21
NovTop 50 Spring Boot Interview Questions and Answer
Spring Boot Interview Question With Answer
Spring Boot is an open-source framework for building standalone, production-ready Spring applications. It provides a quick approach to creating microservices and web applications using the Spring Framework, avoiding the need for significant standard code.
In this Java Tutorial, I'll cover the top 50 Spring Boot interview questions and answers, separated into three categories: 20 for beginners, 15 for intermediate developers, and 15 for experienced experts. This article will help you fully prepare for any Spring Boot interviews you may have.
Top 20 Spring Boot Interview Questions and Answers For Beginners
1. What is Spring Boot?
Spring Boot is developed on top of the Spring framework to create stand-alone RESTful web applications with minimal configuration. The application does not require external servers to function because it has embedded servers such as Tomcat and Jetty.
- The Spring Boot framework operates independently.
- It creates production-ready spring apps.
2. What are the Features of Spring Boot?
Spring Boot includes several important features. A few of them are listed below:
- Auto-configuration: Spring Boot automatically configures dependencies using the @EnableAutoconfiguration annotation, reducing boilerplate code.
- Spring Boot Starter POM: These POMs provide pre-configured dependencies for functions such as database, security, and Maven setup.
- Spring Boot CLI (Command Line Interface): This command line tool is mostly used for managing dependencies, creating projects, and running apps.
- Actuator: The Spring Boot Actuator performs health checks, collects metrics, and monitors application endpoints. It also facilitates troubleshooting management.
- Embedded Servers: Spring Boot has embedded servers such as Tomcat and Jetty that allow applications to execute quickly. There is no need for external servers.
Q3. Explain the concept of Spring Boot Starters.
Spring Boot Starters are pre-configured sets of dependencies that make it simple to include the necessary libraries for various functionalities, including web development, security, and JPA.
Example:
<dependency>
org.springframework.boot
spring-boot-starter-web
</dependency>
Q4. What is the purpose of the @SpringBootApplication annotation?
@SpringBootApplication represents a shortcut for three annotations: @Configuration, @EnableAutoConfiguration, and @ComponentScan. It initializes the application context and enables auto-configuration.
Q5. What are the advantages of using Spring Boot?
Spring Boot is a framework for building standalone, production-grade Spring-based applications, offering many advantages.
- Easy to use: Spring Boot reduces the amount of boilerplate code required to construct a Spring application.
- Rapid Development: Spring Boot's opinionated approach and auto-configuration allow developers to create apps quickly without the need for time-consuming setup, reducing development time.
- Scalable: Spring Boot applications are designed to be scalable. This means that they may be easily scaled up or down to meet your application's requirements.
- Production-ready: Spring Boot contains capabilities such as metrics, health checks, and externalized configuration, all of which are intended for usage in production situations.
Q6. Define the Key Components of Spring Boot.
The key components of Spring Boot are given below:
- Spring Boot Starters
- Auto-configuration
- Spring Boot Actuator
- Spring Boot CLI for Embedded Servers
Q7. How does Spring Boot handle dependencies?
Spring Boot manages dependencies with the Maven or Gradle build tools. It includes a set of default dependencies that may be modified, allowing developers to select the most relevant versions and libraries for their applications.
Feature | Default Dependency |
Web Development | spring-boot-starter-web |
Data Access | spring-boot-starter-data-jpa |
Security | spring-boot-starter-security |
Q8 Why do we prefer Spring Boot over Spring?
Aspect | Spring Boot | Spring Framework |
Setup and Configuration | Spring Boot automatically configures your project, making setup simpler and faster. | Manual configuration is required, which might take a long and be complex. |
Development Speed | Allows faster development with less boilerplate code. | Development is slower because more setup is needed. |
Dependency Management | Manages dependencies automatically, simplifying project setup. | Dependencies need to be managed manually, which can be complicated. |
Embedded Server | It includes an embedded server that allows you to run applications directly. | Requires an external server setup to deploy applications. |
Microservices | Designed for microservices, making them easy to create and deploy. | It can be used for microservices but requires more configuration. |
Production-Ready Features | It includes features like metrics and health checks that are out of the box. | Does not include these features by default; they need to be added manually. |
Community& Ecosystem | Has a large community with frequent updates and extensive resources. | Also has a strong community, but it evolves more slowly compared to Spring Boot. |
Q9 What is Spring Initializr?
Q10 How do you create a Spring Boot project using Spring Initializer?
- Step 1: Open Spring Initializr by going to https://start.spring.io.
- Step 2:Specify Project Details: To produce and download a Spring Boot project, input all the required information and click the Produce Project button. Then, open the downloaded zip file and drag it into your favorite integrated development environment.
- Step 3: After that, import the file into Eclipse.
Q11 What are the Spring Boot Starter Dependencies?
- Data JPA starter
- Web starter
- Security starter
- Test Starterz
- Thymeleaf starter
Q12 How does a spring application get started?
- A Spring application is launched by invoking the main() method of the SpringApplication class, which has the @SpringBootApplication annotation.
- This function accepts a SpringApplicationBuilder object as an argument and is used to configure the application.
- Once the SpringApplication object is constructed, the run() function is invoked.
- After initializing the application context, the run() method launches the program's embedded web server.
Example
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MySpringApplication {
public static void main(String[] args) {
SpringApplication.run(MySpringApplication.class, args);
}
}
Q13 Explain the concept of auto-configuration in Spring Boot.
- The application context is automatically configured via auto-configuration using the dependencies found in the classpath.
- As a result, less manual configuration is required, which facilitates getting started.
Q14 How do you disable a specific auto-configuration class?
To disable a certain auto-configuration class in a Spring Boot application, use the @EnableAutoConfiguration annotation and the "exclude" attribute.
Example
spring.autoconfigure.exclude=org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration
Q14 What is the starter dependency of the Spring boot module?
- Dependencies
- Version Control
- Certain features require configuration to function properly.
Example
<dependency>
org.springframework.boot
spring-boot-starter-web
</dependency>
Q15 How do you create a Spring Boot project using boot CLI?
- Setting up the CLI: Use SDKMAN to install the Spring Boot CLI (Command-Line Interface) directly!
- Employing the CLI: Once installed, run the CLI by typing spring and pressing Enter.
- Launch a New Project: Using start.spring.io and the init command, you can launch a new project without leaving the shell.
- Using the Embedded Shell: Spring Boot includes command-line completion techniques for the BASH and ZSH shells.
Q16 How does Spring Boot handle security?
- Spring Boot interfaces with Spring Security to handle authentication and authorization.
- It has default setups that can be changed with annotations and security configuration classes.
Example
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().permitAll();
}
}
Q17 How do you create a custom banner in Spring Boot?
- To build a custom startup banner, place a banner.txt file in the src/main/resources directory.
- Inside banner.txt, you can add any text, ASCII art, or messages you want to display when the application starts.
- Run the application, and your custom Spring Boot banner will appear in the console.Q18 What is the use of @ConfigurationProperties in Spring Boot?
@ConfigurationProperties maps properties from application.properties or application.yml to Java objects. This provides organized access to your application's configuration properties.
Example
@ConfigurationProperties(prefix = "app")
public class AppConfig {
private String name;
private String description;
// getters and setters
}
Q19 How does Spring Boot handle external configuration?
- Spring Boot supports a variety of external configuration options.
- It supports property files (application.properties or application.yml) that can be stored in a variety of locations, including the classpath, file system, and external directories.
- Spring Boot also allows environment variables, command-line arguments, and the creation of profiles for various deployment contexts.
- The configuration values can be obtained using the @Value annotation or bound to Java objects using the @ConfigurationProperties annotation.
Q20 How do you handle exceptions in Spring Boot?
- The @ControllerAdvice and @ExceptionHandler annotations in Spring Boot allow exceptions to be handled globally.
- This enables the logic for addressing errors throughout the program to be centralized.
Example
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity handleException(Exception e) {
return new ResponseEntity<>(e.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Top 15 Spring Boot Interview Questions and Answers For Intermediates
Q21 What is Tomcat's default port in spring boot?
Q22 Can we disable the default web server in the Spring Boot application?
Q23 Explain the flow of HTTPS requests through the Spring Boot application.
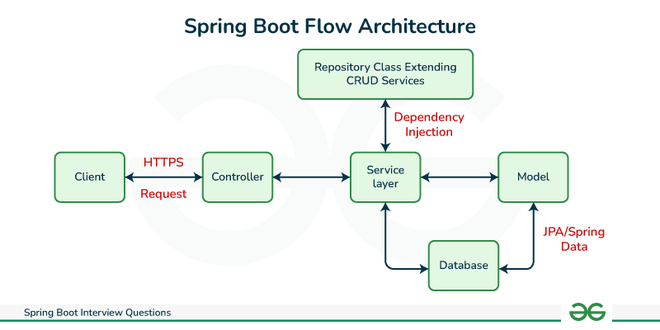
- The client initiates an HTTP request (GET, POST, PUT, DELETE) to the browser.
- Following that, the request is routed to the controller, where it is mapped and handled along with all other requests.
- Following this, all business logic will be executed in the Service layer. It performs business logic on data that has been mapped to JPA (Java Persistence API) via model classes.
- The repository layer handles all CRUD activities for the REST APIs.
- If there are no errors, the end users receive a JSP page.
Q24 Explain @RestController annotation in Spring Boot.
The @RestController annotation is essentially a shortcut for creating RESTful services. It combines two annotations.
- @Controller: Indicates that the class is a request handler in the Spring MVC framework.
- @ResponseBody: This tells Spring to convert method return values (objects, data) directly into HTTP responses rather than rendering views.
It allows us to define endpoints for multiple HTTP methods (GET, POST, PUT, and DELETE), return data in various forms (e.g., JSON, XML), and map request parameters to method arguments.
Q25 How do you implement RESTful web services in Spring Boot?
Spring Boot allows you to construct RESTful web services using the @RestController and @RequestMapping annotations. The @RestController annotation automatically serializes return objects to JSON or XML, making it simple to build REST APIs.
Example
@RestController
@RequestMapping("/api")
public class MyRestController {
@GetMapping("/items")
public List getItems() {
return itemService.getAllItems();
}
}
Q26 What is the difference between @RequestMapping and @GetMapping in Spring Boot?
Annotation | Purpose |
@RequestMapping | Annotation that can be used for handling HTTP requests of all methods, including GET and POST. |
@GetMapping | A specialized version of @RequestMapping is used to handle GET requests. |
Q27 How do you integrate Spring Boot with a database?
Example
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
Example application.properties:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=password
Q28 What is the purpose of @Entity in Spring Boot?
Example
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// getters and setters
}
Q29 How do you implement pagination in Spring Boot?
Example
@Repository
public interface UserRepository extends JpaRepository {
Page findAll(Pageable pageable);
}
Example usage:
Pageable pageable = PageRequest.of(0, 10);
Page users = userRepository.findAll(pageable);
Q30 What is the purpose of @Transactional in Spring Boot?
Example
@Service
public class UserService {
@Transactional
public void createUser(User user) {
userRepository.save(user);
}
}
Q31 Difference between @Controller and @RestController.
Features | It is used to mark a class as a controller. | It is a combination of @Controller and @ResponseBody. |
Application | It is used for web applications. | It is used for RESTful APIs. |
Request handling & mapping. | It is used with @RequestMapping to map HTTP requests to methods. | It is used to handle requests like GET, PUT, POST, and DELETE. |
Q32 What are the differences between @SpringBootApplication and @EnableAutoConfiguration annotation?
Features | @SpringBootApplication | @EnableAutoConfiguration |
When to Use | It is used when we want to use auto-configuration. | It is used when we want to customize auto-configuration. |
Entry Point | It is typically used on the main class of a Spring Boot application, serving as the entry point. | It can be used on any configuration class or with @SpringBootApplication. |
Component Scanning | It includes @ComponentScan annotation to enable component scanning. | It does not perform component scanning by itself. |
Example |
|
|
Q33 What are Profiles in Spring?
- You create sets of configurations (such as database URLs) for various scenarios (development, testing, production).
- Use the @Profile annotation to specify which configuration belongs where.
- Activate profiles using environment variables or command-line parameters.
To use Spring Profiles, simply define the spring.profiles.active property and select which profile to use.
Q34 What are the differences between WAR and embedded containers?
Feature | WAR | Embedded Containers |
Packaging | It contains all the files needed to deploy a web application to a web server. | It is a web application server included in the same JAR file as the application code. |
Configuration | It requires external configuration files (e.g., web.xml, context.xml) to define the web application. | It uses configuration properties or annotations within the application code. |
Security | It can be deployed to a web server that is configured with security features. | It can be made more secure by using security features provided by JRE. |
Q35 Spring Vs. Spring Boot?
- Spring is a framework that includes a variety of modules for developing enterprise-level applications.
- Spring Boot is a framework that makes Spring development easier by offering a pre-configured environment in which developers may focus on developing application logic.
Top 15 Spring Boot Interview Questions and Answers For Experienced.
Q36 What are the best practices for building a production-grade Spring Boot application?
Practice | Description |
Profiles | It is used to manage different settings for development, testing, and production environments. |
Security | It involves implementing HTTPS, OAuth2, and JWT to ensure secure communication. |
Monitoring | It is done using Actuator, Prometheus, and Grafana to monitor application health and performance effectively. |
Performance Optimization | It is achieved by fine-tuning database connections, caching strategies, and memory usage to enhance application performance. |
Q37 How do you implement microservices using Spring Boot?
Example
@SpringBootApplication
public class MyMicroserviceApplication {
public static void main(String[] args) {
SpringApplication.run(MyMicroserviceApplication.class, args);
}
}
Q38 What is Spring Cloud Config, and how is it used in Spring Boot?
Example Configuration
spring.cloud.config.uri=http://localhost:8888
Q39 How do you optimize the performance of a Spring Boot application?
Optimization Area | Techniques |
Database Connections | It is done by using connection pooling and lazy loading. |
Caching | It is implemented using @Cacheable and Redis. |
Startup Time | It is improved by using a spring-context-indexer to reduce startup time. |
Memory Usage | It is optimized by adjusting JVM settings and bean scopes. |
Q40 What is dependency injection, and what are its types?
- Constructor injection is the most used method of DI in Spring Boot, and constructor injection involves injecting the dependent object into the dependent object's constructor.
- Setter injection involves injecting the dependent object into the dependent object's setter method.
- Field injection involves injecting the dependent object into the dependent object's field.
Q41 What is the difference between Constructor and Setter Injection?
Features | Constructor Injection | Setter Injection |
Dependency | It is provided through constructor parameters. | It is set through setter methods after object creation. |
Immutability | It promotes immutability as dependencies are set at creation. | It allows dependencies to be changed dynamically after object creation. |
Dependency Overriding | It is harder to override dependencies with different implementations. | It allows easier overriding of dependencies using different setter values. |
Q42 What is the role of Spring Cloud Gateway in a Spring Boot microservices architecture?
Example
@SpringBootApplication
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
Example Configuration
spring:
cloud:
gateway:
routes:
- id: myService
uri: http://localhost:8081
predicates:
- Path=/service/**
Q43 How do you handle versioning in a Spring Boot application?
Example
@RestController
@RequestMapping("/api/v1")
public class MyController {
@GetMapping("/users")
public List getUsersV1() {
// Version 1 implementation
}
}
@RestController
@RequestMapping("/api/v2")
public class MyControllerV2 {
@GetMapping("/users")
public List getUsersV2() {
// Version 2 implementation
}
}
Q44 What differentiates Spring Data JPA and Hibernate?
- Hibernate, a Java Persistence API (JPA) implementation, enables Object-Relational Mapping (ORM) by allowing users to store, retrieve, map, and change application data across Java objects and relational databases.
- Hibernate converts Java data types to SQL (Structured Query Language) data types and Java classes to database tables, allowing developers to avoid scripting data persistence in SQL applications.
- Spring Data JPA, a Spring Data sub-project, provides abstraction over the DAL (Data Access Layer) by combining JPA and Object-Relational Mapping implementations like Hibernate.
- Spring Data JPA makes it easier to construct JPA repositories and aims to improve DAL implementation significantly.
Q45 How do you secure RESTful web services in Spring Boot?
Example
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/public").permitAll()
.anyRequest().authenticated()
.and()
.oauth2Login();
}
}
Q46 How do you handle transactions in Spring Boot?
- Spring Boot manages transactions with the @Transactional annotation.
- This annotation ensures that database actions within a method are carried out within a transaction.
- If an operation fails, all changes are reversed to ensure data consistency.
- Advanced transaction management allows you to configure propagation behavior, isolation level, and rollback rules.
Example
@Service
public class AccountService {
@Transactional
public void transferMoney(Long fromAccountId, Long toAccountId, BigDecimal amount) {
// Perform debit from source account
// Perform credit to destination account
}
}
Q47 How can you optimize the performance of a Spring Boot application?
Q48 What is Spring Boot's DevTools, and how does it enhance development productivity?
Q49 How do you externalize configuration in Spring Boot, and why is it important?
Example
# application.yml
server:
port: 8080
spring:
datasource:
url: jdbc:mysql://localhost:3306/mydb
username: user
password: pass
Q50 What are the different ways to test Spring Boot applications?
- Spring Boot apps can be tested using a variety of approaches: Unit testing focuses on testing individual components, such as services or repositories, utilizing frameworks such as JUnit and Mockit.
- This ensures that each component of the program works independently. Integration testing determines how various components of an application interact with one another.
- Spring Boot supports the @SpringBootTest annotation, which loads the entire application context for testing.
- Slice testing focuses on specific layers of the application (such as the web, service, and data layers).
- To test only the necessary parts of the application, use annotations such as @WebMvcTest, @DataJpaTest, and @RestClientTest. End-to-End Testing: Ensures that the entire application works as expected, which is commonly accomplished with automated UI testing tools such as Selenium or Cucumber.