11
JulReact Redux Interview Questions: Crack Your Next Interview in 2025
React Redux Interview Questions are essential for developers preparing for frontend and full-stack roles. React is a popular JavaScript library for building user interfaces, while Redux is a state management library that helps manage application states efficiently. To ace a React Redux interview, you should understand concepts like actions, reducers, stores, middleware, hooks, and asynchronous data handling.
In this React tutorial, we provide a list of commonly asked React Redux Interview Questions with detailed answers. Whether you're a beginner or an experienced developer, this guide will help you confidently prepare for your next interview.
React Redux Interview Questions and Answers
Preparing for the React Redux interview? These 60 questions and answers will help you understand key concepts like state management, actions, reducers, middleware, and best practices. They will also enhance your confidence in explaining Redux in real-world applications.
Top 20 React Redux Interview Questions and Answers for Freshers
For freshers preparing for a React Redux interview, these top 20 questions will help you grasp essential concepts like state management, actions, reducers, and the Redux workflow. They will also boost your confidence in explaining Redux fundamentals effectively.
Q1. What is Redux?
Ans: Redux is a state management library used inapplications in JavaScript. It allows you to manage the application's state in a centralized store, making it accessible to all components. Instead of manually passing data through props from one component to another, Redux ensures that all components can access the shared state. This centralized state management helps in building large-scale applications efficiently and maintainably, especially when dealing with complex data flow.
Q2. Why do you need Redux in a React application?
Ans: You needReduxwhen your application has multiple components that need to be shared or managed in a global state.Without Redux, passing data between components can get cumbersome, especially as your application grows. Redux simplifies this by allowing a single, central store to hold the state, which any component can access and update. This makes managing the state more predictable and the flow of data more transparent.
Q3. What are the core principles of Redux?
Ans: The core principles of Redux are:
- Single source of truth: The state of the application is stored in one global object, known as the Redux store. This ensures that you have a consistent view of the entire application state, making debugging and state management easier.
- State is read-only: You cannot directly change the state. Instead, you dispatch actions to express the intention to change the state, ensuring that the state remains immutable and predictable.
- Changes are made with pure functions: The logic for updating the state is encapsulated in reducers, which are pure functions. These functions receive the current state and an action and return a new state without mutating the previous one.
Q4. What is an action in Redux?
Ans: An action is a plain JavaScript object that describes what happened or what should happen in the application. It is the only way to send information to the Redux store. Actions typically have a type property, which is a string that identifies the action, and an optional payload, which contains any additional data the action needs to carry.
const incrementAction = {
type: 'INCREMENT'
};
Q5. What is a reducer in Redux?
Ans: A reducer is a pure function that specifies how the state of the application should change in response to an action. It takes the current state and the action as arguments and returns a new state. The state is not mutated directly; instead, a new copy of the state is created and returned.
function counterReducer(state = 0, action) {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
}
Q6. What is the Redux store?
Ans: The store is a central repository where all of your application's state lives. It is created using a reducer, and it holds the state of the entire application. The store allows you to dispatch actions, subscribe to state changes, and get the current state of the application. In essence, it acts as a centralized source of truth for your app's state.
Q7. How do you create a Redux store?
Ans: You create a Redux store by calling the createStore method and passing your root reducer to it. The store can be configured with React Middleware, and it is typically wrapped in a Provider component to make it available throughout your React component tree.
import { createStore } from 'redux';
const store = createStore(counterReducer);
Q8. How do you connect React and Redux?
Ans: To connect React and Redux, you use the react-redux library. The Provider component is used to pass the Redux store to your React component tree, allowing all components to access the store. The connect function is used to connect specific components to the store and allows those components to receive state and dispatch actions.
import { Provider } from 'react-redux';
Q9. What is middleware in Redux?
Ans: Middleware in Redux provides a third-party extension point between dispatching an action and the moment it reaches the reducer. It allows you to extend Redux's capabilities, such as logging actions, handling asynchronous operations (e.g., API calls), and modifying actions before they reach the reducer. Common middleware includes redux-thunk and redux-saga.
Q10. What is the use of the combineReducers
function?
Ans: The combineReducers function is used to combine multiple reducers into one. It allows you to manage different slices of the state in separate reducer functions. This is useful when you have a large application and want to break the state management into smaller, more manageable pieces.
import { combineReducers } from 'redux';
const rootReducer = combineReducers({
counter: counterReducer,
user: userReducer,
});
Q11. How do you dispatch an action?
Ans: You dispatch an action by calling the dispatch method on the Redux store. The dispatch method takes an action object as an argument and sends it to the reducer for state updates.
store.dispatch({ type: 'INCREMENT' });
Q12. What is the difference between Redux and Context API?
Ans: The Redux library is a more powerful and feature-rich tool for managing complex states in large applications. It provides a central store, middleware, tools, and a more structured way to manage the state. On the other hand, React's Context API is a simpler and more lightweight tool primarily designed to share data across components without passing Props in React. Redux is more suitable for large-scale applications where the state needs to be shared across multiple components.
Q13. How do you handle asynchronous actions in Redux?
Ans: You handle asynchronous actions using middleware like redux-thunk or redux-saga. These middlewares allow you to dispatch functions instead of plain action objects. The function can contain asynchronous code like API requests, and once the data is received, it can dispatch another action to update the state.
Q14. What is Redux Thunk?
Ans: Redux Thunk is a middleware that allows action creators to return functions instead of plain action objects. These functions can contain asynchronous code and dispatch multiple actions, making them suitable for handling complex, async-related actions like fetching data from APIs.
const fetchUser = () => {
return (dispatch) => {
fetch('/user')
.then(response => response.json())
.then(data => dispatch({ type: 'SET_USER', payload: data }));
};
};
Q15. What are selectors in Redux?
Ans: Selectors are functions that retrieve specific parts of the state from the Redux store. They can be used to improve performance by memorizing the result of the state extraction and preventing unnecessary re-renders of components. A selector is a function that takes the state and returns the data that a component needs.
Q16. What is the role of the Provider component in Redux?
Ans: The Provider component is used to provide the Redux store to your entire React application. It allows all components within the application to access the Redux store and interact with it, either by reading state or dispatching actions. The Provider is typically used at the top level of your component tree to ensure that the store is accessible to all components.
Q17. What is the purpose of the connect function in Redux?
Ans: The connect function is used to connect React components to the Redux store. It allows components to access the store's state and dispatch actions. By using mapStateToProps and mapDispatchToProps, you can specify which parts of the state a component should subscribe to and which actions it can dispatch. This helps in optimizing performance by reducing unnecessary renders and enabling efficient state management.
Q18. What is the use of mapStateToProps and mapDispatchToProps in Redux?
Ans: The mapStateToProps function is used to map the Redux store state to the props of a React component. It allows the component to subscribe to specific parts of the state that it needs. The mapDispatchToProps function is used to map action creators to the component's props so that the component can dispatch actions. These functions are commonly used in the connect function to link React components to the Redux store.
Q19. What is the difference between the React Context API and Redux?
Ans: The React Context API is a simpler tool built into React for passing data through the component tree without manually passing props down at every level. It is best suited for small-scale applications or sharing global data like themes or user authentication. On the other hand, Redux is a more advanced state management library that provides a structured approach to handling complex states in large applications. Redux includes a global store, actions, reducers, and middleware, making it more suitable for managing the state of large-scale applications with complex logic.
Q20. How does Redux handle immutability?
Ans: Redux enforces immutability by making sure that the state is never mutated directly. Instead of modifying the state directly, the state is replaced by a new object in the reducer. This is achieved by using functions that return a new state object rather than modifying the existing one. This immutability ensures that the application state remains predictable and helps with debugging and time-travel debugging using Redux DevTools.
Top 15 React Redux Interview Questions and Answers for Intermediates
For those at an intermediate level, these top 15 React Redux interview questions will help you deepen your understanding of concepts like middleware, asynchronous actions, performance optimization, and best practices in state management. They will also enhance your ability to explain Redux in real-world applications.
Q21. What is the difference between Redux and React's Context API?
Ans: The Redux library is a state management solution for JavaScript applications that provides a predictable state container. It is designed for managing complex state across large applications with many components. On the other hand, React's Context API is a lighter, simpler tool for passing data between components without the need for props. While Redux is best suited for large-scale applications requiring actions, middleware, and more structured management, the Context API is better for smaller apps or situations where you don't need complex state management.
Q22. Explain the concept of middleware in Redux. Can you name some commonly used middleware?
Ans: Middleware in Redux is a way to extend Redux’s capabilities. It acts as a middle layer between dispatching an action and reaching the reducer, allowing you to intercept or modify actions before they reach the reducer. This is particularly useful for handling asynchronous actions, logging, or performing side effects. Some commonly used middlewares are:
- Redux Thunk: Allows you to write action creators that return functions instead of action objects, enabling asynchronous operations like API calls.
- Redux Saga: A more advanced middleware for handling side effects, often used for managing complex asynchronous flows like retries, cancellations, etc.
Q23. What are the advantages of using Redux in a React application?
Ans: Some key advantages of using Redux in a React application include:
- Predictable state: Redux ensures that your application state is predictable, as it’s stored in a single object that can only be modified by dispatching actions.
- Centralized state management: All state is stored in one place, making it easier to manage, debug, and update state across your application.
- Middleware support: Redux allows middleware for handling side effects, making it easy to deal with asynchronous operations.
- DevTools integration: Redux works seamlessly with Redux DevTools for inspecting actions, states, and even time travel debugging.
Q24. Can you explain how Redux's store works?
Ans: The store in Redux holds the entire state of your application. It's the central place where all application data resides. The store is created by calling createStore()
and can be accessed by components via the useSelector
hook (in functional components) or connect
(in class components). The store provides three key functions:
- getState(): Allows access to the current state.
- dispatch(): Dispatches actions that trigger state changes.
- subscribe(): Registers a callback to listen for state updates.
Q25. What is the purpose of action creators in Redux?
Ans: Action creators are functions that return action objects. They help ensure actions are created consistently across your application. Instead of directly creating action objects, which can lead to errors, you use action creators to encapsulate this process. Action creators can also be used to handle more complex logic, such as asynchronous operations. An action creator might look like this:
function fetchUserData() {
return async (dispatch) => {
const response = await fetch('/api/user');
const data = await response.json();
dispatch({ type: 'USER_FETCH_SUCCESS', payload: data });
};
}
Q26. What is the purpose of the combineReducers function in Redux?
Ans: The combineReducers function is used to combine multiple reducers into a single reducer function. It is necessary when your application has multiple slices of state, and each slice has its own reducer. It helps keep your reducer logic modular and organized. Here's an example:
import { combineReducers } from 'redux';
const rootReducer = combineReducers({
user: userReducer,
posts: postsReducer,
comments: commentsReducer
});
Q27. What are "reducer" functions in Redux?
Ans: A reducer in Redux is a pure function that takes the current state and an action as arguments and returns a new state. It determines how the state should change in response to an action. Reducers do not modify the current state directly but return a new state object. This ensures that state changes are predictable and traceable. Here’s an example of a simple reducer:
function userReducer(state = {}, action) {
switch (action.type) {
case 'SET_USER':
return { ...state, user: action.payload };
default:
return state;
}
}
Q28. How would you optimize performance in Redux-based applications?
Ans: Optimizing performance in Redux can involve several strategies:
- Memoization: Use Reselect to memoize selectors, preventing unnecessary re-renders of components.
- Lazy loading: Lazy loadingin React Load reducers only when they are needed to avoid unnecessary state updates in parts of the app that are not currently in use.
- Batching updates: Avoid multiple dispatches in quick succession by batching updates to prevent unnecessary re-renders.
- Immutable data structures: Use immutable data structures to prevent accidental mutations, which can lead to unnecessary re-renders.
Q29. Can you explain the concept of "selector" in Redux?
Ans: A selector is a function that extracts and derives data from the Redux store. Selectors can be used to compute derived data, combine multiple pieces of state, or filter data before passing it to a component. Selectors help make the code more readable and maintainable. You can use libraries like Reselect to create efficient, memoized selectors. Here's an example:
import { createSelector } from 'reselect';
const selectUserData = (state) => state.user;
const selectUserName = createSelector([selectUserData], (user) => user.name);
Q30. What is the purpose of Redux DevTools?
Ans: Redux DevTools is a set of developer tools that allow you to inspect and debug the Redux store. It provides features like time-travel debugging, inspecting actions and states, and even dispatching actions manually. It’s extremely useful for understanding how the state changes over time and troubleshooting issues in complex Redux applications. The tools can be integrated into your application by adding the Redux DevTools extension or configuring it programmatically.
Q31. What is the concept of "action types" in Redux?
Ans: In Redux, action types are constants that define the type of action being dispatched. These constants help identify what kind of state change an action is requesting. It’s a best practice to define action types as constants to avoid typos in action creators or reducers. For example:
export const ADD_ITEM = 'ADD_ITEM';
export const REMOVE_ITEM = 'REMOVE_ITEM';
Q32. How do you handle async operations in Redux?
Ans: Async operations are handled in Redux using middleware such as Redux Thunk or Redux Saga. These middlewares allow you to dispatch functions (in the case of Redux Thunk) or manage complex asynchronous flows (in the case of Redux Saga). With Redux Thunk, an action creator can return a function instead of an action, which can then dispatch actions based on the outcome of the asynchronous operation.
Q33. What is a "payload" in a Redux action?
Ans: The payload in a Redux action refers to the data that is passed along with the action to update the store. The payload typically contains the necessary information to modify the state. For example:
const action = {
type: 'ADD_ITEM',
payload: { id: 1, name: 'Item 1' }
};
Q34. What are "actions" in Redux?
Ans: In Redux, actions are plain JavaScript objects that represent an event or a change that you want to happen in the application’s state. An action must have a type property that describes the action being performed. It may also have additional data, typically in a payload property, which provides the information necessary for updating the state. Actions are dispatched to trigger state changes in the Redux store.
Q35. How would you handle large-scale state management in a Redux-based application?
Ans: To handle large-scale React State management in a Redux-based application, you should focus on structuring your state, actions, and reducers efficiently. Some strategies to manage large-scale state include:
- Modularize reducers: Use combineReducers to break down the state into smaller, manageable parts. This way, each reducer only handles a specific slice of the state.
- Normalize state: Flatten nested objects or arrays within the state. Tools like normalizr can help keep your data normalized, making it easier to manage.
- Use selectors: Utilize selectors (using libraries like Reselect) to avoid repetitive logic and optimize performance by memoizing complex derived state.
- Lazy load reducers: For applications with large amounts of state, consider using code splitting or lazy loading of reducers only when necessary, preventing unnecessary large chunks of state from being loaded all at once.
- Implement pagination or infinite scroll: For large datasets, implement pagination or infinite scroll to reduce the amount of data loaded at once and to manage state more efficiently.
Top 25 React Redux Interview Questions and Answers for experienced
For experienced professionals, these top 25 React Redux interview questions will help you master advanced concepts like middleware, async state management with Redux Thunk or Saga, performance optimization, and best practices. They will also enhance your ability to build scalable and efficient Redux applications.
Q36. How do you structure a large-scale Redux application?
A large-scale Redux application should follow a modular structure to maintain scalability and maintainability. A common approach is to structure it by feature:
- Store: Contains the main Redux store configuration.
- Features: Each feature has its own slice with actions, reducers, and selectors.
- Components: UI components connected to Redux.
- Hooks: Custom hooks for encapsulating Redux logic.
Example folder structure:
/src
├── store/
│ ├── index.js
│ ├── rootReducer.js
│ ├── middleware.js
├── features/
│ ├── auth/
│ │ ├── authSlice.js
│ │ ├── authActions.js
│ │ ├── authSelectors.js
│ ├── user/
│ │ ├── userSlice.js
│ │ ├── userActions.js
│ │ ├── userSelectors.js
Q37. What are the best practices for managing complex state in Redux?
To manage complex state efficiently:
- Normalize State: Use a flat structure instead of deeply nested objects.
- Use Slices: Divide state into feature-specific slices using Redux Toolkit.
- Use Selectors: Keep logic separate from components with Reselect.
- Async Handling: Use Redux Thunk or Redux Saga for side effects.
- Persist Data: Use Redux Persist to retain state between reloads.
Q38. How does Redux persist state between page reloads?
Redux state is lost on a page reload unless persisted using Redux Persist. It stores the Redux state in localStorage or sessionStorage.
import { persistStore, persistReducer } from 'redux-persist';
import storage from 'redux-persist/lib/storage';
import { createStore } from 'redux';
const persistConfig = {
key: 'root',
storage,
};
const persistedReducer = persistReducer(persistConfig, rootReducer);
const store = createStore(persistedReducer);
const persistor = persistStore(store);
Q39. What is the difference between Redux Saga and Redux Thunk?
Redux Thunk and Redux Saga are middleware for handling asynchronous actions, but they work differently:
- Redux Thunk: Uses functions to dispatch async actions, working well for simple cases.
- Redux Saga: Uses generator functions for better control over async flows, suitable for complex side effects.
Q40. How do you handle race conditions in Redux?
Race conditions occur when multiple async operations compete for the same state. To handle them:
- Cancellation Tokens: Use AbortController for API requests.
- Redux Saga Effects: Use takeLatest to cancel previous requests.
Q41. What are some common performance pitfalls in Redux, and how do you avoid them?
Common pitfalls include:
- Unnecessary Renders: Avoid excessive re-renders by using memoization with Reselect.
- Large State Trees: Normalize state to prevent deep object updates.
- Too Many Actions: Batch actions to reduce state updates.
Q42. How do you implement optimistic updates in Redux?
Optimistic updates assume success before the server confirms. Steps:
- Update the UI immediately.
- Send the API request.
- Revert changes if the request fails.
Q43. What is the role of reselect in Redux applications?
Reselect improves performance by memoizing derived state, preventing unnecessary re-computation.
import { createSelector } from 'reselect';
const selectUsers = (state) => state.users;
const selectActiveUsers = createSelector([selectUsers], (users) =>
users.filter((user) => user.active)
);
Q44. How do you debug a Redux application effectively?
Use Redux DevTools to inspect state changes, actions, and time travel debugging.
Q45. What are normalized state shapes, and why are they important in Redux?
Normalization flattens deeply nested objects to improve performance and maintainability.
Q46. How do you handle server-side rendering (SSR) with Redux?
Server-side rendering (SSR) with Redux involves initializing the Redux store on the server before sending the HTML to the client. In frameworks like Next.js, you can use getServerSideProps or getInitialProps to fetch data and pre-populate the Redux store. The preloaded state is then sent to the client, where Redux rehydrates it, ensuring the application starts with the correct state.
Q47. What is the difference between Redux Toolkit and traditional Redux?
Redux Toolkit simplifies Redux development by reducing boilerplate code and providing built-in support for reducers, actions, and middleware. Traditional Redux requires manually defining action creators, reducers, and middleware, which can be repetitive. Redux Toolkit introduces createSlice for managing reducers efficiently, configureStore for setting up the store with middleware, and includes Immer for immutable state updates.
Q48. How do you implement authentication and authorization using Redux?
In Redux, authentication and authorization are managed by storing user authentication tokens and roles in the Redux store. Middleware is used to intercept actions and restrict access based on authentication status. When a user logs in, the token is stored in Redux and optionally persisted using Redux Persist. Protected routes ensure that unauthorized users are redirected to login pages.
Q49. How can you manage multiple reducers efficiently in Redux?
To manage multiple reducers efficiently, use the combineReducers function, which allows splitting Redux state into separate, independent modules. Each reducer handles a specific slice of the state, such as authentication, user data, or UI state. This improves code maintainability and scalability in large applications.
Q50. How do you handle WebSockets and real-time updates in Redux?
To handle WebSockets in Redux, use middleware that listens for WebSocket events and dispatches actions based on the received data. The middleware establishes a WebSocket connection, processes incoming messages, and updates the Redux store in real time. This ensures the UI stays synchronized with the backend data.
Q51. What is Redux Persist, and how does it work?
Redux Persist is a library that enables persistence of the Redux state across page reloads by storing data in local storage or session storage. It wraps the Redux store and automatically saves and rehydrates state when the application loads, preventing data loss on refresh.
Q52. How do you manage form state using Redux?
Managing form state in Redux can be done by storing input values in the Redux store and updating them through actions. Libraries like Redux Form and Formik simplify form handling by managing field updates, validation, and submission logic. Keeping form state in Redux allows centralized state tracking for complex forms used across multiple components.
Q53. How do you optimize a Redux application for mobile devices?
To optimize a Redux application for mobile devices, minimize state size, reduce unnecessary re-renders, and batch multiple state updates. Use Reselect for memoized selectors, avoid deeply nested state structures, and implement lazy loading for large datasets. Optimizing network requests by caching responses can also enhance performance.
Q54. What are the trade-offs of using Redux vs. React Context for state management?
Redux is more suitable for complex global state management, offering structured data flow, debugging tools, and middleware support. However, it introduces additional setup and complexity. React Context is a built-in, lightweight alternative for managing state in small applications but can lead to performance issues due to excessive re-renders in deeply nested components.
Q55. How do you handle API request failures gracefully in Redux?
Handling API request failures in Redux involves dispatching error actions and implementing retry strategies. Middleware like Redux Thunk or Redux Saga can catch errors and trigger fallback actions. Displaying error messages and using exponential backoff for retries ensures a better user experience, preventing unnecessary disruptions caused by network failures.
Q56. What is entity normalization in Redux, and how do you implement it?
Entity normalization in Redux structures state efficiently by storing entities in a flat format. Instead of keeping nested objects, data is stored using an entities object where each entity type is a key, and its values are stored by unique IDs. This improves state management, performance, and lookup efficiency.
To implement entity normalization:
- Use libraries like normalizr to structure the data automatically.
- Store entities in a dictionary format (e.g.,
state.users.byId[userId]
). - Maintain an ids array to track entity order.
- Use selectors to access denormalized data.
import { normalize, schema } from 'normalizr';
const user = new schema.Entity('users');
const article = new schema.Entity('articles', { author: user });
const normalizedData = normalize(apiResponse, article);
console.log(normalizedData);
Q57. How do you handle module splitting in Redux for large-scale applications?
Handling module splitting in Redux ensures better code organization and performance. Instead of loading all reducers at once, dynamically load parts of the Redux store when needed.
Best practices:
- Use React.lazy and loadable-components for code-splitting.
- Inject reducers dynamically using
store.replaceReducer
. - Organize Redux modules by feature (e.g., authentication, cart).
const asyncReducer = (state = {}, action) => {
switch (action.type) {
case 'DYNAMIC_ACTION':
return { ...state, data: action.payload };
default:
return state;
}
};
store.replaceReducer(combineReducers({ async: asyncReducer }));
Q58. How do you test Redux reducers, actions, and selectors?
Testing Redux ensures state management functions correctly. Use Jest and React Testing Library for testing.
- Reducers: Validate state transitions.
- Actions: Ensure correct action objects.
- Selectors: Verify data retrieval.
import reducer from '../reducers';
import { someAction } from '../actions';
test('should return the initial state', () => {
expect(reducer(undefined, {})).toEqual(initialState);
});
test('should handle SOME_ACTION', () => {
expect(reducer(initialState, someAction())).toEqual(expectedState);
});
Q59. How do you handle undo and redo functionality in Redux applications?
Implementing undo and redo in Redux requires tracking previous states and future states.
- Store an array of past states and future states.
- Push current state to past states on each action.
- Provide UNDO and REDO actions.
import undoable from 'redux-undo';
const rootReducer = combineReducers({
counter: undoable(counterReducer)
});
store.dispatch({ type: 'UNDO' });
store.dispatch({ type: 'REDO' });
Q60. How do you migrate a legacy React application to use Redux efficiently?
Migrating a legacy React application to Redux requires a step-by-step approach.
- Identify global state: Determine shared state.
- Introduce Redux gradually: Start with a single feature.
- Use Redux Toolkit: Simplify state management.
- Refactor components: Replace prop-drilling with Redux state.
import { configureStore, createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: 0,
reducers: {
increment: (state) => state + 1,
},
});
const store = configureStore({
reducer: { counter: counterSlice.reducer },
});
Read More: React Interview Questions & Answers |
Summary
The React Redux Interview Questions article covers 60+ essential questions to help you master Redux concepts like state management, actions, reducers, middleware, and best practices. It provides clear explanations and real-world insights to boost your confidence in interviews. Whether you're a beginner or an experienced developer, these questions will help you strengthen your understanding of Redux.Looking to level up your React skills? Check out the ReactJS Certification Training and become a certified expert!
React Redux Interview Questions - Test Your Knowledge!
Q 1: What is Redux in React?
- (a) A React library for animations
- (b) A state management library
- (c) A database for React applications
- (d) A CSS framework
Q 2: What are the core principles of Redux?
- (a) Single source of truth
- (b) State is read-only
- (c) Changes are made with pure functions
- (d) All of the above
Q 3: What is an action in Redux?
- (a) A function that updates the state
- (b) An object that describes what should change
- (c) A built-in method of Redux
- (d) A component in React
Q 4: What is a reducer in Redux?
- (a) A function that modifies state based on actions
- (b) A built-in React Hook
- (c) A database query
- (d) A Redux middleware
Q 5: What is middleware in Redux?
- (a) A function that intercepts actions
- (b) A built-in React function
- (c) A component that connects Redux with React
- (d) A Redux reducer
Download This PDF - React Redux Interview Questions PDF By ScholarHat |
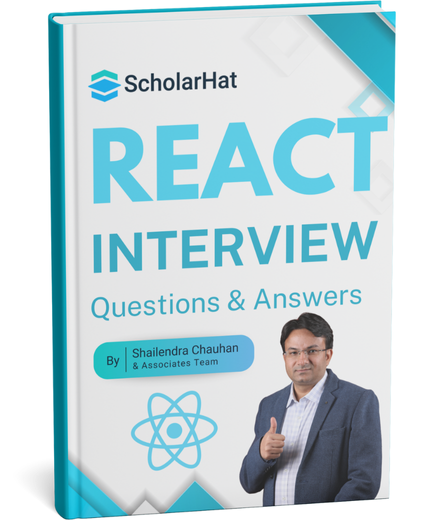
Crack Your Next React Interview – Grab the Free Expert eBook!
React Interview Questions and Answers Book Unlock expert-level React interview preparation with our exclusive eBook! Get instant access to a curated collection of real-world interview questions, detailed answers, and professional insights — all designed to help you succeed.
No downloads needed — just quick, free access to the ultimate guide for React interviews. Start your preparation today and move one step closer to your dream job!.
FAQs
Take our React skill challenge to evaluate yourself!
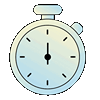
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.