09
NovReact-Router-Dom-npm - Everything You Need to Know
What is react-router-dom?
You must be aware of how react-router-dom works if you have used React several times, but for first-timers, remember their names. The react-router-dom library is important for React developers working on React Applications. It helps them with dynamic routing within web apps.
This React Tutorial will give a gist of the meaning of react-router-dom, react-router-dom example, and much more. To explore various exciting topics in React, enroll in our React Certification Training right now!
Read More: Top 50 React Interview Question & Answers |
Building Single-Page Applications with React Router DOM
Single-page apps are a typical case for web development projects nowadays. This is because these are web applications meant to be in the user’s browser. Therefore, there is no need to reload them each time users click on links or do something else to make them more user-friendly. One of the most favored tools for managing routes within SPAs is react-router-dom. This library helps developers create navigable and dynamic user interfaces with ease. Let's explore its core concepts and see how to use it effectively.
Core Concepts of React Router DOM
In order to fully grasp the code, you need to know the fundamental aspects of react-router-dom:
- Routes—These are used specifically to determine the path that your app will use and choose which component to present according to a given URL.
- Navigation- React Navigation allows users to move between different parts of the application without refreshing the page.
- Components- react-router-dom provides several components that help set up and manage routing in a React application.
Using <BrowserRouter> and <Routes> to Set Up Routing
The first step in using react-router-dom is to set up the routing context with <BrowserRouter>. This component wraps your entire application and enables the use of the routing components.
import { BrowserRouter, Routes, Route } from 'react-router-dom';
function App() {
return (
<BrowserRouter>
<Routes>
{/* Define your routes here */}
</Routes>
</BrowserRouter>
);
}
export default App;
Defining Routes with the <Route> Component
import Home from './Home';
import About from './About';
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
</Routes>
</BrowserRouter>
);
}
Read More: |
Creating Navigation Links with <Link>
import { Link } from 'react-router-dom';
function Navbar() {
return (
<nav>
<Link to="/">Home</Link>
<Link to="/about">About</Link>
</nav>
);
}
Handling Dynamic URLs with Route Parameters
import { useParams } from 'react-router-dom';
function UserProfile() {
const { userId } = useParams();
return <div>User Profile: {userId}</div>;
}
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/user/:userId" element={<UserProfile />} />
</Routes>
</BrowserRouter>
);
}
Nested Routing for Complex UIs in React Router DOM
function Dashboard() {
return (
<div>
<h2>Dashboard</h2>
<Routes>
<Route path="stats" element={<Stats />} />
<Route path="settings" element={<Settings />} />
</Routes>
</div>
);
}
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/dashboard/*" element={<Dashboard />} />
</Routes>
</BrowserRouter>
);
}
Read More: React Developer Salary in India 2024 (For Freshers & Experienced) |
Additional Features
1. Redirects
import { Navigate } from 'react-router-dom';
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/old-path" element={<Navigate to="/new-path" />} />
<Route path="/new-path" element={<NewComponent />} />
</Routes>
</BrowserRouter>
);
}
2. Route Guards
3. Custom Route Handling with use routes
4. Programmatic Navigation with us navigate
5. Lazy Loading with React.lazy
Conclusion
FAQs
Q1. What is react-router-dom and what does it do?
Q2. How do I set up basic routing with react-router-dom?
Q3. What is the difference between <BrowserRouter> and <Routes>?
Q4. What happened to withRouter in v6?
Take our React skill challenge to evaluate yourself!
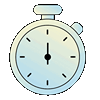
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.