18
AprArray in Typescript: An Easy Learning with Examples
Array in TypeScript
An array in TypeScript is similar to a container that contains several items of the same type in a defined order. Consider it a row of numbered boxes, each with a value that can be readily found, added, or changed. Arrays in TypeScript include useful capabilities for managing and working with lists of data. They maintain everything type-safe, which means they ensure that each item in the list contains the correct kind of data.
The TypeScript Tutorial will focus on learning what is an Array in TypeScript, creating Arrays in TypeScript, types of Arrays in TypeScript, Array Objects in TypeScript, accessing Array Elements in TypeScript, Type Safety with Arrays, Array methods in TypeScript, and a lot more
What is an Array in TypeScript?
In TypeScript, an array is used to store several elements of the same type in a specified sequence. Array in TypeScript allows you to structure data lists with type safety, guaranteeing that each item is of the required type. Using arrays in TypeScript allows you to conveniently organize, retrieve, and alter data sets in code.
Features of an Array in TypeScript
The array is an important technique for developers, as it allows them to store and manage various values in one place. Some important features of Array are:
- An array contains many values of the same type in a specified order.
- You can quickly retrieve elements in an array by their index.
- It provides you with different types of built-in methods for adding, deleting, and altering items.
- TypeScript enforces type safety in arrays, forbidding the usage of mismatched data types in TypeScript.
- As for your requirement, Arrays can be dynamically resized, increasing or decreasing as needed.
- To iterate over the entries in an array, use loops or array methods like forEach() loop in TypeScript.
Creating Arrays in TypeScript
You can create an array in TypeScript using the following methods:
1. Using Array Literal Syntax
In TypeScript, you may use literal syntax to define an array with values inside square brackets:
Example
let numbers: number[] = [1, 2, 3, 4, 5];
let fruits: string[] = ["apple", "banana", "cherry"];
2. Using the Array Constructor
You can create an array in TypeScript using the array constructor:
Example
let numbers: Array = new Array(1, 2, 3, 4, 5);
let fruits: Array = new Array("apple", "banana", "cherry");
Types of Arrays in TypeScript
There are two types of an array you should understand that are:
- Single Dimensional Array
- Multi-Dimensional Array (Matrix Form)
1. Single-Dimensional Array
A single-dimensional array is a basic list where elements are arranged in a single line. Each element can be accessed using a single index.
Syntax
let arrayName: dataType[] = [element1, element2, ...];
Example
let numbers: number[] = [10, 20, 30, 40];
console.log(numbers[2]); // Output: 30
Output
30
Explanation
The single-dimensional array, numbers, stores elements in a single row.
2. Multi-Dimensional Array
A multi-dimensional array is one that contains additional arrays, resulting in a structure similar to a matrix or table. A basic example is a two-dimensional array, which may be represented as rows and columns.
Syntax
let arrayName: dataType[][] = [
[row1Element1, row1Element2, ...],
[row2Element1, row2Element2, ...],
...
];
Example
let matrix: number[][] = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
console.log(matrix[1][2]); // Output: 6
Output
6
Explanation
The multi-dimensional array, matrix, organizes elements in rows and columns, where matrix[1][2] accesses the element in the second row and third column.
Read More - Typescript Interview Questions And Answers |
Array Objects in TypeScript
In TypeScript, an array is an object that contains numerous values of the same type in a predefined sequence, allowing for easy data management and manipulation using several built-in functions.
Importance of Array Objects
- Allows storage and easy access to multiple items in a single variable.
- Provides built-in methods to efficiently manage data (add, remove, search, etc.).
- Ensures type safety by restricting elements to a specified data type.
- Supports iteration for quick data processing and manipulation.
Syntax
1. Using square brackets:
let arrayName: dataType[] = [element1, element2, ...];
2. Using the Array constructor:
let arrayName: Array<dataType> = new Array(element1, element2, ...);
Example
let fruits: Array<string> = new Array('Apple', 'Banana', 'Mango');
fruits.push('Orange'); // Adds 'Orange' to the end
console.log(fruits); // Output: ['Apple', 'Banana', 'Mango', 'Orange']
fruits.pop(); // Removes the last element ('Orange')
console.log(fruits); // Output: ['Apple', 'Banana', 'Mango']
Output
[ 'Apple', 'Banana', 'Mango', 'Orange' ]
[ 'Apple', 'Banana', 'Mango' ]
Explanation
In this example:
- We create an array fruits with initial values.
- push() adds an element to the end, while pop() removes the last element.
Accessing Array Elements in TypeScript
1. Accessing Array Element by Index:
The index function in TypeScript allows you to retrieve individual items of an array. The index starts at 0. So, the first element has an index of 0.
Code Example:
let fruits: string[] = ['Apple', 'Banana', 'Orange', 'Grapes'];
let firstFruit = fruits[0]; // Accesses the first element
console.log('First Fruit:', firstFruit); // Output: Apple
Output
First Fruit: Apple
Explanation:
Here, fruits[0] access the first element of the array ('Apple'). The index 0 refers to the first element.
2. Using a for Loop to Iterate Through the Array
To iterate over an array, use the standard for loop. The loop goes from 0 to the array's length minus one, and you may access each element using the index.
Example
let fruits: string[] = ['Apple', 'Banana', 'Orange', 'Grapes'];
console.log('Fruits using for loop:');
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]); // Accesses each element by index
}
Output
Fruits using for loop:
Apple
Banana
Orange
Grapes
Explanation:
In the above example, Theloop start from0 to fruits.length - 1, and fruits[i] generate each element in the array by using its index.
3. Using forEach() Method to Access Array Elements
The forEach() method runs a given function one time for each item in the array. It gives both the element and its index in the callback.
Code Example:
let fruits: string[] = ['Apple', 'Banana', 'Orange', 'Grapes'];
console.log('Fruits using forEach:');
fruits.forEach((fruit, index) => {
console.log(`Index ${index}: ${fruit}`); // Accesses each element with its index
});
Output
Fruits using forEach:
Index 0: Apple
Index 1: Banana
Index 2: Orange
Index 3: Grapes
Explanation:
The forEach()method calls the provided function once for each element in the array. The callback gets the element (fruit)and its index (index), and we can access both inside the function.
4. Using for...of Loop to Iterate Over the Array:
The for...of loop iterates over the elements of an array without needing the index. It directly gives each element.
Code Example:
let fruits: string[] = ['Apple', 'Banana', 'Orange', 'Grapes'];
console.log('Fruits using for...of loop:');
for (let fruit of fruits) {
console.log(fruit); // Accesses each element directly
}
Output
Fruits using for...of loop:
Apple
Banana
Orange
Grapes
Explanation:
The for...of loop automatically iterates over the elements of the array without using the index. This is a simpler and more readable approach when you don’t need the index.
Modifying Array Elements in TypeScript
There are two ways to modify an element of an array in TypeScript that are:
- Modifying Elements Using Index
- Using Array Methods to Modify Elements
1. Modifying Elements Using Index
You can change an element in an array by directly accessing it with its index and assigning a new value.
Code Example:
let numbers: number[] = [10, 20, 30, 40];
numbers[1] = 25; // Changes the second element from 20 to 25
console.log(numbers); // Output: [10, 25, 30, 40]
Output
[ 10, 25, 30, 40 ]
Explanation
Here, we update the second element (index 1) of the array to 25.
2. Using Array Methods to Modify Elements
Using several Array methods to modify elements that are:
1. push() Method
Adds new elements to the end of the array.
let fruits: string[] = ['Apple', 'Banana'];
fruits.push('Orange'); // Adds 'Orange' at the end
console.log(fruits); // Output: ['Apple', 'Banana', 'Orange']
Output
[ 'Apple', 'Banana', 'Orange' ]
Explanation
We use push() to add 'Orange' to the end of the fruit array.
2. pop() Method
Removes the last element from the array.
let colors: string[] = ['Red', 'Green', 'Blue'];
colors.pop(); // Removes 'Blue'
console.log(colors); // Output: ['Red', 'Green']
Output
[ 'Red', 'Green' ]
Explanation
The pop() method removes the last element, 'Blue', from the colors array.
3. splice() Method
Adds or removes elements at any position in the array.
let animals: string[] = ['Dog', 'Cat', 'Rabbit'];
animals.splice(1, 1, 'Elephant'); // Replaces 'Cat' with 'Elephant'
console.log(animals); // Output: ['Dog', 'Elephant', 'Rabbit']
Output
[ 'Dog', 'Elephant', 'Rabbit' ]
Explanation
The splice() method removes 'Cat' and replaces it with 'Elephant' at index 1.
4. map() Method
Creates a new array by modifying each element in the original array according to a function you provide.
let prices: number[] = [100, 200, 300];
let discountedPrices = prices.map(price => price * 0.9); // Apply a 10% discount
console.log(discountedPrices); // Output: [90, 180, 270]
Output
[ 90, 180, 270 ]
Explanation
The map() method applies a 10% discount to each price, creating a new array.
Type Safety with Arrays
TypeScript's type safety assures that variables and arrays only contain values of the given type, preventing mistakes during compilation. For example, if an array is specified as a number[], only numbers may be added to it, which prevents type incompatibilities.
let ages: number[] = [25, 30, 35];
ages.push("forty"); // Error: Argument of type 'string' is not assignable to parameter of type 'number'.
Output
Argument of type '"forty"' is not assignable to parameter of type 'number'.
Type Checking, Index Signature, and Union Types in TypeScript
1. Type Checking
TypeScript’s type checking ensures variables, functions, and data structures hold only the types you specify. It provides errors at compile time, making your code safer by catching mismatches before runtime.
Example:
let age: number = 25;
age = "twenty-five"; // Error: Type 'string' is not assignable to type 'number'.
Explanation
Here, TypeScript catches the error of assigning a string to a number type, helping to avoid runtime issues.
2. Index Signatures
Index signatures allow you to define dynamic properties in an object where the names are unknown but the types are consistent. This is useful for objects where keys are not predetermined, but their value types are.
Syntax:
interface StringDictionary {
[key: string]: string;
}
const userRoles: StringDictionary = {
"admin": "John",
"guest": "Anika",
"moderator": "Raj"
};
Explanation
The StringDictionary interface ensures that any property with a string key in userRoles will have a string value. TypeScript will enforce this rule, preventing accidental assignment of other types.
3. Union Types
Union types allow a variable to hold multiple specified types. This is useful when a value could be one of several types, giving flexibility while still retaining type safety.
Syntax
let id: number | string;
id = 101; // Valid
id = "user-101"; // Also valid
id = true; // Error: Type 'boolean' is not assignable to type 'number | string'.
Explanation
In this example, id can be either a number or a string, but not any other type. TypeScript enforces that id will only accept values that are number or string, reducing errors from unexpected types.
TypeScript Interfaces and Object Literals
TypeScript Interfaces
An interface in TypeScript is similar to a blueprint for an object. It specifies what properties and methods the object should have, as well as their kinds. Using interfaces ensures that objects have a particular structure, making your code more stable and understandable.
Example
interface Person {
name: string;
age: number;
greet(): void;
}
const person: Person = {
name: "Rahul",
age: 30,
greet() {
console.log("Hello, my name is " + this.name);
}
}; person.greet(); //calling the function
Output
Hello, my name is Rahul
Explanation
The Person interface defines the structure an object must follow, including name (a string), age (a number), and a greet method. The person object adheres to this structure, making TypeScript enforce its validity at compile time.
Object Literals
Object literals are simply objects defined with a fixed structure directly in your code. When combined with interfaces, object literals become type-checked, meaning TypeScript will ensure they match the interface's requirements.
Example
interface Car {
brand: string;
model: string;
year: number;
}
const myCar: Car = {
brand: "Toyota",
model: "Corolla",
year: 2020
};
Explanation
The Car interface defines what properties an object must have, along with their types. The myCar object literal matches the Car interface, so TypeScript confirms that it’s structured correctly.
Benefits of Using Interfaces with Object Literals
- Type Safety: Ensures objects meet specific structural requirements, reducing runtime errors.
- Code Readability: Provides clear structure and expectations for data.
- Easier Maintenance: Changes in the interface are immediately reflected throughout the code where objects use that interface.
Using interfaces with object literals in TypeScript is a powerful way to ensure consistent and predictable data structures, making your codebase more robust and easier to understand.
Array Methods in TypeScript
Here are the several array methods used in TypeScript:
Method | Description |
push() | Adds one or more elements to the end of the array. |
pop() | Removes the last element from the array. |
shift() | Removes the first element from the array. |
unshift() | Adds one or more elements to the beginning of the array. |
concat() | Combines two or more arrays into one new array. |
map() | Creates a new array with the results of a function applied to each element. |
filter() | Creates a new array with all elements that pass the test. |
reduce() | Executes a function on each element, returning a single value. |
forEach() | Executes a provided function once for each element. |
find() | Returns the first element that satisfies the provided testing function. |
includes() | Check if an array contains a specific element. |
some() | Tests whether at least one element passes the test. |
every() | Tests whether all elements pass the test. |
Compare With: An Easy Solution for Array Methods in JavaScript
Conclusion
Finally, arrays in TypeScript provide an organized mechanism for managing collections of data while ensuring type safety, making development more predictable. TypeScript array methods provide strong data manipulation features, including sorting, filtering, and mapping. Using TypeScript array methods allows developers to produce efficient and maintainable code while fully using the benefits of TypeScript arrays.
Further Read |
Access Modifiers in TypeScript Explained |
Understanding Functions in TypeScript |
Generics in TypeScript |
FAQs
Take our Typescript skill challenge to evaluate yourself!
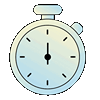
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.