21
NovFile Handling In Python
Introduction to File Handling in Python
File handling is an essential concept in Python's programming framework. It enables you to use your Python code directly to create, read, write, and manage files. The ability to handle data storage, retrieval, and modification in a flexible manner facilitates the management and processing of huge datasets.
In this Python Tutorial, we will discuss file handling, including "What is File Handling in Python?" and when to utilize it. Additionally, file handling in Python will be covered with examples. Let's begin by talking about "What is File Handling?"
What is File Handling?
- A file is a named location used for storing data. Python has file-handling functionality, enabling users to read, write, and perform various other file-handling operations on files.
- Although the idea of file handling has been extended to many other languages, its implementation is typically difficult or time-consuming. However, this idea is simple to understand, just like other Python concepts.
- Python handles text and binary files differently, and this is significant. A text file is formed by the characters that make up each line of code.
- A unique character known as the End of Line (EOL) character, such as the comma {,} or newline character, ends each line in a file. It informs the interpreter that a new line has begun and ends the existing one.
Understanding Types of Files in Python
It's essential to understand the two main file formats in Python when working with files:
1. Text files:
- Typically ending in.txt, these files contain data in a readable format for humans to access.
- Any text editor can be used to edit the plain text that they contain.
- Text files can be handled in Python utilizing modes like a for appending, w for writing, and r for reading.
2. Binary Files:
- Unlike text files, binary files contain data like pictures, films, or computer programs that are not readable by humans. .bin or.dat are typical extensions for them.
- Similar to text files, binary files are handled by Python with the addition of a b suffix, such as rb for reading binary files and wb for writing them.
Understanding these file formats helps you select appropriate techniques for data reading and writing, ensuring effective file management in your Python programs.
Python File Operations
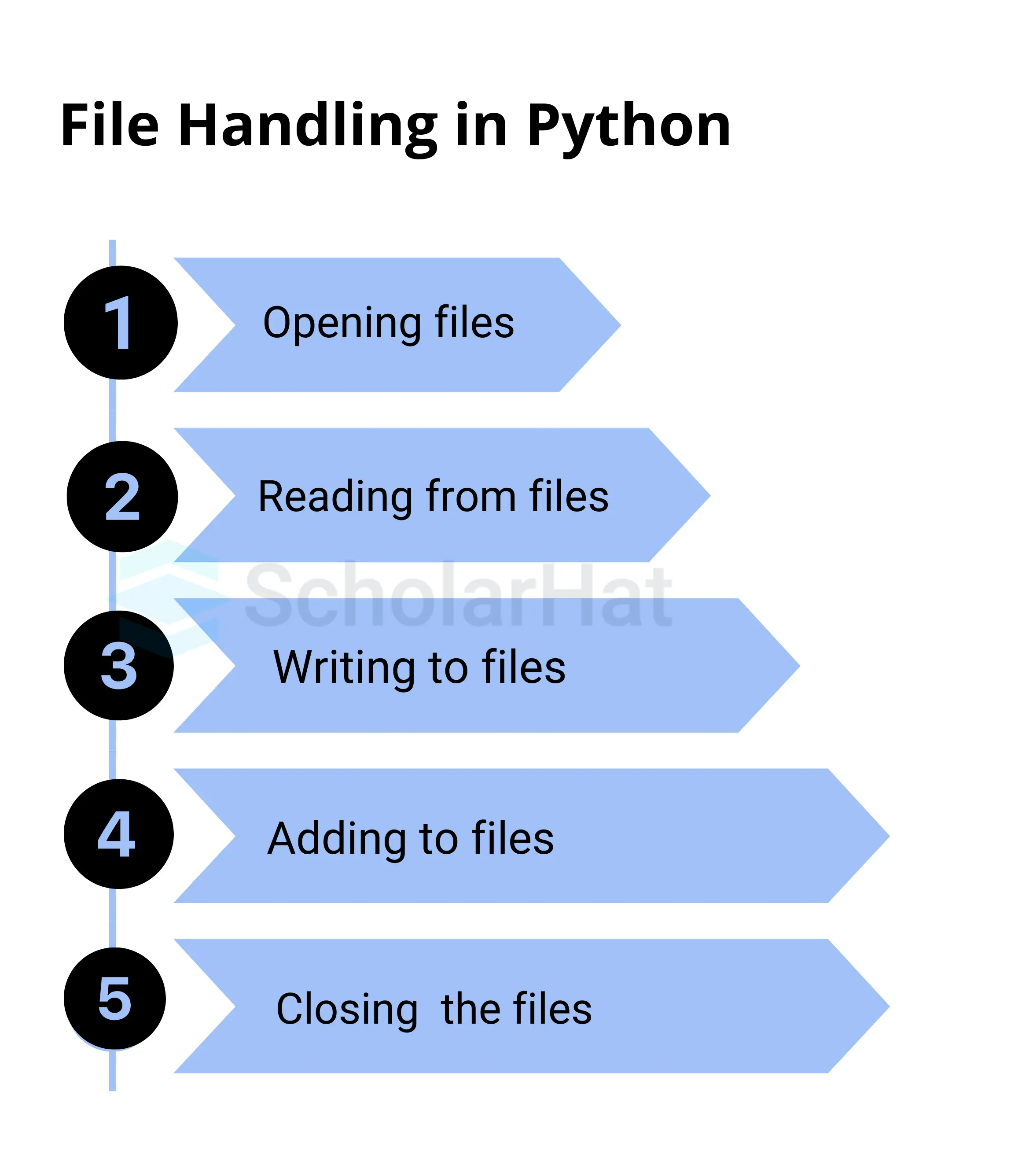
For this article, we will consider the following “Scholarhat.txt” file as an example.
Welcome to Python
Learn with ScholarHat
789 101
Opening a file
- Opening a file is the first step in doing any activity on it, whether writing or reading.
- The open() function in Python is used for this, and it returns a file object that lets you work with the file.
- However, we must define the mode that represents the purpose of the opening file.
f = open(filename, mode)
Wherever the mentioned mode is offered:
- r: Allows you to read an existing file. An error happens if the file is missing.
- w: Allows writing to an open file. Data in the file will be removed if it already exists. If the file doesn't exist, a new one is created.
- a: Opens a file to add data at the end. It keeps the info that already exists.
- r+: Allows you to read and write to a file. You can make changes to the file from the beginning without deleting any already-existing content.
- w+: Allows you to write and read data in a file. It erases any existing data. If the file doesn't exist, it creates a new one.
- a+: Allows you to read and append files. It adds data to the end without erasing existing content.
Working in Read mode
1. Opening the File:
file = open("Scholarhat.txt", "r")
Reading Content:
You can read the entire content or specific parts of the file using methods like read(), readline(), or readlines()content = file.read()
print(content)
line = file.readline()
print(line)
lines = file.readlines()
print(lines)
file.close()
Example
with open("Scholarhat.txt", "r") as file:
content = file.read()
print(content)
Example
file.write("Welcome to Python\n")
file.write("Learn with ScholarHat\n")
file.write("789 101\n")
Example using "with()" method
with open("Scholarhat.txt", "w") as file:
file.write("Welcome to Python\n")
file.write("Learn with ScholarHat\n")
file.write("789 101\n")
Working of Append Mode
Example
file = open("Scholarhat.txt", "a")
file.write("Python is versatile.\n")
file.write("Expand your skills with ScholarHat.\n")
file.close()
Explanation
This code uses the open("Scholarhat.txt", "a") function to open the file in append mode, allowing you to add new content to the end of the file without damaging existing data. The write() function is then used to add two additional lines of text. Finally, the file is closed by calling the file. close() to save the modifications.Closing Files in Python
After completing any file activity, use the close() function to close the file. This ensures that all modifications are stored while clearing up system resources. If you do not close the file, you may experience problems such as data not being stored properly or running out of file handles in your software.Example
file = open("Scholarhat.txt", "w")
file.write("This is a new line.\n")
file.close() # Closes the file and saves changes
Implementing all the functions in File Handling
Example
import os
def create_file(filename):
try:
with open(filename, 'w') as f:
f.write('Welcome to ScholarHat!\n')
print("File " + filename + " created successfully.")
except IOError:
print("Error: could not create file " + filename)
def read_file(filename):
try:
with open(filename, 'r') as f:
contents = f.read()
print(contents)
except IOError:
print("Error: could not read file " + filename)
def append_file(filename, text):
try:
with open(filename, 'a') as f:
f.write(text)
print("Text appended to file " + filename + " successfully.")
except IOError:
print("Error: could not append to file " + filename)
def rename_file(filename, new_filename):
try:
os.rename(filename, new_filename)
print("File " + filename + " renamed to " + new_filename + " successfully.")
except IOError:
print("Error: could not rename file " + filename)
def delete_file(filename):
try:
os.remove(filename)
print("File " + filename + " deleted successfully.")
except IOError:
print("Error: could not delete file " + filename)
if __name__ == '__main__':
filename = "Scholarhat.txt"
new_filename = "Scholarhat_Updated.txt"
create_file(filename)
read_file(filename)
append_file(filename, "Expanding knowledge with ScholarHat.\n")
read_file(filename)
rename_file(filename, new_filename)
read_file(new_filename)
delete_file(new_filename)
Output
File Scholarhat.txt created successfully.
Welcome to ScholarHat!
Text appended to file Scholarhat.txt successfully.
Welcome to ScholarHat!
Expanding knowledge with ScholarHat.
File Scholarhat.txt renamed to Scholarhat_Updated.txt successfully.
Welcome to ScholarHat!
Expanding knowledge with ScholarHat.
File Scholarhat_Updated.txt deleted successfully.
Explanation
Working with Binary Files in Python
Example
# Step 1: Writing to a Binary File
file = open("example.bin", "wb") # Open the file in write-binary mode
file.write(b'\xDE\xAD\xBE\xEF') # Write some binary data to the file
file.close() # Close the file to save changes
# Step 2: Reading from the Binary File
file = open("example.bin", "rb") # Open the file in read-binary mode
content = file.read() # Read the content of the file
print(content) # Output: b'\xde\xad\xbe\xef'
file.close() # Close the file after reading
# Step 3: Appending to the Binary File
file = open("example.bin", "ab") # Open the file in append-binary mode
file.write(b'\xBA\xAD\xF0\x0D') # Append more binary data to the file
file.close() # Close the file to save changes
# Step 4: Reading Again to Verify
file = open("example.bin", "rb") # Open the file again in read-binary mode
content = file.read() # Read the updated content of the file
print(content) # Output: b'\xde\xad\xbe\xef\xba\xad\xf0\x0d'
file.close() # Close the file after the final read
Explanation
- Step 1: First, open the file "example.bin" in write-binary mode to create it and write binary data b'\xDE\xAD\xBE\xEF'. The changes are then saved by closing the file.
- Step 2: It involves reopening the file in read-binary mode to read and print the content, which displays the previously written data.
- Step 3: It is reopening the file in append-binary mode to include more data b'\xBA\xAD\xF0\x0D', then closing it to save the modifications.
- Step 4: Finally, in Step 4, the file is reopened in read-binary mode to ensure that the modified content includes both the original and appended data.
Handling File Exceptions in Python
Example
try:
file = open("Scholarhat.txt", "r") # Open the file in read mode
read_content = file.read() # Attempt to read the file content
print(read_content) # Print the file content
finally:
file.close() # Ensure the file is closed, even if an error occurs
Output
//Content of the file
Welcome to Python
Learn with ScholarHat
789 101
If "Scholarhat.txt" Does Not Exist:
Error: could not read file Scholarhat.txt
Explanation
Summary
Python file-handling functionality allows you to interact with files on your computer. Python allows you to create, read, write, and modify files. This is important for things like saving data, reading configuration files, and logging information. There are two types of files: text files (human-readable) and binary files. Python's file management processes include open(), read(), write(), and close(). It is essential to use the 'with' statement or 'try...finally' block to guarantee that files are correctly closed after use. Also, consider our Python Programming For Beginners Free course for a better understanding of other Python concepts.FAQs
- Text files are used to store human-readable data by separating characters with line breaks.
- Binary files store data in a machine-readable format that is not directly interpretable by humans.
- Other file types exist (e.g., CSV, JSON), but they are all variations on text or binary files.
- Data Persistence: Data is saved after the software has finished running, making it available later.
- Efficiency: Handles huge datasets effectively, resulting in improved application performance.
- Reusability: Data can be reused across multiple program runs and functions.
- Organization: Enables structured storage and retrieval of information.
- Portability: Allows for the easy transmission of data between systems.
Files are digital storage boxes. You can insert information into them and save it for later. Python allows you to enter these boxes, read what's inside, create new objects, and even change what's within. It's similar to having a place to keep your digital files safe and organized.