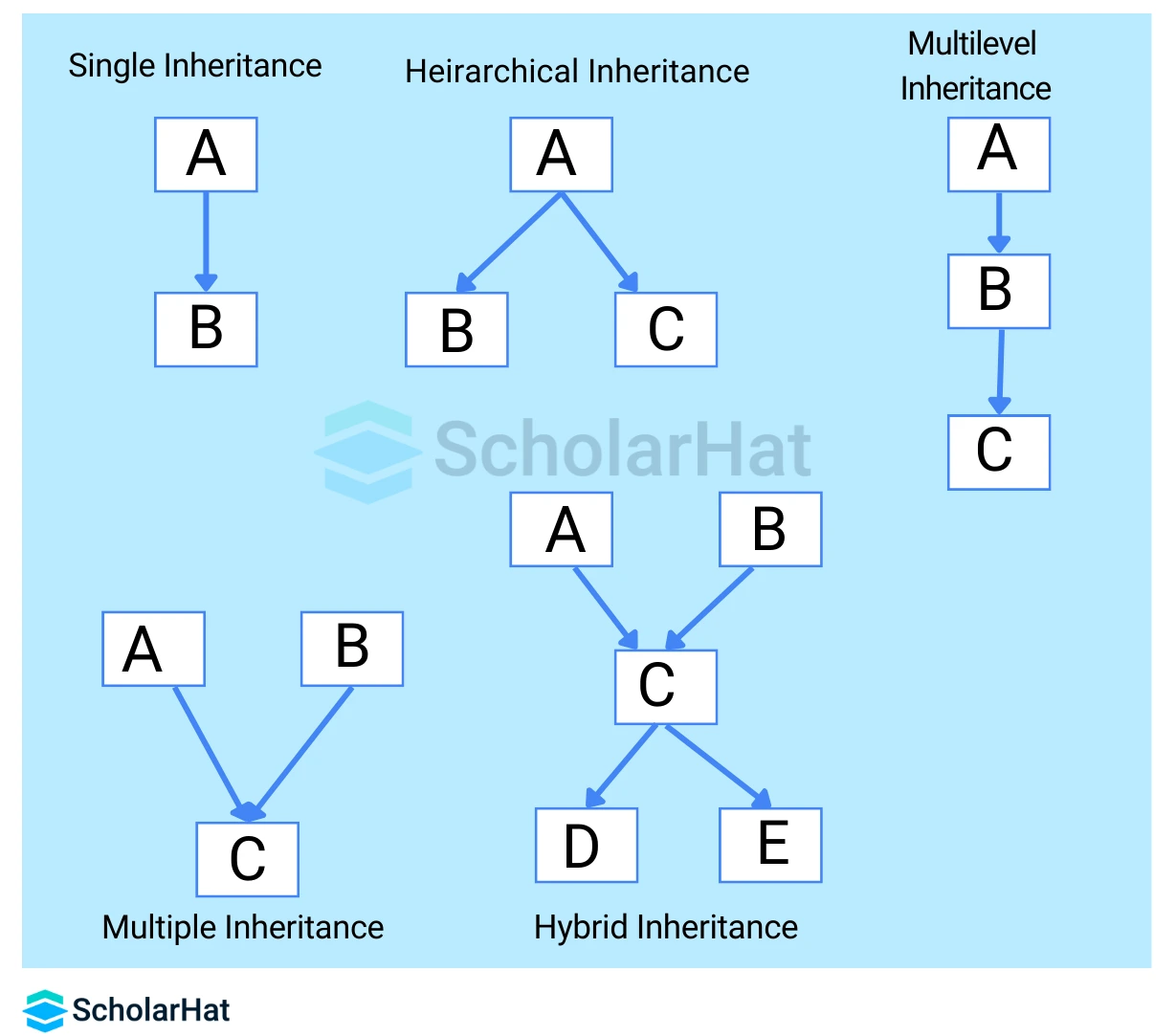
public class Vehicle
{
public string Brand { get; set; }
public void Start()
{
Console.WriteLine("Vehicle started");
}
}
public class Car : Vehicle
{
public string Model { get; set; }
}
4. Polymorphism
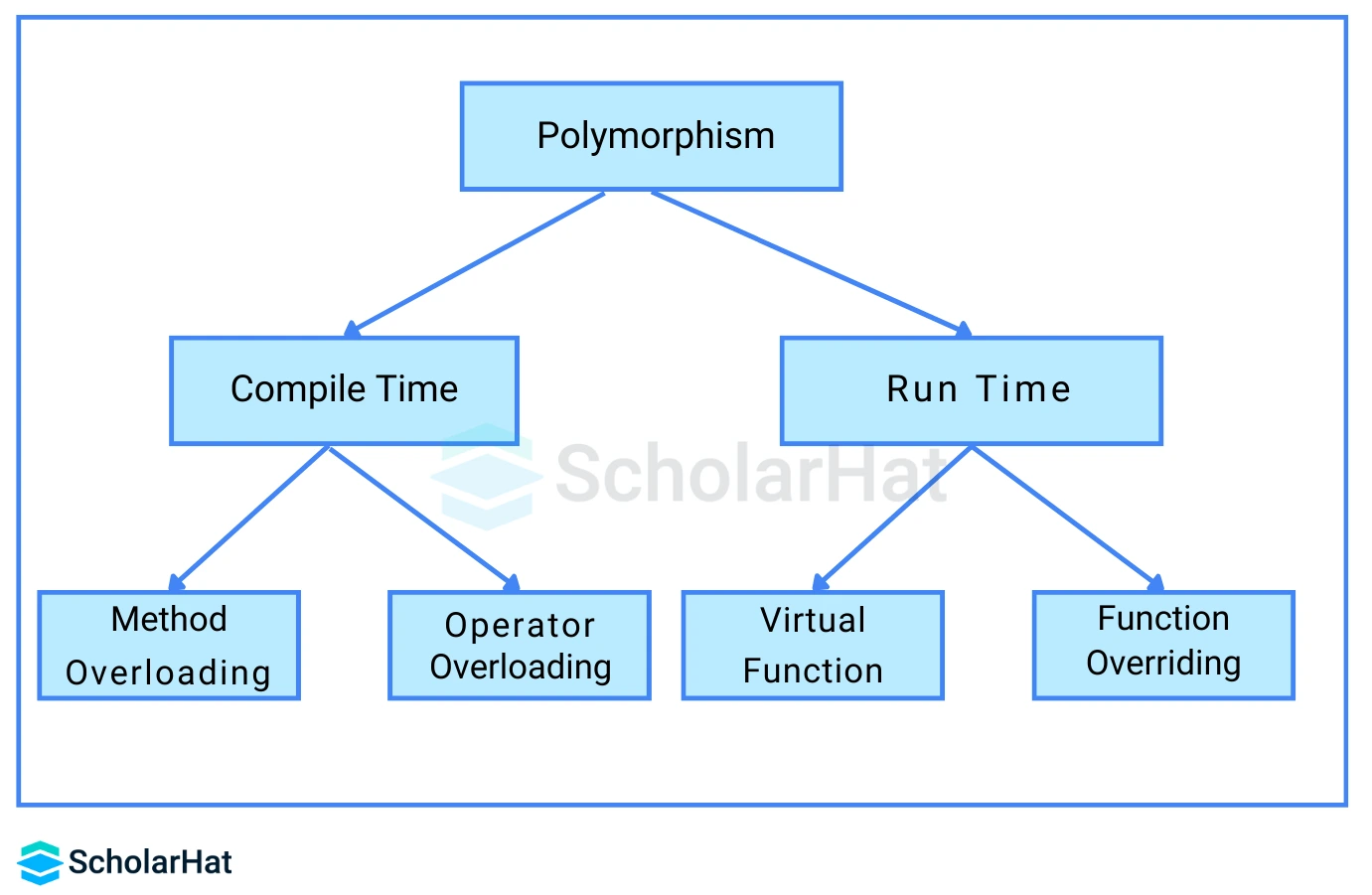
- Compile-time (method overloading)
- Runtime (method overriding)
public class Animal
{
public virtual void MakeSound()
{
Console.WriteLine("Some sound");
}
}
public class Dog : Animal
{
public override void MakeSound()
{
Console.WriteLine("Woof!");
}
}
Read More: Salary Offered to ASP.NET Developers |
The Significance of Composition over Inheritance in .NET
While inheritance is a powerful tool, it can lead to complicated hierarchies and tight coupling between classes. Composition, on the other hand, involves creating objects that contain other objects, allowing for more flexible and modular code. This principle is often summarized as "favor composition over inheritance."
public class Engine
{
public void Start()
{
Console.WriteLine("Engine started");
}
}
public class Car
{
private Engine engine = new Engine();
public void StartCar()
{
engine.Start();
Console.WriteLine("Car started");
}
}
Exception Handling in Object-Oriented .NET Programs
try
{
// Code that may throw an exception
}
catch (Exception ex)
{
// Handle exception
Console.WriteLine(ex.Message);
}
finally
{
// Cleanup code
}
Advanced Topics in OOP
1. Delegates
public delegate void Notify(); // delegate
public class ProcessBusinessLogic
{
public event Notify ProcessCompleted; // event
public void StartProcess()
{
Console.WriteLine("Process Started!");
// Some processing logic
OnProcessCompleted();
}
protected virtual void OnProcessCompleted()
{
ProcessCompleted?.Invoke();
}
}
2. Events
public class Program
{
public static void Main()
{
ProcessBusinessLogic bl = new ProcessBusinessLogic();
bl.ProcessCompleted += Bl_ProcessCompleted; // register with an event
bl.StartProcess();
}
public static void Bl_ProcessCompleted()
{
Console.WriteLine("Process Completed!");
}
}
3. Generics
public class GenericList<T>
{
private List<T> items = new List<T>();
public void Add(T item)
{
items.Add(item);
}
public T Get(int index)
{
return items[index];
}
}
Conclusion
FAQs
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
Take our Net skill challenge to evaluate yourself!
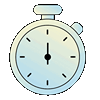
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.