24
JanVariable in TypeScript
Understanding Variables in TypeScript
Variables in TypeScript...! You might be familiar with variables from other programming languages, but In TypeScript variable provides several additional capabilities that help you write safer and more maintainable code. So what precisely are variables? Simply speaking, they are containers for holding data values, such as numbers, texts, or even more complicated structures. TypeScript provides several ways to define these variables, including let, const, and var, each with its own distinct behavior and scope.
So in this TypeScript Tutorial, We are gonna explore Variables in TypeScript including its types and examples.
What Are Variables?
Variables are containers that hold data values. In TypeScript, you can think of them as named pointers to a memory region containing a specific value. A variable's value can change during the execution of a program.1
Declaring Variables in TypeScript
In TypeScript, variables can be declared using three keywords: let
, const
, and var
. Each keyword has its own scope and behavior.
1. let
The let
keyword allows you to declare block-scoped variables. This means that the variable is only accessible within the block in which it was defined (such as within a function or an if statement).
let x: number = 10;
if (true) {
let x: number = 20; // Different x
console.log(x); // Output: 20
}
console.log(x); // Output: 10
Output
20
10
In this example, the first x
is defined outside the block and retains its value, while the x
inside the block shadows the outer variable.
2. const
The const
keyword is used to declare variables that are read-only, meaning their values cannot be reassigned after their initial definition. Similar to let
, const
variables are block-scoped.
const PI: number = 3.14;
// PI = 3.14159; // This will cause an error: Cannot assign to 'PI'
console.log(PI); // Output: 3.14
Output
3.14
Here, attempting to reassign a new value to PI
will result in a compile-time error.
3. var
The var
keyword declares variables that are function-scoped or globally scoped, which can lead to unexpected behaviors due to hoisting. Hoisting means that variable declarations are moved to the top of their containing scope during compilation.
function example() {
if (true) {
var y: number = 30; // y is function-scoped
}
console.log(y); // Output: 30
}
example();
Output
30
In this case, the variable y
is accessible outside the if
block because it is function-scoped.
Read More - Typescript Interview Questions And Answers |
Types of Variables
TypeScript supports various types of variables, allowing developers to specify the type of data a variable can hold. This feature provides better type safety and enables the TypeScript compiler to catch potential errors.
1. Basic Types
Some of the basic types include:
- Number: Represents numeric values (both integers and floating-point).
let age: number = 25;
- String: Represents textual data.
let name: string = "John Doe";
- Boolean: Represents a value that can be either
true
orfalse
.let isStudent: boolean = true;
2. 3Arrays and Tuples
You can also declare arrays and tuples in TypeScript:
- Array in Typescript: Represents a collection of items of the same type.
let numbers: number[] = [1, 2, 3, 4];
- Tuple in Typescript: Represents an array with fixed sizes and known data types for each index.
let user: [string, number] = ["Alice", 30];
3. Enums
Enums allow you to define a set of named constants, providing a way to work with a set of related values.
enum Direction {
Up,
Down,
Left,
Right,
}
let move: Direction = Direction.Up; // move will be 0
Best Practices for Using Variables
- Use
const
by Default: Unless you know the value of a variable needs to change, useconst
to promote immutability. This helps prevent accidental changes to variables. - Limit Scope with
let
: Uselet
for variables that may change, and keep their scope as narrow as possible. This enhances readability and maintainability. - Type Annotations: Always provide type annotations for better type safety. This will help you catch errors early during development.
- Avoid
var
: Prefer usinglet
andconst
overvar
to avoid hoisting issues and unexpected behaviors. - Descriptive Names: Use meaningful variable names to make your code self-documenting. For example, use
studentCount
instead ofx
ory
.
Variable Statement
In TypeScript, a variable statement is extended to include optional type annotations.
Syntax
: = ;
: ;
1. Block Scoping
let supports block scope. When you define, a variable using let within a block like if statement, for loop, switch statement, etc., the block-scoped variable is not visible outside of its containing block.
block.js
function foo(flag: boolean) {
let a = 100;
//if block
if (flag) {
// 'a' exists here
let b = a + 1;
return b;
}
// Error: 'b' doesn't exist here
return b;
}
2. Hoisting
When you use var to declare a variable, you have to deal with hoisting. Hoisting is JavaScript's default behavior of moving variable declarations to the top of the current scope (i.e. top of the current js file or the current function). It means, in JavaScript, a variable can be used before it has been declared.
hoisting.js
var x = "Gurukulsight";
function foo() {
console.log(x); //Gurukulsight
}
function bar() {
console.log(x); //undefined
var x; //x declaration is hoisted, that’s why x is undefined
}
foo();
bar();
In the above bar function declaration of the x variable will move to the top of the bar function variable declaration and within the bar function, we have not specified any value to x, hence its default value is undefined.
Important Information
JavaScript only hoists variable declarations, not variable initializations.
To avoid issues because of hoisting, always declare all variables at the beginning of every scope.
Use ES6 - let and const to avoid hoisting.
var x = "Gurukulsight";
function bar() {
console.log(x); //Error: x is not defined
let x; //x declaration is not hoisted
}
bar();
3. Destructuring
Destructuring means breaking up the structure. Destructuring is a way to quickly extract the data from a single object or array and assign it to multiple variables within a single statement. ES6 also supports object destructuring.
TypeScript supports the following forms of Destructuring:
Object Destructuring
Array Destructuring
destructuring.ts
let list = ['one', 'two', 'three'];
let [x, y, z] = list; // Destructuring the array values into three variables x, y, z
console.log(x); // 'one'
console.log(y); // 'two'
console.log(z); // 'three'
let obj = { x: 'one', y: 'two', z: 'three' };
// Use different variable names to avoid conflict
let { x: objX, y: objY, z: objZ } = obj; // Destructuring the object properties into objX, objY, objZ
console.log(objX); // 'one'
console.log(objY); // 'two'
console.log(objZ); // 'three'
Output
one
two
three
one
two
three
Destructuring can also be used for passing objects into a function that allows you to extract specific properties from an object.
destructuring_auguments.ts
function greet(
{ firstName, lastName }:
{ firstName: string; lastName: string }): void {
console.log(`Hello, ${firstName} ${lastName}!`);
}
let p1 = { firstName: 'Shailendra', lastName: 'Chauhan' };
let p2 = { firstName: 'Kanishk', lastName: 'Puri' };
greet(p1); // -> Hello, Shailendra Chauhan!
greet(p2); // -> Hello, Kanishk Puri!
Output
Hello, Shailendra Chauhan!
Hello, Kanishk Puri!
Conclusion
FAQs
Take our Typescript skill challenge to evaluate yourself!
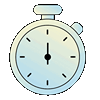
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.