21
NovIntegrating Bootstrap with React
Integrating Bootstrap With React: An Overview
React is a JavaScript-based library that is used to create the interactive user interface. It follows the Virtual DOM concept in which the view will be updated efficiently whenever your application’s state changed. Few advantages to using react.
Declarative
Component-based architecture
Learn once, write anywhere kind of features
Compare to a framework like Angular, in React we don’t have any pre-defined structure, so in React, we can have multiple options to achieve responsiveness of an application. In this React Tutorial, we are going to use Bootstrap with React application to use different components/parts of bootstrap to achieve responsiveness. For that, we need to create a new react application, and in order to create a new react app we need to install react into our machine by using the following npm command.
If you're a newbie to React Development, we recommend you to first understand its basic concepts and usage step by step through React JS Certification Training.
Read More - React Interview Questions And Answers For Experienced
Prerequisites
Node 6.0 > installed
Npm(windows) 5.2 > or Nvm(Linux/macOS)
Any standard code editor like Visual Studio Code, Visual Studio, sublime.
To install react to your machine, we need to run the following npm command.
Npm install create-react-appAfter executing the above npm command, now we will be able to use and create a new react application, let’s start by using bootstrap into our react application, to get started we need to create a new application by using the following npm command.
create-react-app reactwithbootstrapAfter running the above command, our react boilerplate application will be created and you can see the complete information in the power shell as given below.
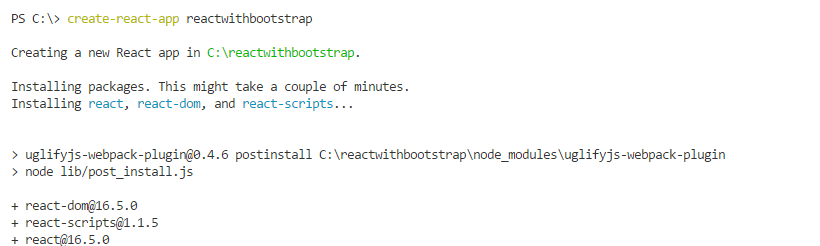
The React app has been created in the specified directory, the next step is to now open the project and there you can see the following application folder structure.
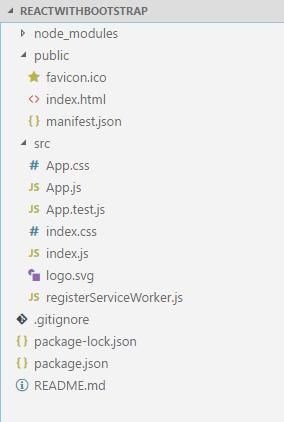
When we create a new application, at a time many important dependencies have been installed by default such as react, react-dom, react-scripts, etc to work with the React library.
After the application configuration, the next step is to integrate the bootstrap using the bootstrap CDNs, in order to use CDN in react app, open public/index.html and add all the required CDNs as given below.
Public/index.html <!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<link rel="manifest" href="%PUBLIC_URL%/manifest.json">
<link rel="shortcut icon" href="%PUBLIC_URL%/favicon.ico">
<!-- Bootstrap CDN -->
<link rel="stylesheet" href= "https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
<title>React App</title>
</head>
<body>
<noscript>
You need to enable JavaScript to run this app.
</noscript>
<div id="root"></div>
</body>
</html>
Read More - React JS Roadmap 2024
Vanilla Bootstrap with React
Let me give you a brief that what is Vanilla bootstrap and what is being used, vanilla bootstrapis just a term that defines that we are using the core bootstrap features without using any additional extensions or plug-ins.
So far we have created a new react application, and then added bootstrap CDNs into an index.html file, the next step we should try the layout of the bootstrap grid into our app, now let’s see a simple example using the bootstrap component.
Open App.js and replace the following code snippet.
import React, { Component } from 'react';
import logo from './logo.svg';
import './App.css';
class App extends Component {
render() {
return (
<div className="App">
<div class="container">
<div class="row">
<div class="col-sm">
One of three columns
</div>
<div class="col-sm">
One of three columns
</div>
<div class="col-sm">
One of three columns
</div>
</div>
</div>
</div>
);
}
}
export default App;
In the above file, multiple bootstrap classes have been used such as container, row,and col-sm and we also have added one class for columns, and to add the stylesheet of the class, we can open the file index.css and add the following style sheet.
Index.css.col-sm
{
background-color: yellow;
height: 100px;
}
Now let’s try to run our react application and see the output.

This is how we have used a simple grid system without using an additional plug-in which is called vanilla bootstrap, but in this case, if we want to use other functionality like navigation bar, toggle panel, etc so for that we need to have jquery to be used in our app, but by just using the vanilla bootstrap we cannot achieve so many functionalities stated above, so for that, we can make use of different another third-party libraries with react.
Third-party Libraries for React and Bootstrap
As we know React is a JavaScript-based library so we have the choice to use structure our application as per our business requirement and also can use a third-party library. There are tons of libraries are available to use with React to implement the Bootstrap-based features or elements in our application, Some of the popular UI frameworks or libraries are given below.
Reactstrap
React-bootstrap
Grommet
Blueprint
Ant design react
Semantic UI React
And many more libraries are available, but in this article, we are going to cover two of them which are popular such as Reactstrap and React-bootstrap.
Reactstrap
Reactstrap is one of the most used with the 50k+ download on weekly basis, mainly reactstrap is used to include and use Bootstrap in our react application. Reactstrap has a large number of components to use with web applications and a few of the components are given below.
Card
Carousel
Form
Breadcrumbs
Modals
Navbar
Tables
Tabs
And many other useful components are available in Reactstrap, let’s implement two of the components into our react application, but before using any of the component, we need to install the reactstrap library in our project, by executing the below npm command.
After executing the above command, you can see that the reactstrap package is installed in our React app.
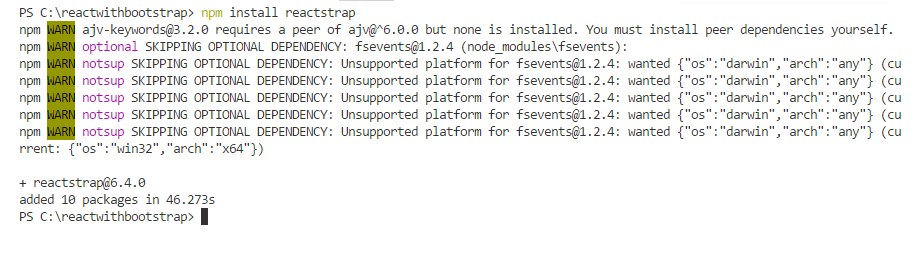
Now we have installed the reactstrap, and our next step is to create a new folder named reactstrap-components and inside the folder, create a new file named Table.jswhere we are going to use an Table element and the code snippet is given below.
/reactstrap-compoents/Table.jsimport React, { Component } from 'react';
import { Table } from 'reactstrap';
class ReactTable extends Component {
render() {
return (
<Table>
<thead>
<tr>
<th>ID</th>
<th>Employee Name</th>
<th>Department</th>
</tr>
</thead>
<tbody>
<tr>
<th scope="row">1</th>
<td>Manav Pandya</td>
<td>Information Technology</td>
</tr>
<tr>
<th scope="row">2</th>
<td>C#Corner</td>
<td>Management</td>
</tr>
</tbody>
</Table>
);
}
}
export default ReactTable;
In the above code snippet of Table.js, we have imported a Table element from the reactstrap package which contains functionality of a native bootstrap table, and in the code snippet, we have created a table along with the structure same as we are using for creating a simple HTML bootstrap table, now let’s import it into app component as described below.
App.jsHere in the App.js file, we need to import the file which was previously created by us named Table.js and use it into the App component for rendering the actual table while running the app, the following code snippet is of App.js.
import React, { Component } from 'react';
import './App.css';
import ReactTable from './reactstrap-components/Table';
class App extends Component {
render() {
return (
<div className="App">
<ReactTable/>
</div>
);
}
}
export default App;
Here in above file, we have used <ReactTable/> markup tag which is used to include an external file as a reusable component and can include conditionally wherever we want in our react application. By executing our project we will get the output as given below.
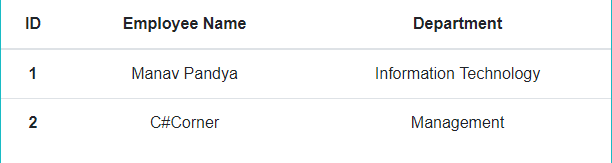
This is how we have developed a reusable component of a bootstrap table, and the same way, let’s create a new File named Card.js to use a Card Component in our application.
/reactstrap-components/Card.jsIn order to use the bootstrap card component, we need to import the card component from reactstrap library and paste the following code snippet inside the newly created file to use multiple card related elements such as Card, CardBody and other components.
import React, { Component } from 'react';
import {
Card, CardImg, CardText, CardBody,
CardTitle, CardSubtitle, Button
} from 'reactstrap';
class ReactCard extends Component {
render() {
return (
<div>
<Card>
<CardImg top width="100%" src="https://dummyimage.com/318x180/000/fff" alt="Image Not Found" />
<CardBody>
<CardTitle>This is card title</CardTitle>
<CardSubtitle>This is card subtitle</CardSubtitle>
<CardText>This is the card text section where you can use different bootstrap element</CardText>
<Button>Click Me</Button>
</CardBody>
</Card>
</div>
);
}
}
export default ReactCard;
Now open app.js, here we are going to import the Card component that we have created as given in above code snippet, and <ReactCard>is used to include the custom card component in order to render the bootstrap card component while running the example.
App.js
import React, { Component } from 'react';
import './App.css';
import ReactCard from './reactstrap-components/Card';
class App extends Component {
render() {
return (
<div className="App">
<ReactCard/>
</div>
);
}
}
export default App;
The next step after creating the card component is to run the above example, and you can see the working example as given below into the browser.
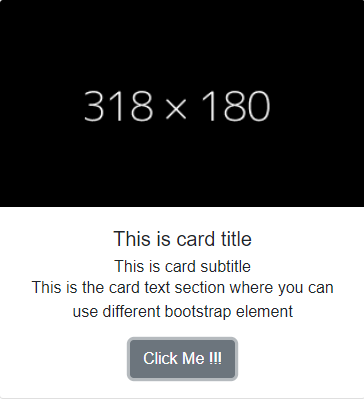
So far we have learned the what is the reactstrap, and what is is used, how to install it, and use their different bootstrap components in our react application, you can try other different components as well according to the choice.
React-bootstrap
React-bootstrap is a powerful front-end framework or a UI library that is used to create a responsive layout with a React; the react-bootstrap is a rebuilt version or a wrapper of the native bootstrap framework.
Currently, react-bootstrap supports v5 (while writing this guide) and it has the support of version 4 and 3 as well, so you can use many components which are included in version 4 of bootstrap. In order to use the react-bootstrap with react, we need to install it using the following npm command.
Npm install react-bootstrap
After successful installation of a package, in console, you can see the output as given below.

Apart from the package installation, we need to add an additional theme or stylesheet file into /public/index.htmlas given below.
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap-theme.min.css" integrity="sha384-rHyoN1iRsVXV4nD0JutlnGaslCJuC7uwjduW9SVrLvRYooPp2bWYgmgJQIXwl/Sp"
crossorigin="anonymous">
After the installation and stylesheet configuration, now we can use all the components of the react-bootstrap and some of the frequently used components are mentioned below.
Carousel
Jumbotron
Modal
Table
Breadcrumbs
Navbar
Forms
Jumbotron
Carousel
Jumbotron.js
Carousel.js
And many more components are included within react-bootstrap, in this tutorial, we are going to use two different react-bootstrap components as mentioned.
For that create one new folder under the src folder named react-bootstrap and create two different js files for the each components.
In this file, we are going to import the jumbotron component from the react-bootstrap package just like the below code snippet.
import React, { Component } from 'react';
import { Jumbotron , Button } from 'react-bootstrap';
class JumbotronDemo extends Component {
render() {
return (
<div>
<Jumbotron>
<h1>React-bootstrap Jumbotron</h1>
<p>
This is the simple example which shows the simple demo for Jumbotron of react-bootstrap
</p>
<p>
<Button bsStyle="primary">Click Me</Button>
</p>
</Jumbotron>
</div>
);
}
}
export default JumbotronDemo;
In this file same way, we need to import the carousel component from a react-bootstrap package like this.
import React, { Component } from 'react';
import Carousel from 'react-bootstrap/lib/Carousel';
class CarouselDemo extends Component {
render() {
return (
<div>
<Carousel>
<Carousel.Item>
<img width={900} height={500} alt="900x500" src="https://dummyimage.com/900x500/000/fff" />
<Carousel.Caption>
<h3>Slide 1 Label</h3>
<p>This is the slide 1 description</p>
</Carousel.Caption>
</Carousel.Item>
<Carousel.Item>
<img width={900} height={500} alt="900x500" src="https://dummyimage.com/900x500/000/fff" />
<Carousel.Caption>
<h3>Slide 2 Label</h3>
<p>This is the slide 2 description</p>
</Carousel.Caption>
</Carousel.Item>
<Carousel.Item>
<img width={900} height={500} alt="900x500" src="https://dummyimage.com/900x500/000/fff" />
<Carousel.Caption>
<h3>Slide 3 Label</h3>
<p>This is the slide 3 description</p>
</Carousel.Caption>
</Carousel.Item>
</Carousel>
</div>
);
}
}
export default CarouselDemo;
As you can see that we have included the code snippet of Carousel with other different components related to Carousel.
Carousel
Carousel.item
Carousel.caption
Which is used to provide separate slides’ data as well as their appropriate captions based on the layout requirements. Now we need to include these two components in our main component which is App.js, and to render both components, we need to import those re-usable components in App.js file as given below.
import React, { Component } from 'react';
import './App.css';
import Jumbotron from './react-bootstrap/Jumbotron';
import Carousel from './react-bootstrap/Carousel';
class App extends Component {
render() {
return (
<div className="App">
{/* Using Jumbotron */}
<Jumbotron />
{/* Using Carousel */}
<Carousel />
</div>
);
}
}
export default App;
Here in this code snippet, we have used two import statements of the reusable components in order to render their content within the main component file, now save it and execute the application, and after running the application, our page may looks like as given in the below snapshot.
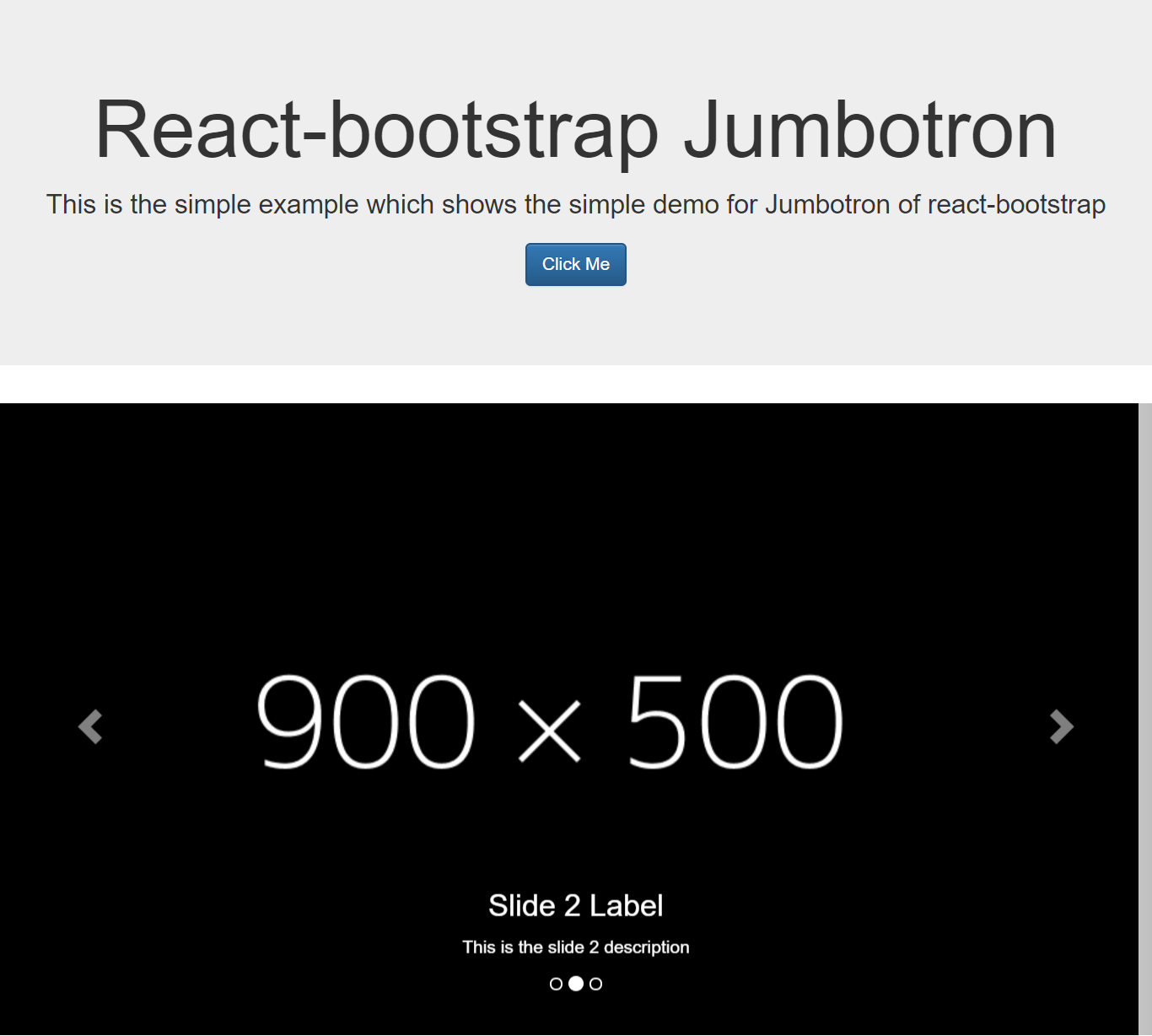
In the output, you can see that both two of our components are rendered as expected with appropriate data along with the bootstrap themed UI; and this is how we can use variety of react-bootstrap components in our react application. I would also recommend you to use other various components which are provided by react-bootstrap and create some amazing stuff out of it as an exercise.
Summary
This guide is all about using Bootstrap with React along with other third-party libraries, alongside, we have covered all the necessary steps by which we learned that how to use different bootstrap components into our react application, their different third-party libraries and their component, how to configure and use them inside the application, bootstrap can provide any website a decent look and feel, I would surely recommend you to go through all the examples and apply multiple components into your react application. Happy learning.
FAQs
- Bootstrap's CSS file can be manually imported into your project.
- You can use the React-Bootstrap library.
- You can also use other UI libraries that are built on top of Bootstrap.
Take our React skill challenge to evaluate yourself!
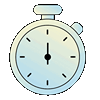
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.