04
JulUnderstanding Functions in TypeScript
Functions in TypeScript
Functions in typescript are an integral part of any programming language and are equally important. Consider a function to be a reusable chunk of code that carries out a given activity. TypeScript allows you to define functions in a way that extends the usual JavaScript technique by including type annotations, which assist ensure that the correct kinds of values are utilized.
So In this TypeScript Tutorial, We will see the different types of Functions in typescript including their syntaxes and examples.
What are the Functions in TypeScript
- Here, Functions in TypeScript are reusable code blocks that execute specific tasks.
- You can define a function using the 'function' keyword, and TypeScript lets you specify parameter types and return types for added type safety.
- This means that if a function expects a number as a parameter, TypeScript will enforce it, allowing you to catch mistakes during development.
- TypeScript also supports features such as optional arguments, default parameters, and rest parameters, making it easier to write versatile and powerful functions for your applications.
Basic Function Syntax
function greet(name: string): string {
return `Hello, ${name}!`;
}
console.log(greet("Welcome to Scholarhat..!"));
Output
Hello, Welcome to Scholarhat..!!
In this example:
name: string
specifies that thename
parameter must be a string.: string
after the parameter list indicates that the function returns a string.
Function Types
TypeScript allows you to define various aspects of functions using type annotations, enhancing code reliability and readability.
1. Typed Parameters and Return Types
By specifying types for function parameters and return values, TypeScript ensures that functions are used correctly.
Example
function add(a: number, b: number): number {
return a + b;
}
console.log(add(5, 3));
Output
8
2. Optional and Default Parameters
1. Optional Parameters
Optional parameters are denoted by a ?
after the parameter name. They may or may not be provided when the function is called.
Example
function buildName(firstName: string, lastName?: string): string {
if (lastName) {
return `${firstName} ${lastName}`;
} else {
return firstName;
}
}
console.log(buildName("John"));
console.log(buildName("John", "Doe"));
Output
John
John Doe
2. Default Parameters
Default parameters allow you to specify a default value for a parameter if none is provided.
Example
function greet(name: string, greeting: string = "Hello"): string {
return `${greeting}, ${name}!`;
}
console.log(greet("Welcome to Scholarhat..!"));
console.log(greet("Welcome to Scholarhat..!", "Hi"));
Output
Hello, Welcome to Scholarhat..!!
Hi, Welcome to Scholarhat..!!
3. Rest Parameters
Rest parameters allow functions to accept an indefinite number of arguments as an array.
Example
function sum(...numbers: number[]): number {
return numbers.reduce((acc, curr) => acc + curr, 0);
}
console.log(sum(1, 2, 3, 4)); // Output: 10
Output
10
Function Signatures
Function signatures define the type of functions, specifying the parameter types and return type without providing the actual implementation. They are particularly useful when defining interfaces or type aliases for functions.
1. Using Interfaces
Example
interface StringGenerator {
(length: number): string;
}
const generateString: StringGenerator = (length: number): string => {
return "a".repeat(length);
};
console.log(generateString(5));
Output
aaaaa
2. Using Type Aliases
Example
type MathOperation = (x: number, y: number) => number;
const multiply: MathOperation = (a, b) => a * b;
console.log(multiply(4, 5)); // Output: 20
Output
20
Function Overloading
Function overloading allows you to define multiple signatures for a single function, enabling different ways to call it based on the number or types of parameters.
Example of Function Overloading
function combine(a: string, b: string): string;
function combine(a: number, b: number): number;
function combine(a: any, b: any): any {
if (typeof a === "string" && typeof b === "string") {
return a + b;
} else if (typeof a === "number" && typeof b === "number") {
return a + b;
}
}
console.log(combine("Hello, ", "World!")); // Output: Hello, World!
console.log(combine(10, 20)); // Output: 30
Output
Hello, World!
30
Read More - Typescript Interview Questions And Answers |
The this
Keyword in Functions
Handling the this
keyword in TypeScript functions can be tricky, especially when dealing with different contexts. TypeScript provides type annotations to specify the type of this
within functions.
Typing this
interface Point {
x: number;
y: number;
move(this: Point, dx: number, dy: number): void;
}
const point: Point = {
x: 0,
y: 0,
move(dx: number, dy: number) {
this.x += dx;
this.y += dy;
},
};
point.move(5, 10);
console.log(point);
Output
{ x: 5, y: 10, move: [Function: move] }
Arrow Functions
Arrow functions offer a concise syntax and lexically bind the this
keyword, making them suitable for scenarios where you want to maintain the context of this
.
Example of Arrow Functions
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map((num) => num * 2);
console.log(doubled); // Output: [2, 4, 6, 8, 10]
Output
[ 2, 4, 6, 8, 10 ]
Higher-Order Functions
Higher-order functions are functions that take other functions as arguments or return functions as their results. TypeScript's type annotations enhance the reliability of higher-order functions by ensuring that the passed functions adhere to expected signatures.
Example of a Higher-Order Function
function operate(a: number, b: number, operation: (x: number, y: number) => number): number {
return operation(a, b);
}
const add = (x: number, y: number): number => x + y;
const subtract = (x: number, y: number): number => x - y;
console.log(operate(10, 5, add)); // Output: 15
console.log(operate(10, 5, subtract)); // Output: 5
Output
15
5
Practical Examples
1. Simple Function Example
function greet(name: string): string {
return `Hello, ${name}!`;
}
console.log(greet("Bob")); // Output: Hello, Bob!
Output
Hello, Bob!
2. Function with Optional and Default Parameters
function createUser(username: string, age?: number, isAdmin: boolean = false): string {
return `User: ${username}, Age: ${age ?? "N/A"}, Admin: ${isAdmin}`;
}
console.log(createUser("Welcome to Scholarhat..!")); // Output: User: Welcome to Scholarhat..!, Age: N/A, Admin: false
console.log(createUser("Bob", 30, true)); // Output: User: Bob, Age: 30, Admin: true
Output
User: Welcome to Scholarhat..!, Age: N/A, Admin: false
User: Bob, Age: 30, Admin: true
3. Function Overloading Example
function merge(a: string, b: string): string;
function merge(a: number, b: number): number;
function merge(a: any, b: any): any {
if (typeof a === "string" && typeof b === "string") {
return a + b;
} else if (typeof a === "number" && typeof b === "number") {
return a + b;
}
}
console.log(merge("Hello, ", "World!")); // Output: Hello, World!
console.log(merge(10, 20)); // Output: 30
Output
Hello, World!
30
Conclusion
Finally, TypeScript functions are effective tools for improving code organization and reusability while also ensuring type safety. TypeScript allows developers to specify parameter and return types, which helps detect potential issues early and leads to more robust programs. The addition of optional and default parameters increases flexibility, making it easier to handle a variety of use cases. Overall, learning TypeScript functions is critical for producing efficient and maintainable code in your projects.
FAQs
Take our Typescript skill challenge to evaluate yourself!
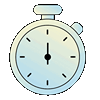
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.