09
NovState in React
Introduction
State in React concept is essential for enabling components to handle and store data. A component's state can vary over time and is represented by it, making it dynamic and interactive. Stateful components, which govern the application's behavior and rendering, are at the core of React.
Any type of data, including characters, numbers, booleans, arrays, and even objects, can be stored in the state. The setState() method can be used to update it after initialization, which sets default values. React forces a re-rendering of the component when the state changes, altering the UI to reflect the new state. If you're looking to enhance your React skills, consider enrolling in specialized React Training Course to deepen your understanding of state management and other advanced concepts.
State in React Components
A default set of values must be declared in the component's constructor to define the state in a React component. The this.state object contains the state's data.
In React, there are two ways to define state:
- Class components
- Hooks
Read More - Top 50 Mostly Asked React Interview Question & Answers
Accessing and Modifying State
The state can be accessed and changed as necessary once it has been defined in a component. In the component's render method or any other method, we can use this.state.propertyName syntax to get access to the state.
We should never update the state directly to change it. Instead, we take advantage of React's setState() method. By doing so, React is informed of the state change, and the component is re-rendered.
Here's an example of how to use setState() to change the state:
import React, { useState } from 'react';
function Example() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const decrement = () => {
setCount(count - 1);
};
const reset = () => {
setCount(0);
};
return (
<div>
<h2>Counter</h2>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
<button onClick={reset}>Reset</button>
</div>
);
}
export default Example;
In this example, the useState hook is used to control the count state variable in a functional component defined as an Example. The component provides buttons for increasing, decreasing, and resetting the count as well as displaying the current count value. When one of these buttons is clicked, the corresponding functions are prompted to use setCount() to update the state, which results in a re-render of the component. Don't forget to export the component for use and import React and useState. This shows how user interactions cause the state to change in a React component.
How to update state in react setState()
In React, updating the state is mostly done via the setState() method. It accepts an object as an argument, and that object should include the state properties' most recent values.
Here are a few guidelines for using setState():
- If the state needs to be modified, do so only then.
- Update the state sparingly; doing so can cause performance issues.
- If necessary, get the value of the prior state using the prevState option.
Read More - React developer salary in India
Conventions of Using State in React
The two state-use conventions in React are as follows:
- The state should never be updated explicitly: This indicates that you want to avoid ever altering a state variable's value directly. Use the setState() function to update the state instead. This function will cause the component to be re-rendered, updating the UI to reflect the new state.
- State updates are independent: This means that when you change the state of one component, the states of all the other components are unaffected. This is due to the separate states that each component has.
Stateful vs Stateless Components
Components in React can be divided into two categories: stateful components and stateless components.
- As the name implies, stateful components have their state and control their data. When necessary, they can re-render and update their state. ES6 classes that extend the React.Component classes are used to generate stateful components.
- Conversely, stateless components have no state and depend only on the props they are given. These simple routines provide back JSX. Stateless elements are frequently referred to as functional elements.
- Stateful components are typically used for complicated components that need state management, whereas stateless components are utilized for specific presentational components.
Difference between state and props
In React, props and state are both used to regulate how components behave and how they are rendered, but they differ significantly in some important ways.
- Data is passed from a parent component to a child component via props, which is short for properties. They are unchangeable, and the kid component is unable to alter them. Child components can have their behavior and look customized via props, which are defined by the parent component.
- On the other hand, the state is utilized to control a component's internal data. It can be changed by the component itself because it is mutable. Event handlers or other component methods typically update the state. Re-rendering of the component is triggered by state changes, altering the UI to reflect the new state.
- While the state is controlled within the component and is not available to other components, props are set by the parent component & given down to child components. As a result, the state is better suited for controlling data and behavior particular to components.
Common Mistakes with State in React
Developers often make specific mistakes when working with the state in React. Let's look at a few of them right now:
- Direct State Modification: One typical error is utilizing this to directly edit the state using this.state.propertyName = newValue. This is wrong and won't cause the component to be rendered again. To update the state, always use the setState() method.
- Making a mistaken use of setState(): Misuse of the setState() function is another error. It's important to keep in mind that setState() may combine several updates and is asynchronous. Use the callback function that is supplied as the second argument to setState() if you need to take some action after the state has been modified.
- Not Using Functional setState(): It's necessary to use the functional form of setState() for dependent state updates. When numerous updates are planned, this guarantees that the right state is used.
- Overusing State: Occasionally, developers tend to save excessive amounts of data in the state, making it challenging to manage and necessitating repeated rendering of the component. The state should only contain the necessary information.
Advanced State Management Techniques
To handle increasingly complex scenarios, React offers several cutting-edge state management solutions. We'll look at a few of them now:
- Using Redux: Redux is a well-liked React state management library that offers a central repository for controlling application state. It makes complex application state management simpler and enables predictable state updates.
- Context API: React has a built-in feature called the Context API that makes it possible to communicate data between components without explicitly giving props at each level. It offers a mechanism to build a global state that any application component may access.
- React Hooks: State and other React capabilities can be used without developing class components thanks to React Hooks, which were introduced in React 16.8. State management is simplified and made more readable via hooks like useState, useEffect, and useContext, which allow functional components to have state and lifecycle functions. If you're looking to dive deeper into React and understand how to leverage the power of hooks effectively, consider enrolling in a specialized React JS Course.
These modern state management methods offer greater flexibility in maintaining the state and can be utilized to handle complex applications with ease.
Best Practices for Working with State
It's necessary to consider a few recommended practices while working with the state in React to create clear and maintainable code. Here are some suggestions to bear in mind:
- Maintain the State Simple: It is best to keep the state as straightforward as possible. A state should not contain complicated data structures or extraneous data. Instead, think about dividing the state into more manageable chunks.
- Set the State's initial value in the constructor: A component's initial state should be defined in the constructor method. This makes sure that the state is set up before any interactions take place.
- Use setState() to Update the State: The setState() function should always be used to update the state. Explicitly altering the state using this.state.propertyName = newValue won't cause the component to re-render and might result in unexpected behavior.
- Avoid Changing State Directly: React's state should be viewed as immutable; avoid directly changing the state. Direct state modification should be avoided because it can result in errors and inconsistencies. Instead, make a new object and provide it to setState() with the modified state values.
- Use the Functional Form of setState() for Dependent State Updates: Use the Functional Form of setState() if you need to update the state dependent on the previous state or other props. When numerous updates are planned, this guarantees that the right state is used.
- Batch numerous setState() Calls: You can group setState() calls together if you need to change numerous state properties. React will streamline the process and execute only one update for all state changes.
Resources for further learning and practice
- Official Redux documentation: Read the documentation at https://legacy.reactjs.org/docs/getting-started.html The official Redux documentation is a comprehensive resource that covers all aspects of Redux, including React Redux.
- Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/reactjs-certification-training. There are numerous online tutorials and courses available that specifically focus on React Redux.
Summary
We have covered all the fundamentals of State in React in this extensive guide. We've looked at the fundamentals of state, how to render data in the UI, how to define the state in components, how to access and modify state, how to update state with setState(), best practices for working with state, stateful vs. stateless components, how to distinguish between state and props, common state management mistakes, and advanced state management techniques.
You should be able to handle state in your React apps and create dynamic, interactive user interfaces after gaining this expertise. Keep in mind to adhere to established practices, prevent common errors, and utilize sophisticated state management approaches when required.
Take our React skill challenge to evaluate yourself!
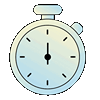
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.