18
AprUnderstanding Modules and Namespaces in TypeScript
Modules and Namespaces in TypeScript
Modules and namespaces in TypeScript help organize code in large applications. Modules allow you to split code into separate files, making it easier to manage and reuse, while namespaces group-related code under a single name to avoid conflicts. Modules are preferred in modern TypeScript, but namespaces can still be useful for organizing complex projects.
In this Typescript tutorial, we will learn the concept of Modules and Namespaces in TypeScript including its implementation.
What Are Modules?
Modules are a way to encapsulate code in TypeScript. They allow you to split your code into separate files, making it easier to manage and maintain. Each module can export variables, functions, classes, or interfaces, making them available for import in other modules. This approach helps prevent global namespace pollution and promotes code reusability.
- A module is an approach to define a collection of linked variables, functions, classes, interfaces, and so on.
- It runs in the local scope rather than the global scope.
- In other words, variables, functions, classes, and interfaces declared in a module cannot be directly accessed outside of the module.
- We can use the export keyword to construct a module that we can then utilize in other modules by using the import keyword.
Example of Modules in Typescript
//math.tsexport
function add(a: number, b: number): number { return a + b;}
// app.tsimport { add } from "./math";
const result = add(5, 7); // result will be 12
console.log(result);
Output
12
math.ts defines a module containing an add function that is exported to other modules. App.ts imports and uses the add function from math.ts, showing modularity and code reuse.Creating a Module
To create a module, simply use the export
keyword to expose the components you want to share. For example, consider a module named math.ts
:
// math.ts
export function add(a: number, b: number): number {
return a + b;
}
export function subtract(a: number, b: number): number {
return a - b;
}
In this module, we define two functions, add
and subtract
, and export them. Now, you can import these functions in another file:
// main.ts
import { add, subtract } from './math';
console.log(add(5, 3)); // Output: 8
console.log(subtract(5, 3)); // Output: 2
Default Exports
Modules can also have a default export, which simplifies importing a single component. You can define a default export like this:
// calculator.ts
export default function multiply(a: number, b: number): number {
return a * b;
}
You can then import it without curly braces:
// main.ts
import multiply from './calculator';
console.log(multiply(5, 3)); // Output: 15
Read More - Typescript Interview Questions And Answers For Freshers |
What Are Namespaces?
Namespaces (previously known as internal modules) are a way to organize code within a single file or across multiple files without using modules. They are used primarily for grouping related code, which can help reduce naming collisions and provide a clear structure for large applications.
Creating a Namespace
To create a namespace, use the namespace
keyword. Here’s an example:
namespace Geometry {
export function areaOfCircle(radius: number): number {
return Math.PI * radius * radius;
}
export function areaOfSquare(side: number): number {
return side * side;
}
}
In this example, we define a namespace called Geometry
that contains two functions, areaOfCircle
and areaOfSquare
. Both functions are exported, making them accessible outside the namespace.
Using a Namespace
To use the functions from a namespace, simply reference them with the namespace name:
console.log(Geometry.areaOfCircle(5)); // Output: 78.53981633974483
console.log(Geometry.areaOfSquare(4)); // Output: 16
Differences Between Modules and Namespaces
While both modules and namespaces help organize code, there are key differences:
- Scope:
- Modules have their own scope and are loaded at runtime. They can be used in any JavaScript environment, including Node.js and browsers.
- Namespaces exist only at compile time and are typically used for organizing code within the same file or across multiple files.
- Loading Mechanism:
- Modules require a module loader (like Webpack, RequireJS, or Node.js) to manage dependencies.
- Namespaces do not require any loader; they are a simple way to group code.
- Export/Import Syntax:
- In modules, you use
export
andimport
keywords to share and access components. - In namespaces, you reference the namespace directly to access its members.
- In modules, you use
When to Use Modules and Namespaces
Use Modules when:
- You need to share code across multiple files.
- You're building applications that will be run in a modular environment (e.g., Node.js, modern browsers).
- You want to take advantage of tree-shaking capabilities in bundlers to reduce the final bundle size.
Use Namespaces when:
- You have a large application with a lot of related functionality that you want to group logically.
- You want to avoid naming conflicts without using modules, particularly in legacy projects or smaller applications.
Conclusion
Both modules and namespaces are essential tools for organizing and managing code in TypeScript. While modules are the preferred way to structure code in modern applications due to their flexibility and compatibility with JavaScript environments, namespaces still offer a valuable way to organize code, especially in larger projects. Understanding when and how to use these features will help you write cleaner, more maintainable TypeScript code, ultimately leading to better software development practices. Happy coding!
FAQs
Take our Typescript skill challenge to evaluate yourself!
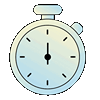
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.