21
FebImplementing Lazy Loading in React
Lazy Loading in React
Lazy loading in React is a powerful method that improves efficiency by loading components only when they are required, rather than all at once. This can significantly shorten the initial load time and improve the user experience, particularly in large-scale apps. As applications increase in size and complexity, managing performance becomes more vital in such cases understanding lazy loading might be helpful.
In this React tutorial, you will get a brief idea about Lazy Loading in React including What Is Lazy Loading?, Why Use Lazy Loading in React?, How Lazy Loading Works in React, Implementing Lazy Loading with React. lazy() and Suspense, Code Splitting in React with Lazy Loading, Key benefits of React Lazy Loading, Challenges in implementing React Lazy Loading, Common Issues with Lazy Loading, and How to Fix Them.
What Is Lazy Loading?
Lazy loading in React is a design pattern that delays the loading of resources or components until they are needed. Lazy loading in React allows you to load components just when they are going to be rendered on the screen, rather than when the app first loads. This reduces the amount of data that the browser must download initially, resulting in speedier load times.
Syntax of Lazy Loading
// Implement Lazy Loding with React.Lazy method
const MyComponent = React.lazy(() => import('./MyComponent'));
Why Use Lazy Loading in React?
Lazy loading in React has several major advantages:
1. Improved Performance
- It minimizes the app's initial load time by loading only the relevant sections first.
2. Efficient Resource Management
- Unused components or resources are not loaded until they are needed, resulting in more efficient use of system resources like memory and bandwidth.
3. Scalability
- Scalability is particularly important in large systems because loading everything at once might be slow and resource-intensive.
Lazy loading allows users to engage with your app more quickly, while secondary or less crucial components are loaded only when needed.
How Does Lazy Loading Work in React
- Lazy loading in React is generally implemented through React.lazy() function, which allows for the dynamic import of components.
- Components wrapped in React.lazy() are only loaded when rendered, not when the application launches.
- React also includes the Suspense component, which handles loading states when a component is being lazily loaded.
- Suspense serves as a fallback method, displaying a loading indicator until the component is finished loading.
Implementing lazy loading using React.lazy() and Suspense
The React. lazy() function allows you to create components that will be loaded asynchronously. Here is a simple example :
Example
import React, { Suspense } from 'react';
// Lazy loading the component
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<div>
<h1>Main Application</h1>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
Key points:
- React.lazy(): This function is used to dynamically import the component (LazyComponent), which will only be imported as needed.
- Suspense: The Suspense component covers the lazily loaded component and displays a fallback UI (such as "Loading...") while it is loading.
This strategy keeps the application's initial load time low by loading non-essential components only when necessary.
Code Splitting in React with Lazy Loading
Lazy loading is frequently used in conjunction with code splitting, which is the process of dividing the software into smaller portions. Splitting the code allows the browser to load only the relevant bits as needed, which improves performance.
In React, code splitting is accomplished by utilizing import() for dynamic imports and tools such as Webpack or Parcel to build smaller bundles.
Example of code splitting
const LazyComponent = React.lazy(() => import(/* webpackChunkName: "lazy-component" */ './LazyComponent'));
Explanation
In this case, Webpack generates a separate bundle for the lazily loaded component. When the component is needed, Webpack retrieves only that exact piece, lowering the application's initial bundle size.Key Advantages of React Lazy Loading
Challenges of Implementing React Lazy Loading
While lazy loading has considerable speed advantages, there are certain problems to consider:- Initial Setup: Configuring lazy loading, particularly in big systems, necessitates careful planning to ensure that components are loaded at the appropriate time without disrupting functionality.
- Handling Loading States: You must control the user experience during the loading phase. If a lazy-loaded component takes too long to load, it may significantly impact the user experience.
- SEO considerations: In server-side rendered apps, lazy loading might have an impact on SEO since search engine bots may not execute JavaScript to load lazy components. Special considerations must be considered in such cases.
Common issues with lazy loading and how to fix them
1. Blank Screen During Load
When lazy-loaded components are fetched, a blank screen may display if there is no adequate fallback UI. Always surround lazy components with Suspense and include a sensible fallback.
Solution:
To retain a nice user experience, utilize a Suspense component with a loading indicator or skeleton screen.
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
2. Lazy Component Fails to Load
If the network is slow or a user disconnects, the lazy component may fail to load, resulting in problems.Solution
To manage errors, enclose your lazy-loaded components with an error boundary.class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
render() {
if (this.state.hasError) {
return <div>Something went wrong.</div>
}
return this.props.children;
}
}
// Usage with Lazy loading
<ErrorBoundary>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</ErrorBoundary>
3. Lack of Support in Older Browsers
Older browsers may not completely support dynamic imports or slow loading.Solution
Use polyfills or verify that your application only uses lazy loading in modern browsers by checking for browser compatibility.4. Long Delays in Loading Components
If your chunks are too large, lazy-loaded components may take a long time to load, frustrating users.Solution
Use tools like Webpack to optimize your bundles and break your code into smaller, more manageable portions.Conclusion
FAQs
Take our React skill challenge to evaluate yourself!
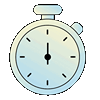
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.