24
JanNode.Js Vs. Express.Js
Difference Between Node.Js and Express.Js
Node.js and Express.js have become vital technologies for developing scalable and efficient web applications. Node.js' autonomous, event-driven architecture has revolutionized server-side JavaScript. Together with Express.js, a compact but powerful framework, they provide a strong basis for dynamic web development.
In this Node.Js tutorial, we will compare Node.js and Express.js's features, benefits, and synergy. Whether you are an experienced developer or a newbie, you will receive practical insights into how to use these tools properly. So let's start with "What is Node.Js ?".
What is Node.Js?
- Node JS is a cross-platform, open-source runtime system for running JavaScript code outside of a web browser.
- Understand that Node.js is not a framework or a programming language.
- Many people get confused and believe it's a framework or a programming language.
- For example, Node.Js is like a car's engine. It does not determine where you go (that is the role of frameworks and programming languages), but it does power the entire system and ensure that it functions properly.
- Before Node.js, JavaScript was mostly utilized for client-side scripting in web browsers.
- Node.js revolutionized the game by allowing JavaScript to be used for server-side programming, allowing you to create full-stack JavaScript apps.
Now we understand what node.js is. Now we will explore the below important concepts
1. Creating a Server in Node.js
To understand how Node.js works, let us begin with a basic example: building a server. Node.js includes a built-in module named http, which makes it simple to build a basic web server.
Example
// Load the http module to create an HTTP server.
const http = require('http');
// Create an HTTP server and listen on port 3000.
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
// The server listens on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
Output
Server running at http://localhost:3000/
Explanation
- This program builds a basic HTTP server with Node.js.
- The server listens on port 3000 for incoming requests and responds with "Hello, World!".
- The HTTP module is used to set up the server and server. The listen() method starts the server and prints a message to the console to show that it is operating.
2. Routing in Node.js
- Routing describes how a server responds to various HTTP requests. Node.js allows you to manage routing directly under the server code.
- Node.js does not come with built-in routing capabilities.
- Instead, developers must manually evaluate each incoming request's URL and method to determine how to handle and react to it correctly.
- This means that routing logic must be implemented in the application code, generally via conditional statements or frameworks like Express.js, which provide more structured routing capabilities.
- By examining the URL and method of each request, developers can dynamically modify replies to the application's individual requirements.
Example
const http = require('http');
const server = http.createServer((req, res) => {
if (req.url === '/') {
res.statusCode = 200;
res.end('Home Page');
} else if (req.url === '/about') {
res.statusCode = 200;
res.end('About Page');
} else {
res.statusCode = 404;
res.end('Page Not Found');
}
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
Output
Home Page
About Page
Page Not Found
Explanation
- This Node.js script creates a basic HTTP server capable of handling several routes (e.g., / for the Home Page and /about for the About page).
- If a user navigates to an unknown route, the server delivers a 404 error with the message "Page Not Found."
- This demonstrates basic routing logic for a Node.js server.
3. Uses of Node.Js
- Building Websites: Node.js is good for constructing websites that require frequent updates, such as chat apps or live notifications.
- Creating APIs: It is used to create APIs that connect various aspects of a system, such as a website's backend and database.
- Single-Page Applications: Node.js is useful for creating websites that load a single page and change dynamically without refreshing.
- Improving SEO: It allows for rendering web pages on the server before they are sent to the user, allowing search engines to better find and rank the site.
- Automating processes: Node.js is helpful for automating processes such as code testing, file compression, and error detection during development.
4. Key Features of Node JS
- Nonblocking I/O: Nonblocking I/O's asynchronous nature allows your applications to handle several requests concurrently, resulting in speedier response times.
- V8 JavaScript Engine: Google's V8 engine, which is noted for its speed and performance, runs JavaScript code quickly.
- NPM (Node Package Manager): NPM simplifies dependency management by giving developers access to a large ecosystem of packages and libraries for their applications.
- Scalability: It supports smooth vertical and horizontal scalability, making it suited for projects of any size.
- Cross-platform: It is compatible with a variety of operating systems, allowing for easy deployment and adaptability.
What is Express.js?
- Express.js is a Node.js web application framework that is both lightweight and flexible.
- It offers a strong set of features that will enable fast development of both web and mobile applications.
- Through Express.js, one can easily handle routing and management of middleware and its integration with database systems to build scalable and high-performance applications.
- It is quite popular and widely used to make it easy to build server-side applications on top of Node.js.
- For example, Think of Express.js as a waiter at a restaurant. The waiter takes your order (the request from the client), serves it to the kitchen (the server), and then gives you back the food when prepared (the response from the server). The waiter takes care of making everything go smoothly and nicely without needing you to do it in the kitchen.
Now we understand what express.js is. Now, we will explore the important concepts below.
1. Creating a Server in Express.js
To understand how Express.js works, consider a simple example of building a server with Express.js. Express.js' simple API makes it easier to construct web servers.
Example
const express = require('express');
const app = express();
// Define a route for the home page.
app.get('/', (req, res) => {
res.send('Hello, World!');
});
// Start the server on port 3000.
app.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
Output
Hello, World!
Explanation
- This code creates a basic web server using the Express.js framework.
- It defines a single route (/) that returns "Hello, World!" when reached.
- The server listens on port 3000, and once operating, you may view the message in your browser by going to http://localhost:3000/.
- Express.js makes it easier to create web servers and manage routes than Node.js' native HTTP module.
2. Routing in Express.js
- Express.jsrouting simplifies implementation and makes it easier to utilize.
- Express's built-in functions allow us to explicitly define route names and associated functions, as well as the kind of request, such as GET or POST.
- This simplified strategy simplifies the development process, increasing productivity while decreasing complexity.
Example
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Home Page');
});
app.get('/about', (req, res) => {
res.send('About Page');
});
app.use((req, res) => {
res.status(404).send('Page Not Found');
});
app.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
Output
http://localhost:3000/:
Home Page
About Page
Page Not Found
Explanation
- This Express.js server script creates routes for the home (/) and about (/about) pages and returns a 404 for all other routes.
- When the server is turned on, it listens on port 3000 and logs a message to the console.
3. Uses of Express.js
Express.js is a framework for Node.js that allows you to build applications and APIs. Some common uses of it are as follows:
- Web Servers: Helps you make servers that deal with HTTP requests and responses so you can develop websites and web applications.
- APIs: It makes it pretty easy to create RESTful APIs, which let different software applications communicate with each other.
- Middleware: Middleware functions can be used to control requests, data processing, and other operations, such as authentication and logging.
- Routing: It eases the process of routing by making it possible to define different routes, which correspond to some URLs, for different pages or actions in the application.
- Error Handling: It provides a way to take control of errors occurring within your application, hence simplifying the debugging and handling of issues.
In other words, Express.js helps you to construct and maintain backends of web applications very effectively.
4. Key Features of Express JS
- Middleware: The Express.js middleware system allows you to enhance your application's request and response handling, making it very customizable.
- Routing: It offers an attractive and intuitive approach for creating routes for handling HTTP requests, making API creation simple.
- Template Engines: It supports a variety of template engines, including Pug, EJS, and Handlebars, which facilitate dynamic content rendering.
- Robust API: It provides a diverse range of HTTP utility methods and middleware, making it ideal for developing APIs of various types.
- Community & Ecosystem: The large Expressjs community ensures that you have access to a variety of libraries and extensions to improve your development experience.
Difference Between Node.js and Express.js
- Node JS is a platform for developing server-side, event-driven I/O applications in JavaScript.
- Express JS is a Node JS framework that allows you to construct web apps utilizing the event-driven architecture of Node JS.
Feature | Express JS | Node JS |
Usage | It's used to develop web-based applications along with the approach and principles of Node JS. | Used to build server-side, input-output, event-driven apps |
Level of Features | More feature-rich than Node JS | Fewer features |
Building Block | Based on Node JS | It runs on Google's V8 Engine |
Written In | JavaScript | C, C++, JavaScript |
Framework/Platform | A framework around Node JS | Run-time platform or environment designed for server-side execution of JavaScript |
Controllers | Controllers are provided | Controllers are not provided |
Routing | It is provided in the routing | It is not provided here |
Middleware | Utilizes middleware to the best of arranging the functions server-side | Does not have that provision |
Coding Time | Less time in coding | Takes more time to code |
Factors to Consider
1. Performance Analysis
- When it comes to performance, both are strong competitors, but they shine in different ways.
- NodeJS has lightning-fast single-threaded performance, making it perfect for I/O-heavy jobs.
- Express, on the other hand, extends Nodejs by adding a layer of abstraction, making it slightly slower but extremely efficient for developing web apps.
- Your decision here is based on your project's specific requirements.
2. Scalability Analysis
- Both are noted for their scalability, which stems from their event-driven architecture.
- The first specializes in creating highly scalable network applications, whilst the latter offers a foundation for developing scalable web applications.
- The key aspect is how successfully your team can leverage these strengths to help your project develop.
3. Test Analysis
- Testing is a critical part of any development process.
- NodeJS and ExpressJS provide robust testing capabilities, with a wide range of modules and tools accessible.
- Node JS offers an advantage in terms of testing libraries. However, Express's simplicity makes it easier to set up and execute tests.
- Your decision will be based on your testing preferences and project requirements.
4. Application Architecture Analysis
- NodeJS provides an effective framework for developing various types of applications, including real-time apps and APIs.
- Express.js, as a web application framework, excels at structuring web applications through its middleware system.
- The decision here is based on whether you require a large application scope or a specialized web application.
5. Microservices Compatibility Analysis
- Microservices architecture is an important concept in modern programming.
- Microservices can be built using either NodeJS or ExpressJS. Node JS, with its non-blocking I/O, can be useful for microservices that communicate with other services.
- The other one simplifies and facilitates the building of individual microservices. The selection is based on your microservices strategy and team skills.
6. Hiring Developers Analysis
- Hiring qualified developers for Node.js vs. Express is generally not difficult due to their popularity.
- Node.js developers are in high demand, and you may locate a wide pool of candidates.
- Express developers, who are typically also NodeJS experts, provide specialist web development services.
- When hiring, keep in mind the scope of your project and its requirements.
7. Database Support Analysis
- Both Node.js and Express offer built-in support for multiple databases.
- NodeJS provides a diverse set of database drivers and libraries, making it adaptable.
- Express, as a web framework, interfaces effortlessly with databases to power web applications.
- Your choice should be based on your preferred database technology and how it fits within your project.
8. Community Analysis
- Node.js and Express both have strong and flourishing communities.
- Node js takes advantage of its widespread use in a variety of domains, while the other has a strong web development community.
- Both communities provide significant resources, lessons, and plugins.
- The decision is based on whether you want a larger or more specialized community to support your project.
When to choose NodeJS or ExpressJS?
When to choose Node.js
- You Need Full Control: When you want to take control of the entirety of your server and how it manages requests and responses.
- Special Requirements: If you are working on something unique that does not fit into the typical web server patterns, such as your own protocol or a special data format.
Example
const http = require('http');
const server = http.createServer((req, res) => {
// Custom data handling
if (req.url === '/custom' && req.method === 'POST') {
let body = '';
req.on('data', chunk => body += chunk);
req.on('end', () => {
res.statusCode = 200;
res.setHeader('Content-Type', 'application/json');
res.end(`Received data: ${body}`);
});
} else {
res.statusCode = 404;
res.end('Not Found');
}
});
server.listen(3000, () => {
console.log('Custom Node.js server running at http://localhost:3000/');
});
Output
Received data: {"key":"value"}
Received data: {"key":"value"}
Output
Not Found
Explanation
- This Node.js script creates an HTTP server that accepts POST requests to the /custom route.
- It collects and processes the data given in the request body before returning the received data in JSON format.
- For any other request, it returns a 404 error message "Not Found." When the server is in operation, it listens on port 3000 and logs a message.
When to Use Express.js
- You Want To Develop Quickly: It makes routine jobs easier, such as routing, handling requests, and managing problems.
- Standard Web Applications: If you're creating a standard web app or API, Express.js has ready-to-use tools and capabilities.
Assume you're building a web app with multiple routes and want to handle failures quickly. Express.js makes it simple.
const express = require('express');
const app = express();
// Middleware to handle JSON data
app.use(express.json());
// Define routes
app.get('/', (req, res) => {
res.send('Hello from Express.js!');
});
app.post('/data', (req, res) => {
res.json({ message: `Received data: ${JSON.stringify(req.body)}` });
});
// Error handling
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
app.listen(3000, () => {
console.log('Express.js server running at http://localhost:3000/');
});
Output
Hello from Express.js!
{ "message": "Received data: {\"key\":\"value\"}" }
Something went wrong!
Explanation
- Choose Node.js if you demand precise control or have specific needs.
- Choose Express.js if you want to create web apps rapidly, with minimal scripting and built-in capabilities.
Popular Applications That Use Node.js and Express.js
Here are some easier highlights of popular apps developed using Node.js and Express.js:
- Netflix: This is for quick and effective movie and show streaming.
- LinkedIn: For a quick and seamless user experience on its website.
- PayPal: Making fast, easy, and reliable online payments.
- Uber: To manage real-time updates for rides and driver locations.
- Walmart: To accommodate a large number of customers, particularly on massive sale days.
- Trello: Real-time updates on project boards.
- Medium: To manage and deliver articles quickly.
- Groupon: Used for managing large deals and users.
- NASA: For a number of tools and systems requiring the processing of a huge amount of data.
- eBay: For real-time messaging and notifications.
These businesses, therefore, depend on Node.js and Express.js to develop applications quickly, in a scalable and robust manner.
Pros of Node.js and Express.js
Fast and Efficient
- Node.js: Uses a non-blocking, event-driven model that does many things at the same time.
- Express.js: Provides lightweight, quick routing for Node.js to make even quicker server responses.
- Node.js: It is an extended JavaScript used to make JavaScript operational on the backend to complement frontend functions.
- Express.js: Integrates seamlessly with Node.js, allowing for the use of JavaScript throughout your stack.
- Node.js: Suitable for dealing with many concurrent connections and is best for big applications.
- Express.js: It helps to simplify the building of scalable web applications and APIs.
- Node.js: Module and library repositories are available, and a large selection is available via npm.
- Express.js: It serves well with most npm modules and middleware that can function to extend its own functionality to Node.js.
Real-Time Capabilities
- Node.js: Ideal for real-time features in heavy applications, such as chat applications and live updates.
- Express.js: This can be implemented because it uses such a robust system to implement real-time functionalities.
- Node.js: Knowledge of JavaScript makes it very easy to learn.
- Express.js: This is a rapid API that's quite simple to help create APIs and web applications.
Single-Threaded
- Node.js: Handles one task at a time per thread, which may be a bottleneck in CPU-heavy operations.
- Express.js: Inherits Node.js's single-threaded nature, so it may not be suitable for computation-intensive applications.
Callback Hell
- Node.js: Juggling many asynchronous operations can result in nested callback hell, which is error-prone and hard to manage.
- Express.js: While it simplifies routing slightly, you can quickly get confused with layers of nested callbacks in middleware.
Less Suitable for Heavy Computing
- Node.js: Not ideal for applications that involve heavy data processing.
- Express.js: Focuses on routing and middleware, not on processing-intensive tasks.
Frequent Updates and Changes
- Node.js: Often has compatibility issues due to rapid evolution.
- Express.js: Regularly updated, which may require changes in the code to stay up-to-date.
Maturity and Stability
- Node.js: Relatively new compared to some other server-side technologies, so many libraries or tools may still be maturing.
- Express.js: While popular, its simplicity can sometimes make it challenging to handle very complex requirements without additional tooling.
Summary
Node.js is an event-driven runtime for server-side JavaScript that provides essential development tools. Express.js is a Node. Js-based lightweight framework that includes routing and middleware to make developing web applications and APIs easier. Node.js is best suited for bespoke solutions that require complete control, whereas Express.js accelerates the development of typical web programs. Together, they provide an effective framework for scalable and efficient web development. Also, consider our Frontend Foundations Certification Training for a better understanding of other web development concepts.
FAQs
Take our Nodejs skill challenge to evaluate yourself!
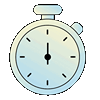
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.