11
AprUnderstanding Outer Join in SQL
Outer Join in SQL
What is an Outer Join in SQL?
- An Outer Join combines rows from two or more tables and includes all rows from one table and matched rows from the other table(s).
- The result includes rows with matches as well as those without matches from the specified table.
- If a row from one table doesn’t have a corresponding row in another, it’s still included, with null values for the unmatched columns.
- Useful for showing data even when relationships aren’t fully established, like customers with or without orders.
Read More: Different Types of SQL Joins
Types of Outer Joins
1. Left Outer Join in SQL: You will see all the rows from the left table and any matching rows from the right table. If there are no matches, the left table's rows will still show up, but the right table's values will be empty. This way, you keep all the data from the left side while getting related info from the right.
2. Right Outer Join in SQL: You will get all the rows from the right table, along with any matching rows from the left table. If there are no matches, the values on the left table will be empty. This join helps you keep all the data from the right side while also including related data from the left.
3. Full Outer Join in SQL: You will have all the rows from both tables, showing matched rows when available. If a row from one table doesn’t match any row in the other, it will still appear, with the missing side left empty. This join gives you a complete view of all data, so you won’t miss anything from either side.
Outer Join Syntax
SELECT column_name(s)
FROM table1
LEFT/RIGHT/FULL OUTER JOIN table2
ON table1.common_column = table2.common_column;
- SELECT: It specifies the columns you want to retrieve.
- FROM:It indicates the primary table.
- LEFT/RIGHT/FULL OUTER JOIN:It combines rows where the common column matches and includes unmatched rows.
- ON: Defines the condition between the tables using a common column.
Example Data
To demonstrate the various types of Outer Joins, let's use the following example data:
Customers Table
CustomerID | CustomerName |
---|---|
1 | Shailesh |
2 | Pradnya |
3 | Aman |
Orders Table
OrderID | CustomerID | Product |
---|---|---|
101 | 1 | Laptop |
102 | 2 | Smartphone |
103 | 4 | Tablet |
1. Left Outer Join Example
This query returns all customers' orders. If a customer has not placed an order, it will show with null values for order details.
SELECT Customers.CustomerName, Orders.Product
FROM Customers
LEFT OUTER JOIN Orders
ON Customers.CustomerID = Orders.CustomerID;
Left Outer Join Result
CustomerName | Product |
---|---|
Shailesh | Laptop |
Pradnya | Smartphone |
Aman | null |
2. Right Outer Join Example
This query returns all orders and the customers who placed them. If an order has no associated customer, it will show with null values for customer details.
SELECT Customers.CustomerName, Orders.Product
FROM Customers
RIGHT OUTER JOIN Orders
ON Customers.CustomerID = Orders.CustomerID;
Right Outer Join Result
CustomerName | Product |
---|---|
Shailesh | Laptop |
Pradnya | Smartphone |
null | Tablet |
3. Full Outer Join Example
This query returns all customers and all orders, including unmatched rows from both tables. If there are no matches, null values will fill the missing data.
SELECT Customers.CustomerName, Orders.Product
FROM Customers
FULL OUTER JOIN Orders
ON Customers.CustomerID = Orders.CustomerID;
Full Outer Join Result
CustomerName | Product |
---|---|
Shailesh | Laptop |
Pradnya | Smartphone |
Aman | null |
null | Tablet |
With Outer Joins, you can create meaningful reports and analyses, even when dealing with incomplete data. Mastering them will enable you to craft more comprehensive queries, keeping all your data relationships visible.
Outer Joins vs. Inner Joins
Join Type | Description |
Inner Join | Inner Join in SQL is used when one wants to retrieve only rows that possess matching values in both tables. If a row in one table does not have a matching row in the other table, that row will not appear in the result. Inner joins are helpful when you are interested only in data related to one another between two tables. |
Left Join | In the Left Join, all records return from the left table, and matching records, if any, return from the right table. In case of no match, the result set will contain the left table's row with NULLs for columns coming from the right table. |
Right Join | The opposite happens with a Right Join: it returns all the rows in the right table, placing NULLs when no match in the left table has been found. |
Full Outer Join | A Full Outer Join returns every row for both tables, with NULLs where no match exists. |
Key Difference | Inner joins give you just matched data, while outer joins ensure you don't miss data from either of the tables if there's no match. |
Example Explained
Let's use the same tables: Products and Orders.
- If we want to retrieve only the products that have been ordered, we would use an Inner Join:
SELECT Products.ProductName, Orders.OrderID
FROM Products
INNER JOIN Orders
ON Products.ProductID = Orders.ProductID;
Inner Join Output
Product Name | Order ID |
Product A | 1 |
Product B | 2 |
- Outer join in SQL with examples we have applied here. If we want to see all products regardless of whether they have been ordered, we will use a Left Outer Join:
SELECT Products.ProductName, Orders.OrderID
FROM Products
LEFT OUTER JOIN Orders
ON Products.ProductID = Orders.ProductID;
Left Outer Join Output
Product Name | Order ID |
Product A | 1 |
Product B | 2 |
Product C | NULL |
In this case, the result of the Left Outer Join would include all products, and for those without any corresponding orders, the OrderID
would be NULL.
CROSS JOIN in SQL
A CROSS JOIN in SQL returns the Cartesian product of the two tables, combining all rows from the first table with all rows from the second. Unlike Outer Joins, it doesn’t require a common column.
SELECT Customers.CustomerName, Orders.Product
FROM Customers
CROSS JOIN Orders;
Practical Applications of Outer Joins
- E-Commerce Applications: Display all customers and their orders, even if some customers haven’t made any purchases.
- Inventory Management: Combine product tables with supplier information, showing all products and their suppliers.
Best Practices for Using Outer Joins
- Understand Data Relationships: Knowing how your tables relate helps in constructing effective Outer Joins.
- Limit Result Sets: Use WHERE clauses to filter results when necessary to improve performance.
- Test Queries: Validate your joins with small datasets before applying them to larger datasets.
Advanced Outer Join Techniques
- Using Outer Joins with Aggregate Functions: Leverage functions like
SUM()
orCOUNT()
with Outer Joins.SELECT Customers.CustomerName, COUNT(Orders.OrderID) AS TotalOrders FROM Customers LEFT OUTER JOIN Orders ON Customers.CustomerID = Orders.CustomerID GROUP BY Customers.CustomerName;
Common Pitfalls and How to Avoid Them
- Ignoring NULL Values: Remember that unmatched rows result in NULL values; handle them in your application logic.
- Excessive Data Retrieval: Be cautious with Full Outer Joins, as they can produce large datasets; filter as needed.
Summary
FAQs
Take our Sqlserver skill challenge to evaluate yourself!
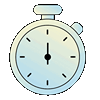
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.