04
JulUnderstanding DataTypes in TypesScript
Data Types in TypeScript
TypeScript Datatypes are one of TypeScript's most interesting features. TypeScript allows you to categorize the types of data your variables can carry, just as we do in real life (such as apples, oranges, and cars).
Imagine you're working on a project and want to make sure the values you're using are exactly what you expect, no surprises! This is where TypeScript's data types come in. They help you decide if a variable should retain a number, a string of text, or something more sophisticated, such as an object. This not only makes your code clearer but also detects any issues before you execute the application.
So, in this TypeScript Tutorial, we gonna explore different Data Types in TypeScript, including the following concepts.
Introduction to TypeScript Data Types
Data types in TypeScript allow developers to specify the kind of data a variable can hold. This static typing capability helps catch errors at compile time, leading to more reliable and maintainable code.
Data Type | Keyword | Description |
---|---|---|
Number | number | It is used to represent both Integer as well as Floating-Point numbers |
Boolean | boolean | Represents true and false |
String | string | It is used to represent a sequence of characters |
Void | void | Generally used for function return types |
Null | null | It is used when an object does not have any value |
Undefined | undefined | Denotes the value given to an uninitialized variable |
Any | any | If a variable is declared with any data type, then any type of value can be assigned to that variable |
Primitive Data Types
Primitive types are the basic building blocks in TypeScript. They include:
1 Number
The number
type can represent both integer and floating-point numbers.
let age: number = 30;
let price: number = 19.99;
2 String
The string
type is used for textual data and can be enclosed in single quotes, double quotes, or backticks (template literals).
let name: string = "Alice";
let greeting: string = `Hello, ${name}!`;
3 Boolean
The boolean
type represents a logical value that can be either true
or false
.
let isActive: boolean = true;
4 Null
The null
type represents the intentional absence of any object value.
let user: null = null;
5 Undefined
The undefined
type is used for uninitialized variables.
let uninitialized: undefined;
Read More - Typescript Interview Questions And Answers For Freshers |
Object Data Types
Object types are more complex structures that can hold multiple values. Some common object types include:
1 Object
The object
type represents a non-primitive type that can hold various properties.
let person: object = {
name: "John",
age: 25
};
2 Array
An Array in typescript
can hold a collection of values of a specific type. You can define an array using the syntax type[]
or Array<type>
.
let numbers: number[] = [1, 2, 3, 4];
let strings: Array = ["apple", "banana", "cherry"];
3 Tuple
A Tuple
represents an array with a fixed number of elements, each of which can have a different type.
let tuple: [string, number] = ["Alice", 30];
Special Types
TypeScript provides special types to handle specific scenarios:
1 Any Type
The any
type allows any value, effectively disabling type checking for that variable.
let variable: any = "Hello";
variable = 5; // Still valid
2 Unknown
The unknown
type is safer than any
. You cannot perform operations on an unknown
type until you perform type checking.
let value: unknown = 5;
// console.log(value.toFixed(2)); // Error: Object is of type 'unknown'
3 Void
The void
type is used for functions that do not return a value.
function logMessage(message: string): void {
console.log(message);
}
4 Never
The never
type represents a type that never occurs, such as a function that always throws an error.
function throwError(message: string): never {
throw new Error(message);
}
Other DataTypes
TypeScript allows developers to define custom types using interfaces and type aliases.
1 Interfaces
Interfaces define the structure of an object by specifying its properties and methods.
interface Person {
name: string;
age: number;
}
2 Type Aliases
Type aliases create a new name for a type, which can be useful for unions and intersections.
type StringOrNumber = string | number;
3. Symbols
A symbol is a new, primitive data type introduced in ES6 just like number and string. A symbol value is created by calling the Symbol constructor.let s1 = Symbol();
let s2 = Symbol("mySymbol");
let s1 = Symbol("mySymbol Description");
let s2 = Symbol("mySymbol Description");
s1 === s2; // false, symbols are unique
- Symbol.hasInstance - Used to determine whether an object is one of the constructor’s instances.
- Symbol. match - Used for matching the regular expression against a string.
- Symbol. iterator - Returns the default iterator for an object and is called by the for-of loop.
- Symbol. search - Returns the index number within a string that matches the regular expression.
- Symbol. split - Splits a string at the indices that match the regular expression.
4. Enum
An enum is a way to give friendly names to a set of numeric values. The enum keyword is used to define the Enum type in TypeScript. By default, the value of the enum’s members starts from 0. But you can change this by setting the value of one of its members. In TypeScript, two types of enums are supported which are string and number enum where the number enum can be auto-incremented whereas the string enum does not support auto-incremented values.enum Courses {TypeScript, Ionic, Angular2, NodeJS};
let tscourse: Courses = Courses.TypeScript;
console.log(tscourse); //0
Output
0
The compiled JavaScript (ES5) code for the above TypeScript code is given below:var Courses;
(function (Courses) {
Courses[Courses["TypeScript"] = 0] = "TypeScript";
Courses[Courses["Ionic"] = 1] = "Ionic";
Courses[Courses["Angular2"] = 2] = "Angular2";
Courses[Courses["NodeJS"] = 3] = "NodeJS";
})(Courses || (Courses = {}));
;
var tscourse = Courses.TypeScript;
console.log(tscourse); //0
Output
0
In the previous example, we can start the enum’s members value from 2 instead of 0.enum Courses {TypeScript=2, Ionic, Angular2, NodeJS};
let tscourse: Courses = Courses.TypeScript;
console.log(tscourse); //2
Output
2
The compiled JavaScript (ES5) code for the above TypeScript code is given below:var Courses;
(function (Courses) {
Courses[Courses["TypeScript"] = 2] = "TypeScript";
Courses[Courses["Ionic"] = 3] = "Ionic";
Courses[Courses["Angular2"] = 4] = "Angular2";
Courses[Courses["NodeJS"] = 5] = "NodeJS";
})(Courses || (Courses = {}));
;
var tscourse = Courses.TypeScript;
console.log(tscourse); //2
Output
2
An enum type can be assigned to the Number primitive type, and vice versa.enum Courses { TypeScript = 2, Ionic, Angular2, NodeJS };
let tscourse: Courses = Courses.Ionic;
let c: number = tscourse; //3
var Courses;
(function (Courses) {
Courses[Courses["TypeScript"] = 2] = "TypeScript";
Courses[Courses["Ionic"] = 3] = "Ionic";
Courses[Courses["Angular2"] = 4] = "Angular2";
Courses[Courses["NodeJS"] = 5] = "NodeJS";
})(Courses || (Courses = {}));
;
var tscourse = Courses.Ionic;
var c = tscourse;
Conclusion
TypeScript's data types help developers write more robust and maintainable code. By leveraging primitive, object, and user-defined types, you can ensure that your variables and functions are accurately typed, significantly reducing the likelihood of runtime errors. Understanding these data types in TypeScript is essential for building effective TypeScript applications and can greatly enhance your development experience.
FAQs
The typeof operator is a straightforward way to determine the data type of a value. It returns a string representing the type, such as “string,” “number,” or “boolean.”
Take our Typescript skill challenge to evaluate yourself!
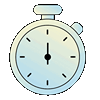
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.