02
MayFunctions in JavaScript: User-Defined and Standard Library Functions
JavaScript Functions
Functions are an essential segment of JavaScript programming. All programming languages start their basics with functions because they help developers break down complex problems into easy ones and make the code cleaner and more efficient.
In this JavaScript tutorial, we will learn about the crucial fundamentals of JavaScript functions, including function expressions, invocations, calling, return statements, and arrow functions. To gain a deeper understanding and practical experience, you can enroll in a Free JavaScript Course With Certificate. This course will help you master JavaScript functions and their various features, enhancing your coding expertise.
Read More: JavaScript Interview Questions and Answers (Fresher + Experience) |
What is a Function in JavaScript?
A function in JavaScript is a block of code written by the developer to perform a definite task, such as multiplying two numbers. It wraps a set of instructions that can be used throughout the program and is executed when it is called. By using functions, we can enhance our program's reusability and readability.
There are two types of functions in JavaScript:
- User-defined Functions: Created by users
- Standard Library Functions: Inbuilt functions in JavaScript
Here, we will look at User-defined and Standard library functions in JavaScript.
1. User-Defined Functions in JavaScript
In JavaScript, a programmer can create any function to perform any task, e.g., a function to calculate the area of a square. A user-defined function groups code to perform a specific task and that group of code is given a name (identifier). When a programmer invokes his created function in any part of the program, the body of that function executes.
There are some aspects of a user-defined function. They are
- Function Declaration
- Function Invocation
- Function Definition
- Function Parameters and Arguments
- Function Return
1. Function Declaration In JavaScript
To create a function in JavaScript, we should remember some essential points.
- When creating a function in JavaScript, we should first use the function keyword.
- After spacing, we should give the function name, which should be unique, and parameters within parenthesis ( ).
- The body of the function is inside the curly braces { }.
Syntax of JavaScript Function
function fun_Name() {
// code of body
}
Example of JavaScript function
function myFunc() {
console.log("Hello, World!");
}
Explanation
Here, we have declared a function named myFunc() that prints the result Hello, World!. Each part of our function is below.
- Function Keyword- The function keyword is used to declare or create a function.
- Function Name- myFunc is the function name, followed by parenthesis ( ).
- Function Body- console.log("Hello, World!") is the body of the function. It is executed when we call the function.
2. Function Invocation in JavaScript
In JavaScript, function invocation is the method to call or execute a function. You can call a function many times if you want. Function invocation happens when-
- It is triggered by an event (when a user hits a button )
- It is called directly by JavaScript code.
- It also can be called by itself (self-invoked).
Syntax of Function Invocation
function fun_Name() {
// code to be executed
}
//calling or invoking the function
fun_Name();
Example of Function Invocation
function myFunc(){
console.log("Hello, World!");}
// call or invoke the function
myFunc();
Output
Hello, World!
Explanation
In this example, we declare a function named myFunc(). Here's how the control of the program flows.
- When myFunc() is called, the program control follows the function definition.
- All the code inside the function is executed, and it prints the result: Hello, World!
3. Function Definition in JavaScript
In JavaScript, the function definition is the body of a function that contains information about the task and is executed when it is called. It is always inside the curly Braces { }.
Example of Function Definition
function myFunc() {
console.log("Hello, World!"); // function definition
}myFunc();
Output
Hello, World!
Explanation
In the above example, we have declared and called a function named myFunc(). It executes the function definition and prints the result: Hello, World!
4. Function Arguments and Parameters in JavaScript
In JavaScript,
- A function argument is the value we pass to a function while calling it.
- A function parameter is a variable that stores the function arguments. It is inside the parentheses and created during function declaration.
Syntax of Function Arguments and Parameter
// here name is the parameter
function func_Name(name) {
// code of the body
}func_Name(Arguments); // function arguments
Example of Function Argument with parameter
function myFunc(name) {// function declare with parameter
console.log(`Hello, ${name}!`);
}
myFunc('Jack'); //function call with arguments
Output
Hello, Jack !
Explanation
In this example, we have declared a function named myFunc() with the parameter name.
- When myFunc is called with arguments Jack, the program controls follow the function definition.
- All the code inside the function is executed, and the results are printed with an argument. (E.g. Hello, Jack !)
Example - JavaScript Function with different arguments
function myFunc(name) {
console.log(`Hello ${name}`);
}
myFunc("Amit"); // pass "Amit" as argument
myFunc("Rakesh"); // pass "Rakesh" as argument
Output
Hello Amit
Hello Rakesh
Explanation
In the above example, we declared a function using the parameter myFunc(name).
- We called the function twice with arguments from John and David.
- It executed the function definition and printed Hello John and Hello David on the screen.
5. Function Return in JavaScript
In JavaScript, the function returns a definite value using the return statement. When a function executes and reaches the return statement, it stops the execution and sends the return value to the caller. If we don't use the"return" statement, the function returns 'undefined.'
Example of Function Return
// function to find square of a number
function findSqr(num) {
// return square
return num * num;
}
// call the function and store the result
let square = findSqr(4);
console.log(`Square: ${square}`);
Output
Square: 16

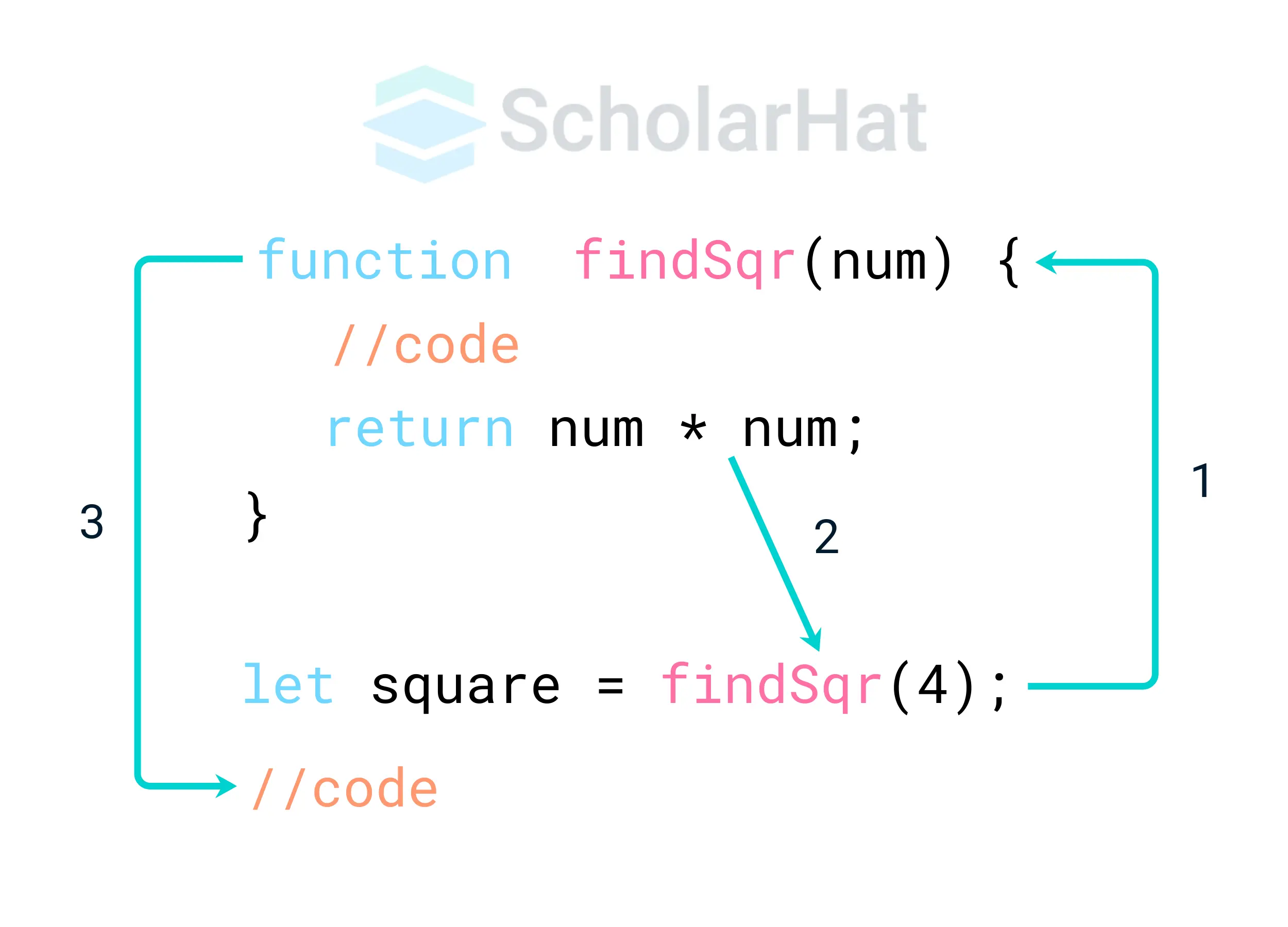
Explanation
In this example, we declare a function findSqr(num) with the parameter and call the function by passing the arguments 4.
- After calling, the return statement is used to calculate the square of the root.
- Then, we store it in a square variable and print the result.
Function Expression in JavaScript
A function expression is the same as a function declaration, but we don't need to specify the function name, and it can be stored in a variable assignment.
Function Expression Syntax
let myFunction= function(param1, param2) {
// code of the body
}
Example of Function Expression
const myFirst = function (number) {
return number * number;};
const x = myFirst(6);
console.log(x);
Output
36
Explanation
In this example, we declare a function with a parameter number without a function named and store it myFirst variable.
- After calling the function with an argument, the return statement is executed.
- We store the result in a variable x and print the result.
You can also compare JavaScript functions with
What is an Arrow function?
An Arrow function in JavaScript is a short method for writing a function expression. It was introduced in ECMA6(ES6). We use '=>' syntax to introduce the arrow function.
Example of Arrow Function
const sumNums = (a, b) => a + b;
// Calling the function
const result = sumNums(4,3);
console.log(result)
Output
7
Explanation
- Next, we call the function with arguments 4 & 3 and store it in the result variable.
- After that, print the output in the console.
2. Standard Library Functions in JavaScript
Functions | Working |
toLowerCase() | it returns the string in lowercase. |
toUpperCase | It returns the string in uppercase. |
Math.sqrt() | It calculates the square of a number. |
Math.pow() | It calculates the power of a number. |
console.log() | It prints the string in the quotation mark. |
Example For Math.sqrt() Function
let squareRoot = Math.sqrt(3);
console.log("Square Root of 3 is", squareRoot);
Output
9
Explanation
In the above example, we have directly called the inbuilt library function Math.sqrt () with arguments 3. The log statement directly prints the result on the screen.
Summary
In this article, we discuss all the crucial aspects related to the JavaScript function. While reading this article, you won't face any difficulty, and it will help you clear your concepts quickly. For a practical understanding, consider our JavaScript Programming Course.
ScholarHat provides different types of Training and Certification Courses to help you in your end-to-end product development. |
FAQs
Take our Javascript skill challenge to evaluate yourself!
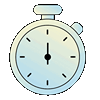
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.