21
NovAn Easy Solution for Array Methods in JavaScript
Array Methods in JavaScript
Array Methods in JavaScript are the predefined built-in JavaScript functions in the JavaScript library. These methods help programmers enable effective array manipulation and traversal. They also provide Important features that improve code readability and efficiency, including adding, deleting, and modifying items, as well as searching, sorting, and iterating across array elements.
In the JavaScript tutorial, we will explore what are the array methods in JavaScript?, types of Array methods, basic Array methods, manipulation methods, access and search methods, iteration methods, transformation and reduction methods, sorting and reversing methods, conversion methods, features and best practices of Array methods in JavaScript, and many more.
What are Array methods in JavaScript?
Array methods in JavaScript are strong tools that let programmers effectively read, modify, and change array items. These techniques make it simpler to handle data sets by offering features for adding, deleting, sorting, and filtering items. They are necessary to execute a variety of tasks in JavaScript arrays.
Read More: |
Introduction to JavaScript |
How to Become a Full-Stack JavaScript Developer? |
Java Arrays: Single-Dimensional and Multi-Dimensional Arrays |
Types of Array methods in JavaScript
For better learning, we have categorized the Array methods in JavaScript in 6 ways that will cover all specified methods on their own and help you to clear your concept:
- Basic Array Methods
- Manipulation Methods
- Access and Search Methods
- Iteration Methods
- Transformation and Reduction Methods
- Sorting and Reversing Methods
- Conversion Methods
Let's understand each of the above methods properly;
1. Basic Array Methods
Several basic Array methods in Javascript are:
1. Array length in JavaScript
The array length method in JavaSript provides the length of the given array.
Syntax
Array.length
Example
// declaring an array name fruit
let fruits = ['papaya', 'grapes', 'orange'];
//print the length of an array
console.log(fruits.length);
Output
3
2. Array toString() in JavaScript
The array toString() method in Javascript converts an array to a string of comma-separated elements.
Syntax
array.toString()
// declare an array of fruits
let fruits = ['apple', 'banana', 'orange'];
//using toString method
console.log(fruits.toString());
Output
apple,banana,orange
3. Array join() in JavaScript
The array join() method in JavaScript combines all elements into a single string in JavaScript with a specified separator.
Syntax
array.join(separator)
Example
//declare an arrayof fruits
let fruits = ['apple', 'banana', 'orange'];
//using join() method with seprater
console.log(fruits.join(' - '));
Output
apple - banana - orange
Read More: Difference between Inner Join and Equi Join and Natural Join |
2. Manipulation Methods
Several Manipulation Array methods in Javascript are:
1. Array push() in JavaScript
The push() method in JavaScript adds one or more elements to the end of an array.
Syntax
Array.push(item1, item2 …)
Example
//declaring an array of fruits
let fruits = ['apple', 'banana'];
//adding new element by push()
fruits.push('orange');
console.log(fruits);
Output
['apple', 'banana', 'orange']
2. Array pop() in JavaScript
The pop() method in JavaScript removes the last element from an array.
Syntax
Array.pop()
Example
//declare an array of fruits
let fruits = ['apple', 'banana', 'orange'];
//Removing the last element using pop()
fruits.pop();
console.log(fruits);
Output
['apple', 'banana']
3. Array shift() in JavaScript
The shift() in JavaScript removes the first element from an array.
Syntax
Array.shift()
Example
// declare an array name fruits
let fruits = ['apple', 'banana', 'orange'];
//using shift() to remove the first element of an array
fruits.shift();
console.log(fruits);
Output
['banana', 'orange']
4. Array unshift() in JavaScript
The unshift() in JavaScript adds one or more elements to the beginning of an array.
Syntax
Array.unshift(item1, item2 …)
Example
//Declare an array of fruits
let fruits = ['banana', 'orange'];
// using unshift() method to add element in array
fruits.unshift('apple');
console.log(fruits);
Output
['apple', 'banana', 'orange']
5. Array splice() in JavaScript
The splice() in JavaScript adds and removes elements at any position in the array.
Syntax
Array.splice (start, deleteCount, item 1, item 2….)
Example
//Declare an array name fruits
let fruits = ['apple', 'banana', 'orange'];
// using splice() method to add and remove element
fruits.splice(1, 1, 'mango', 'pineapple');
console.log(fruits);
Output
['apple', 'mango', 'pineapple', 'orange']
6. Array concat() in JavaScript
The concat() method in JavaScript merges one or two arrays into one array.
Syntax
let newArray = arr.concat() // or
let newArray = arr1.concat(arr2) // or
let newArray = arr1.concat(arr2, arr3, ...) // or
let newArray = arr1.concat(value0, value1)
Example
//Declare two arrays fruits and more fruits
let fruits = ['apple', 'banana'];
let moreFruits = ['orange', 'mango'];
//use concat() method to merge theem
let allFruits = fruits.concat(moreFruits);
console.log(allFruits);
Output
['apple', 'banana', 'orange', 'mango']
7. Array slice() in JavaScript
The slice() method in JavaScript returns a shallow copy of a portion of an array.
Syntax
Array.slice (startIndex , endIndex);
Example
//declare an array name fruits
let fruits = ['apple', 'banana', 'orange', 'mango'];
//declare an array citrus using slice() method
let citrus = fruits.slice(1, 3);
console.log(citrus);
Output
['banana', 'orange']
3. Access and Search Methods
Several access and search methods in Javascript are:
1. Array indexOf() in JavaScript
The indexOf() method in JavaScript finds the first occurrence of an element.
Syntax
Array.indexOf(searchElement, fromIndex)
Example
//declare an array fruts
let fruits = ['apple', 'banana', 'orange'];
//using indexOf to print occurrence
console.log(fruits.indexOf('banana'));
//declare an array startFromIndex
//print occurrence of element using index
let startFromIndex = fruits.indexOf('banana', 1);
console.log(startFromIndex);
Output
1
1
2. Array lastIndexOf() in JavaScript
The lastIndexOf() in JavaScript finds the last occurrence of an element.
Syntax
array.lastIndexOf(searchElement, fromIndex)
Example
//declare am array fruits
let fruits = ['apple', 'banana', 'apple'];
//use lastIndexOf() for occurrence of last element.
console.log(fruits.lastIndexOf('apple'));
//declare an array reference by fruits
//and find occurrence
let startFromIndex = fruits.lastIndexOf('banana', 1);
console.log(startFromIndex);
Output
2
1
3. Array includes() in JavaScript
The includes() in JavaScript checks if an array contains a specific value.
Syntax
array.includes(searchElement, fromIndex)
Example
// Declare an array fruits
let fruits = ['apple', 'banana', 'orange'];
// check element by includes()
console.log(fruits.includes('banana'));
Output
True
4. Iteration Methods
Several iterations methods in Javascript are:
1. Array forEach() in JavaScript
The forEach() in JavaScript executes a function for each array element.
Syntax
array.forEach(function(currentValue, index, arr), thisValue)
Example
let fruits = ['apple', 'banana', 'orange'];
fruits.forEach((fruit) => console.log(fruit));
Output
apple
banana
orange
2. Array map() in JavaScript
The map() in JavaScript creates a new array by applying a function to each element.
Syntax
array.map(callback(currentValue, index, array), thisArg);
Example
// declare an array numbers
let numbers = [1, 2, 3];
// using map() to print the result
let doubled = numbers.map((num) => num * 2);
console.log(doubled);
Output
[2, 4, 6]
2. Array filter() in JavaScript
The filter() in JavaScript returns a new array with elements that pass a test.
Syntax
Array.filter(callback(element, index, array), thisArg)
Example
let numbers = [1, 2, 3, 4, 5]; // Array of numbers
// Filter out even numbers from the array
let even = numbers.filter((num) => num % 2 === 0);
console.log(even);
Output
[2, 4]
3. Array every() in JavaScript
Syntax
array.every(callback(element, index, array), thisArg)
Example
//declare an array of numebrs
let numbers = [1, 2, 3, 4];
// use every() method to check the condition
let allEven = numbers.every((num) => num % 2 === 0);
console.log(allEven);
Output
false
3. Array some() in JavaScript
Syntax
array.some(callback(element, index, array), thisArg)
Example
let numbers = [1, 2, 3, 4]; // Array of numbers
// Check if there's at least one even number
let someEven = numbers.some((num) => num % 2 === 0);
console.log(someEven);
Output
true
5. Transformation and Reduction Methods
Several transformation and reduction methods in Javascript are:
1. Array reduce() in JavaScript
The reduce() in JavaScript reduces the array to a single value by applying a function.
Syntax
array.reduce(callback(accumulator, currentValue, index, array), initialValue);
Example
//Declare an array of numbers
let numbers = [1, 2, 3, 4];
//using reduce() method to find the sum
let sum = numbers.reduce((total, num) => total + num, 0);
console.log(sum);
Output
10
2. Array reduceRight() in JavaScript
The reduceRight() in JavaScript issimilar to reduce()but works from right to left.
Syntax
array.reduceRight(callback(accumulator, currentValue, index, array), initialValue)
Example
//declare an array of numbers
let numbers = [1, 2, 3, 4];
// using reduceRight() to find the sum
let sum = numbers.reduceRight((total, num) => total + num, 0);
console.log(sum);
Output
10
3. Array flat() in JavaScript
The flat() in JavaScript flattens nested arrays into a single array.
Syntax
arr.flat([depth])
Example
//Declare an array of arrays
let arr = [1, [2, [3, [4]], 5]];
let flatArr = arr.flat(2); //using flat() method
console.log(flatArr);
Output
[1, 2, 3, [4], 5]
4. Array flatMap() in JavaSCript
The flatMap() in JavaScript combines mapping and flattening into a single method.
Syntax
array.flatMap(callback(element, index, array), thisArg)
Example
//declare an array name arr
let arr = [1, 2, 3];
let mapped = arr.flatMap((num) => [num, num * 2]); // using flatMap() method
console.log(mapped);
Output
[1, 2, 2, 4, 3, 6]
6. Sorting and Reversing Methods
Several sorting and reversing Methods in Javascript are:
1. Array sort() in JavaScript
The sort() in JavaScript sorts the elements of an array.
Syntax
array.sort(compareFunction)
Example
//declare an array name fruits
let fruits = ['banana', 'apple', 'orange'];
fruits.sort(); //using sort() method to arrange an array
console.log(fruits);
Output
['apple', 'banana', 'orange']
2. Array reverse() in JavaScipt
The reverse() in JavaScript reverses the order of the elements in an array.
Syntax
array.reverse()
Example
// Declare an array fruits
let fruits = ['apple', 'banana', 'orange'];
fruits.reverse(); // use reverse() mthod to reverse the array
console.log(fruits);
Output
['orange', 'banana', 'apple']
Compare with: How To Reverse A String In Java: Explained |
7. Conversion Methods
In the conversion method, there are two methods that we have discussed before:
- array. join()
- array.toString()
Read More: |
JavaScript Developer Salary |
JavaScript Interview Questions and Answers (Fresher + Experience) |
Conclusion
FAQs
Take our Javascript skill challenge to evaluate yourself!
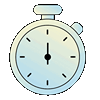
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.