18
AprReact with TypeScript: A Complete Guide for Developers
React TypeScript
React with TypeScript is ideal for developers who want to build scalable, maintainable, and type-safe applications. Imagine combining React's powerful UI-building capabilities with TypeScript’s strict type-checking, allowing you to catch errors early and write clearer, more predictable code. This combination helps you develop dynamic, component-based applications efficiently while maintaining code quality and consistency.
In this Typescript tutorial, I’ll explain how React and TypeScript work together to simplify application development, why type safety matters, and how mastering this combination can boost your productivity and code reliability.
Why Use TypeScript with React?
Using TypeScript with React provides several benefits:
- TypeScript's Type: Safety feature ensures that data sent between components is of the correct kind. This boosts your code's confidence and lowers runtime errors.
- Improved Developer Experience: TypeScript allows for safer code refactoring, intelligent code completion, and improved error handling. Type-based documentation and tips can be found in IDEs.
- Scalability: By enforcing types and giving functions and components more defined intent, TypeScript keeps your codebase manageable and scalable as your React application expands.
- Early Error Detection: TypeScript detects errors early in the development process rather than waiting till the application is live.
How to Use TypeScript with React
Setting Up React with TypeScript
If you're starting a new project, setting up React with TypeScript is straightforward. You can use the create-react-app
command-line tool, which now supports TypeScript out of the box.
1. Setting Up a New Project with create-react-app
npx create-react-app my-app --template typescript
This command creates a new React app with TypeScript pre-configured. The file extensions will be .tsx
(for components) and .ts
(for other TypeScript files).
2. Adding TypeScript to an Existing React Project
If you have an existing React project and want to add TypeScript, follow these steps:
- Install TypeScript and the necessary type definitions:
- Rename your React files from
.js
to.tsx
. - Create a
tsconfig.json
file in your project root by running:
npm install typescript @types/react @types/react-dom @types/node
npx tsc --init
TypeScript is now set up in your React project, allowing you to use types throughout.
Defining Components in TypeScript
One of the first things you'll do in React with TypeScript is define components with proper typing. Here's how you can define both function and class components.
1. Functional Components
A functional component is the most common type of component in modern React. With TypeScript, you can define the component’s props using an interface or type.
import React from 'react';
type ButtonProps = {
label: string;
onClick: () => void;
};
const Button: React.FC<ButtonProps> = ({ label, onClick }) => {
return (
<button onClick={onClick}>{label}</button>
);
};
export default Button;
Explanation
ButtonProps
defines the types of thelabel
andonClick
props.React.FC
(orReact.FunctionComponent
) is a type for function components, providing typing for the component’s props and returning JSX.Element.
2. Class Components
Although functional components are preferred in modern React, class components are still in use. Here’s how to type a class component in TypeScript:
import React, { Component } from 'react';
interface CounterProps {
initialCount: number;
}
interface CounterState {
count: number;
}
class Counter extends Component<CounterProps, CounterState> {
state: CounterState = {
count: this.props.initialCount,
};
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
export default Counter;
Explanation
- The
CounterProps
an interface defines the expectedinitialCount
prop, whileCounterState
defines the state. - The component’s state is initialized with the
initialCount
prop, and theincrement
method updates the state when the button is clicked.
Read More - Typescript Interview Questions And Answers For Freshers |
Typing Props and State
Typing Props and state are the main data inputs for React components. TypeScript ensures that you always pass and manage data correctly by defining types for both.
1. Optional and Default Props
- You can make props optional by using
?
in the type definition:
import React from 'react';
type CardProps = {
title: string;
description?: string;
};
const Card: React.FC = ({ title, description }) => {
return (
{title}
{description || 'No description available'}
);
};
export default Card;
Explanation
- The
CardProps
type definestitle
as a required string anddescription
as an optional string. - If no
description
is passed, the text "No description available" will be displayed.
2. Typing Event Handlers
- Event handlers in React, like
onClick
oronChange
, can also be strongly typed using TypeScript:
import React from 'react';
const handleClick = (event: React.MouseEvent) => {
console.log('Button clicked!', event);
};
const MyButton: React.FC = () => {
return Click Me;
};
export default MyButton;
Explanation
- The
React.MouseEvent
type ensures that thehandleClick
function only accepts click events from a button. - When the button is clicked, the event details are logged into the console.
Using TypeScript with React Hooks
React hooks like useState
and useEffect
are widely used in functional components. TypeScript provides type safety even when using hooks.
1. Typing useState
The useState
hook can be typed based on the expected type of the state value:
import React, { useState } from 'react';
const Counter: React.FC = () => {
const [count, setCount] = useState(0);
return (
Count: {count}
setCount(count + 1)}>Increment
);
};
export default Counter;
Explanation
- The
useState
hook is typed to accept only numbers, ensuring thatcount
is always a number. - Clicking the button increments the value of
count
by 1.
2. Typing useEffect
The useEffect
hook doesn’t typically need typing, but you can add types for the values it uses, especially for async functions:
import React, { useState, useEffect } from 'react';
const DataFetcher: React.FC = () => {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
const response = await fetch('/api/data');
const result = await response.text();
setData(result);
};
fetchData();
}, []);
return Data: {data || 'Loading...'};
};
export default DataFetcher;
Explanation
- The
useState
hook is used to manage the state of the fetched data, and it can either be a string ornull
. - Inside
useEffect
, we define an async function to fetch data from an API and update the state once the data is fetched.
Read More: Top 50+ React Interview Questions & Answers |
Summary
This React typescript tutorial shows React with TypeScript combines the best aspects of both tools to provide a very efficient and type-safe environment for building large-scale online projects. Using TypeScript's static typing and React's component-based architecture, code may be created in a clean, manageable, and error-resistant manner.
Whether you're working with simple components or complex applications, adopting TypeScript in your React projects helps improve developer experience, reduces bugs, and makes your codebase easier to scale and maintain. Unlock the power of TypeScript with React! Enroll in ScholarHat's TypeScript Programming Course and start building robust, scalable web applications today.
FAQs
Take our Typescript skill challenge to evaluate yourself!
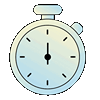
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.