21
NovProps in React
React Props
React's prop-based architecture allows data to be passed across components, resulting in reusable and dynamic components. Parent and child components can communicate by using properties, sometimes known as "props." Declaring and passing props from a parent component to its child component allows us to alter and adjust the behavior and look of the child component based on the received data. Since data is immutable, any kind of data or text, numbers, functions, and so on can be utilized as props. Because of this versatility, developers can create flexible, modular, and interactive user interfaces with React with ease.
So in this React Tutorial, we are going to explore props in React including What are Props in React, its uses, syntax, and Best Practices for working with props. Let's see one-by-one.
What are Props?
- React's core concept of props makes it possible to pass data from one component to another.
- In essence, they are a collection of properties that receive values and are transmitted to child components.
- Similar to passing arguments to a function, think of props as a means to provide your component's parameters.
Read More - Top 50 Mostly Asked React Interview Questions & Answers
How to Declare and Use Props
When a component is rendered in React, an attribute's value is assigned to specify a prop. With the help of props, we are able to build reusable elements that can be rendered with new information each time they are utilized.
Here are some extra considerations regarding props:
- Despite the fact that there may only be one prop, it is always passed as an object.
- The child component cannot modify props.
- Any type of data, including texts, numbers, objects, and arrays, can be passed with props.
Here is an easy example showing how to define and make use of props in React:
Example
import React from 'react';
// Functional component that accepts props
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
// Parent component that renders the Greeting component
const App = () => {
return (
<div>
<Greeting name="John" />
<Greeting name="Jane" />
<Greeting name="Alice" />
</div>
);
};
export default App;
Explanation
- Greeting and App are the two elements in this example.
- The name prop is displayed as a heading by Greeting, and the App creates many Greeting components with various names.
- "Hello, John!" "Hello, Jane!" and "Hello, Alice!" are displayed when the app is rendered.
- Props transfer information and settings between parent and child components.
- In this case, the name prop in Greeting personalizes the greeting.
The Unidirectional Flow of Props
- Props follow a unidirectional flow in React.
- Which means that they are passed from parent components to child components.
- This flow guarantees predictable and controlled data propagation.
- The only thing that child components can do with the props they get is to ingest and display the information.
Read More - React Developer Salary
Props vs. State
- Props & state are both used in React to manage data, although they have different functions.
- The state is used to maintain internal data within a component, whereas props are used to transmit data from parent components to child components.
- Props are read-only and immutable, so children's components cannot change them. State, on the other hand, can be modified asynchronously in response to user or system events and is mutable.
Passing props in reacting and accessing props in react
For creating reusable and dynamic components in React, passing and accessing props is an essential idea. A parent component passes properties, often known as props, to a child component in order to offer data or settings.
Following are the steps for passing props in React and accessing props in React:
- Declare the properties in the child component's props interface. The child component's expected props are defined via the props interface, an object
- Give the child component the prop. Use the props keyword to pass a prop to a child component.
- Utilize the child's component's prop. The props object can be used to access a prop in the child component.
Passing props in react & accessing props in react has the following benefits:
- Reusability: Props make it possible to build reusable parts, cutting down on code duplication.
- Dynamic Behaviour: To create interactive user interfaces, components can render various data and change functionality based on props.
- Communication: Props make it possible for components to communicate with one another, passing information and configuration between them.
- Data Flow Control: Props follow a unidirectional flow, which makes controlling data flow simpler to understand and implement.
- Encapsulation: Props promote the encapsulation of component logic & data, which enhances testability and maintainability.
As an example of passing props in React and accessing props in React, consider this:
Example
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const name = 'John';
const age = 25;
return (
<div>
<h1>Parent Component</h1>
<ChildComponent name={name} age={age} />
</div>
);
};
export default ParentComponent;
// ChildComponent.js
import React from 'react';
const ChildComponent = (props) => {
return (
<div>
<h2>Child Component</h2>
<p>Name: {props.name}</p>
<p>Age: {props.age}</p>
</div>
);
};
export default ChildComponent;
Explanation
This example shows how the ParentComponent produces the ChildComponent while passing the two props, name and age. By using the props object, the ChildComponent can access these props and view their values. "John" is entered as the name and "25" as the age.
Pass data from one component to another
In React, there are three primary methods for passing data from one component to another:
- Props: Passing data from the parent component to a child component via props is the most popular method. Because props are read-only, the data that is sent to the child component cannot be changed.
- State: State is a method of storing data that the component can modify. Although a state can be sent from a parent component to a child component, it is more typical for the child component to use its own state.
- Context: Using context, several components can share data. Context is a more complex way to convey data, but it can be helpful when you need to communicate data among several unrelated components.
Default Props in react
In React, default props in React are a means to establish default values for properties that might not be given in from the parent component. This can be helpful to make sure that even if the parent component doesn't explicitly indicate it, your components always have a value for a specific prop. You can use the default props property on your component class to define default props. Maps the names of props to their default values using this property's object.
Here are some further concerns with default props in react:
- There is no need for default props.
- If there are no default properties that need to be defined, you don't have to.
- The parent component may override default properties.
- A prop's value passed in by the parent component will take precedence over its default value.
- At runtime, default props in react are examined. As a result, rather than the values that were defined when the component was defined, the default props' values will be those that were defined at the time the component was shown.
Here's an example
Example
import React from 'react';
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
// Default props
Greeting.defaultProps = {
name: 'Guest',
};
const App = () => {
return <Greeting />;
};
export default App;
Explanation
In the example, the Greeting component has a default value of 'Guest' for the name prop. When rendering the Greeting component without providing a name prop, it uses the default value. Thus, if you render the App component, it displays the greeting "Hello, Guest!".
Destructuring Props
React offers a simple mechanism to directly destructure props in the function signature in addition to accessing props through the props object. This makes it easier for us to access specific props and makes the code more readable. We can utilize the prop names without having to prefix them with props by destructuring the props.
Here is an example for destructuring props:
Example
import React from 'react';
const Greeting = ({ name, age }) => {
return (
<div>
<h1>Hello, {name}!</h1>
<p>You are {age} years old.</p>
</div>
);
};
const App = () => {
return <Greeting name="John" age={25} />;
};
export default App;
Explanation
The Greeting component in the example uses destructuring to obtain the name and age props directly. This eliminates referencing props.name and props.age a number of times. In order to personalize the greeting, the App component renders the name prop ("John") & age prop (25) parameters. When several props are supplied to a component, destructuring the props makes the code more compact and readable.
Best Practices for working with props:
There are a few best practices to keep in mind when using props in React:
- Use descriptive prop names: To improve the readability and maintainability of your code, give your props concise, meaningful names.
- Document props: Make it simpler for other developers (including yourself) to understand and utilize your components by providing documentation or comments that explain the function and expected values of each prop.
- Destructuring props: You can use destructuring to extract particular props from the function signature of your component. This increases readability and helps your code become more concise.
- Validate props: Ensure that the types and shapes of the props are correct by using prop-types or TypeScript.
- Use default props: Using default props, you may set default values for props. By doing this, you can be guaranteed that your component will work as intended even if some props are not explicitly provided.
- Avoid modifying props: React properties should be viewed as immutable, therefore avoid making changes to them.
- Lift state up: Take into account lifting the state up to a shared parent component if several components require access to the same data or state.
- Use callback props for interaction: Pass callback functions as props from the parent component to the child component if it needs to update data or start an action
Resources for further learning and practice
- Official Redux documentation: Read the documentation at https://legacy.reactjs.org/docs/getting-started.html The official Redux documentation is a comprehensive resource that covers all aspects of Redux, including React Redux.
- Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/reactjs-certification-training. There are numerous online tutorials and courses available that specifically focus on React Redux.
Conclusion
We fully explored the topic of React's props and how to use them to transfer data across components in this extensive guide. Props enable us to build modular, reusable components, improving the maintainability and reuse of our codebase. We can use the power of props to create dynamic and interactive React apps by comprehending the unidirectional flow of props, the distinctions between props and state, and making use of default props and restructuring. To become proficient with this potent library and if you're interested in learning React, think about signing up for the Best React JS Certification Training Course accessible.
FAQs
Take our React skill challenge to evaluate yourself!
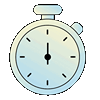
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.