21
FebUnderstanding Arrays and Tuples in TypeScript
Arrays and Tuples in TypeScript
Arrays and Tuples in TypeScript, are two of the most frequent methods for storing multiple variables. But do you know when to use each? Arrays are extremely flexible, allowing you to store lists of data such as integers, characters, and objects. Tuples, on the other hand, provide greater structure by allowing you to store a fixed number of items, each of which is of a specific type.
In this TypeScript Tutorial, We are gonna explore Arrays and Tuples in TypeScript, Let's break them down so you can see how each one works and when to use them!
Arrays in TypeScript
1. Defining Arrays
In TypeScript, arrays can be defined in two primary ways:
- Using Square Bracket Syntax (
type[]
) - Using Generic Array Type (
Array<type>
)
1. Square Bracket Syntax
let numbers: number[] = [1, 2, 3, 4, 5];
let fruits: string[] = ["Apple", "Banana", "Cherry"];
2. Generic Array Type
let numbers: Array<number> = [1, 2, 3, 4, 5];
let fruits: Array<string> = ["Apple", "Banana", "Cherry"];
2. Array Types
TypeScript allows arrays to be homogeneous or heterogeneous, although homogeneous arrays are more common due to type safety.
1. Homogeneous Arrays
All elements in the array are of the same type.
let scores: number[] = [95, 85, 76, 88];
2. Heterogeneous Arrays
Elements can be of different types using union types.
let mixed: (number | string)[] = [1, "two", 3, "four"];
3. Array Methods
Method Name | Description |
push() | This method Adds new elements to the array and returns the new array length. |
pop() | It Removes the last element of the array and returns that element |
sort() | It sorts all the elements of the array |
concat() | It Joins two arrays and returns the combined result |
indexOf() | This method returns the index of the first match of a value in the array (-1 if not found) |
copyWithin() | It Copies a sequence of elements within the array |
fill() | As its name, it fills the array with a static value from the provided start index to the end index |
shift() | It removes and returns the first element of the array |
splice() | Splice adds or removes elements from the array |
unshift() | It adds one or more elements to the beginning of the array |
includes() | This method checks whether the array contains a certain element |
join() | It joins all elements of the array into a string |
lastIndexOf() | Returns the last index of an element in the array |
slice() | It extracts a section of the array and returns the new array |
toString() | It returns a string representation of the array |
toLocaleString() | It returns a localized string representing the array |
Let's elaborate on this, By the way, TypeScript supports all standard JavaScript array methods, with type safety enforced based on the array's defined type.
1. Push
Adds a new element to the end of the array.
Example
let numbers: number[] = [1, 2, 3];
// Add a single number
numbers.push(4);
// Add multiple numbers
numbers.push(5, 6);
console.log(numbers);
Output
[ 1, 2, 3, 4, 5, 6 ]
2. Pop
Removes the last element from the array.
Example
let numbers: number[] = [10, 20, 30, 40];
// Remove the last element
let lastNumber = numbers.pop();
console.log("Removed Element:", lastNumber); // Output: Removed Element: 40
console.log("Updated Array:", numbers);
Output
Removed Element: 40
Updated Array: [ 10, 20, 30 ]
3. Map
Transforms each element in the array.
Example
let numbers: number[] = [1, 2, 3, 4, 5];
// Double each number
let doubledNumbers = numbers.map(num => num * 2);
console.log("Original Array:", numbers); // Output: [1, 2, 3, 4, 5]
console.log("Doubled Array:", doubledNumbers); // Output: [2, 4, 6, 8, 10]
Output
Original Array: [ 1, 2, 3, 4, 5 ]
Doubled Array: [ 2, 4, 6, 8, 10 ]
4. Filter
Filters elements based on a condition.
Example
let numbers: number[] = [10, 15, 20, 25, 30];
// Filter numbers greater than 20
let filteredNumbers = numbers.filter(num => num > 20);
console.log("Original Array:", numbers); // Output: [10, 15, 20, 25, 30]
console.log("Filtered Array:", filteredNumbers); // Output: [25, 30]
console.log("Doubled Array:", doubledNumbers); // Output: [2, 4, 6, 8, 10]
Output
Original Array: [ 10, 15, 20, 25, 30 ]
Filtered Array: [ 25, 30 ]
5. Reduce
Reduces the array to a single value.
Example
let numbers: number[] = [1, 2, 3, 4, 5];
// Calculate the sum of all numbers
let sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log("Original Array:", numbers); // Output: [1, 2, 3, 4, 5]
console.log("Sum of Numbers:", sum); // Output: 15
Output
Original Array: [ 1, 2, 3, 4, 5 ]
Sum of Numbers: 15
Some Important Examples
Example 1: Array of Objects
An array of objects in TypeScript is a collection of items where each item is an object with a defined structure, typically using interfaces or types to enforce consistency.
interface Person {
id: number;
name: string;
age: number;
}
let people: Person[] = [
{ id: 1, name: "Alice", age: 25 },
{ id: 2, name: "Bob", age: 30 },
{ id: 3, name: "Charlie", age: 35 }
];
console.log(people);
Output
[
{ id: 1, name: 'Alice', age: 25 },
{ id: 2, name: 'Bob', age: 30 },
{ id: 3, name: 'Charlie', age: 35 }
]
Example 2: Array with Union Types
An array of union types in TypeScript allows an array to contain elements of different types, such as number | string, enabling multiple data types within a single array.
let mixedArray: (number | string)[] = [1, "hello", 2, "world", 3];
console.log(mixedArray);
Output
[ 1, 'hello', 2, 'world', 3 ]
Read More - Typescript Interview Questions And Answers |
Accessing Array Elements in TypeScript
In TypeScript, accessing elements of an array works similarly to JavaScript since TypeScript is a superset of JavaScript. You can use the index of the array to access its elements. Here's a quick guide on how to access array elements in TypeScript:
Example
let numbers: number[] = [10, 20, 30, 40, 50];
// Accessing the first element
console.log(numbers[0]); // Output: 10
// Accessing the third element
console.log(numbers[2]); // Output: 30
// Accessing the last element
console.log(numbers[numbers.length - 1]); // Output: 50
Output
10
30
50
Key Points:
- Arrays in TypeScript are zero-indexed, meaning the first element is at index
0
. - You can use the
length
property to dynamically access the last element by subtracting 1 from the array length. - TypeScript ensures that you are working with the correct type of elements when accessing or modifying an array. For example, if you define an array of numbers (
number[]
), you can't accidentally assign a string to an element without getting a compilation error.
Tuples in TypeScript
Defining Tuples
Tuples are ordered collections of elements with fixed types and length. They are particularly useful when the data structure represents a fixed pattern.
let user: [number, string];
user = [1, "Alice"]; // Correct
// user = ["Alice", 1]; // Error: Type 'string' is not assignable to type 'number'.
Tuple Types
Each element in a tuple has a designated type and position, making tuples distinct from regular arrays.
let coordinate: [number, number] = [10, 20];
let product: [number, string, boolean] = [101, "Laptop", true];
Readonly Tuple
// define our tuple
let ourTuple: [number, boolean, string];
// initialize correctly
ourTuple = [5, true, 'Welcome to Scholarhat']
// We have no type safety in our tuple for indexes 3+
ourTuple.push('Something new and wrong');
console.log(ourTuple);
Output
[ 5, true, 'Welcome to Scholarhat', 'Something new and wrong' ]
Named Tuples
const graph: [x: number, y: number] = [55.2, 41.3];
Accessing Tuple Elements
Elements in a tuple are accessed by their index, similar to arrays.
let firstId = user[0]; // firstId is number
let userName = user[1]; // userName is string
Heterogeneous Data Types in Tuples
Tuple Examples
Example 1: Returning Multiple Values from a Function
function getUserInfo(): [number, string] {
return [1, "Alice"];
}
let [id, name] = getUserInfo();
Example 2: Defining a Key-Value Pair
type KeyValue = [string, number];
let pair: KeyValue = ["age", 30];
Differences Between Arrays and Tuples
While both arrays and tuples store collections of elements, they differ in structure and use cases.
Feature | Arrays | Tuples |
---|---|---|
Type Flexibility | Typically homogeneous with uniform types | Can have heterogeneous types per position |
Length | Variable length | Fixed length |
Use Cases | Lists of items, collections, iteration | Representing structured data, multiple return values |
Type Enforcement | Enforces element types uniformly | Enforces type and order for each element |
Mutability | Elements can be added or removed dynamically | Limited modifications beyond the defined structure |
Use Cases
When to Use Arrays
- Lists of Similar Items: Managing collections like lists of users, products, or numbers.
- Iteration: Performing operations like mapping, filtering, and reducing over collections.
- Dynamic Data: When the number of elements can change over time.
When to Use Tuples
- Structured Data: Representing data with a fixed structure, such as database records or configurations.
- Function Returns: Returning multiple values from functions in a type-safe manner.
- Destructuring: Assigning multiple variables from a single data structure with known types.
Advanced Features and Best Practices
Readonly Arrays and Tuples
TypeScript allows defining arrays and tuples as read-only to prevent modification.
let readonlyNumbers: readonly number[] = [1, 2, 3];
// readonlyNumbers.push(4); // Error: Property 'push' does not exist on type 'readonly number[]'.
let readonlyUser: readonly [number, string] = [1, "Alice"];
// readonlyUser[0] = 2; // Error: Cannot assign to '0' because it is a read-only property.
Spread Operator with Tuples
Tuples can be combined using the spread operator, maintaining type integrity.
let tuple1: [number, string] = [1, "Alice"];
let tuple2: [boolean] = [true];
let combined: [number, string, boolean] = [...tuple1, ...tuple2];
Optional and Rest Elements in Tuples
Example
// Tuple with an optional element
let tupleWithOptional: [number, string?, boolean?] = [1, "hello"]; // Both the second and third elements are optional
console.log(tupleWithOptional);
// Tuple with rest elements
let tupleWithRest: [number, ...string[]] = [1, "apple", "banana", "cherry"];
console.log(tupleWithRest);
Output
[ 1, 'hello' ]
[ 1, 'apple', 'banana', 'cherry' ]
Best Practices
- Prefer Arrays for Homogeneous Data: Use arrays when dealing with lists of similar items.
- Use Tuples for Fixed Structures: Utilize tuples for fixed-size collections with known types.
- Type Inference: Allow TypeScript to infer types when possible to reduce redundancy.
- Employ Readonly Types: Use
readonly
to prevent unintended mutations. - Document Tuple Structures: Clearly document the meaning of each tuple element for better code readability.
Conclusion
Arrays and tuples in typescript are indispensable data structures, each serving distinct purposes. Arrays offer flexibility and are ideal for collections of similar items, while tuples provide structure and type safety for fixed-size, heterogeneous data. Understanding their differences, use cases, and best practices enables developers to write more robust and maintainable TypeScript code.
FAQs
Take our Typescript skill challenge to evaluate yourself!
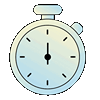
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.