11
JulConquer React with Top 15 Advanced JavaScript Techniques
React Advanced JavaScript Techniques
Advanced JavaScript techniques are like core concepts for React developers. It will improve your capacity to build scalable, effective, and dynamic online applications as a React Developer. So, In order to make your React projects stand out in terms of performance and user experience, you have to learn advanced JavaScript techniques in React.
In this React tutorial, We will see these React Advanced JavaScript Techniques, regardless of your goals of enhancing your component architecture, successfully managing state, or making use of advanced JavaScript techniques.
React Overview
- React is a JavaScript library created by Facebook.
- It is designed to construct user interfaces, for single-page applications that involve frequent data updates.
- It is Recognized for its DOM component-oriented structure and declarative approach.
- React has established itself as a tool in contemporary web development.
- While it's crucial to grasp Reacts principles delving into JavaScript methods is key, to fully leveraging its capabilities.
Why do we need Advanced JavaScript Techniques for React?
- React developers need to have a grasp of JavaScript techniques.
- This knowledge helps in building applications that are efficient easy to maintain and scalable.
- These techniques do not improve the performance of your apps.
- Also, make it simpler to manage complex features, By using these concepts.
- You can write code that's neater and more modular.
Top 15 Advanced JavaScript Techniques with React
1. Higher-Order Components (HOCs)
- Higher-order components are, like functions that accept a component and give back a component making it easy to recycle component logic.
- HOCs come in for concerns such, as tracking, managing errors, and controlling access.
- Let's see pseudocode in JavaScript Compiler.
const withLogging = (WrappedComponent) => {
return class extends React.Component {
componentDidMount() {
console.log('Component mounted');
}
render() {
return <WrappedComponent {...this.props} />
}
};
};
// Usage
const MyComponent = (props) => <div>{props.message} <div>
const MyComponentWithLogging = withLogging(MyComponent);
2. Render Props
- Render Props is a technique for sharing code between React components using a prop whose value is a function.
- This function returns a React element and enables dynamic rendering, promoting component reusability and modularity.
class DataProvider extends React.Component {
state = { data: null };
componentDidMount() {
// Fetch data here
this.setState({ data: 'Fetched Data' });
}
render() {
return this.props.render(this.state.data);
}
}
// Usage
<DataProvider render={(data) => <div>{data}<div>} />
3. Hooks
- In React 16.8 hooks were introduced to enable the utilization of state and various other React functionalities, within components.
- Essential hooks comprise useState, useEffect, useContext, and custom hooks that package logic.
import React, { useState, useEffect } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `Count: ${count}`;
}, [count]);
return (
<div> {count} <button onClick={() => setCount(count + 1)}>Increment</button>
<div>
);
};
4. Context API
- The Context API provides a way to pass data through the component tree without having to pass props down manually at every level.
- It's particularly useful for global state management, such as themes and authentication.
const MyContext = React.createContext();
const ParentComponent = () => {
return (
<MyContext.Provider value="Hello from Context">
<ChildComponent />
</MyContext.Provider>
);
};
const ChildComponent = () => {
return (
<MyContext.Consumer>
{(value) => <div>{value}</div>}
</MyContext.Consumer>
);
};
5. Optimizing Performance with Memoization
- Memoization, achieved through React's React. memo and hooks like useMemo and useCallback, optimizes performance by caching the results of expensive calculations and preventing unnecessary re-renders.
import React, { useMemo } from 'react';
const ExpensiveComponent = ({ value }) => {
const compute = (num) => {
// Expensive computation
return num * 2;
};
const computedValue = useMemo(() => compute(value), [value]);
return <div>{computedValue}</div>
};
6. Error Boundary
- Error boundaries are React components that catch JavaScript errors in their child component tree, log those errors, and display a fallback UI.
- They help gracefully handle exceptions and maintain application stability.
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// Log error info
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>
}
return this.props.children;
}
}
// Usage
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
7. Lazy Loading
- Lazy and Suspense enables you to load components, on demand thus decreasing the loading time and enhancing overall performance.
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
const App = () => (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
8. Immutability and Pure Components
- By maintaining consistency, in how states manage and incorporate components (components that yield identical outputs for the same inputs) we can avoid unnecessary re-renders ultimately enhancing performance.
import React from 'react';
const PureChildComponent = React.memo(({ value }) => {
return <div>{value}</div>
});
// Usage
const ParentComponent = () => {
const value = 'Immutable Value';
return <PureChildComponent value={value} />
};
9. Server-Side Rendering (SSR) and Static Site Generation (SSG)
- SSR and SSG are techniques for rendering React applications on the server or at build time, respectively.
- They improve performance, SEO, and user experience by delivering pre-rendered HTML to the client.
/ Example using Next.js for SSR
import React from 'react';
const HomePage = ({ data }) => {
return <div>{data}</div>
};
export async function getServerSideProps() {
// Fetch data from an API
const data = 'Server-Side Rendered Data';
return { props: { data } };
}
export default HomePage;
10. Using Web Workers
- Web Workers enable us to execute JavaScript code in a background thread from the primary execution thread of a web application.
- This approach can help handle computations and improve the responsiveness of the application.
const worker = new Worker('worker.js');
worker.onmessage = function(event) {
console.log('Data from worker:', event.data);
};
worker.postMessage('Start computation');
11. Optimistic UI Updates
- Optimistic UI updates involve updating the UI before receiving a server response, providing a snappier user experience.
- This technique is particularly useful for interactions where immediate feedback is expected.
const handleClick = async () => {
const oldValue = someState;
updateState(newValue);
try {
await serverRequest();
} catch (error) {
updateState(oldValue);
}
};
12. State Management Libraries
- Tools and libraries such, as Redux, MobX, and Recoil play a role in handling state within applications.
- They offer a method for managing the state, which leads to reliable and expected changes, in the application state.
// Example using Redux
import { createStore } from 'redux';
const reducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
default:
return state;
}
};
const store = createStore(reducer);
store.dispatch({ type: 'INCREMENT' });
console.log(store.getState()); // { count: 1 }
13. Functional Programming Concepts
- Learning about programming principles, like functions, higher-order functions, and immutability can help you write React code that is easier to maintain and cleaner.
const add = (x, y) => x + y;
const multiply = (x, y) => x * y;
const compose = (m, n) => (x) => f(g(x));
const addAndMultiply = compose(multiply, add);
console.log(addAndMultiply(4, 5));
14. TypeScript Integration
- TypeScript, a superset of JavaScript.
- It includes typing can boost the quality of code ease maintenance tasks, and increase developer efficiency.
- Combining TypeScript with React can detect errors at a stage.
- It enhances support, within IDEs.
type User = {
name: string;
age: number;
};
const getUser = (id: number): User => {
return { name: 'Sourav Kumar', age: 22 };
};
const user = getUser(1);
console.log(user.name); // 'Sourav Kumar'
15. Performance Profiling and Optimization
- Using tools such, as React Profiler and Chrome DevTools can assist in pinpointing performance issues within your application.
- Mastering the utilization of these tools has the potential to enhance your application's performance significantly.
import React, { Profiler } from 'react';
const onRenderCallback = (
id,
phase,
actualDuration,
baseDuration,
startTime,
commitTime,
interactions
) => {
console.log(`ID: ${id}, Phase: ${phase}, Actual Duration: ${actualDuration}`);
};
const App = () => (
<Profiler id="App" onRender={onRenderCallback}>
<MyComponent />
</Profiler>
);
Conclusion
To excel as a React developer and create top-notch applications it's crucial to grasp JavaScript methods. Incorporating tools such, as HOCs, hooks, the Context API and Render Props can elevate your React projects. Provide users with experiences. Keeping abreast of these concepts in the evolving React will keep you ahead in web development. Also, take into account our React JS Certification Course for a better understanding of React core concepts.
React Interview Preparation |
React js Interview Questions for Freshers and Experienced |
Top 50 React Interview Questions & Answers |
FAQs
Take our React skill challenge to evaluate yourself!
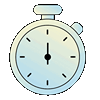
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.