21
NovWebGrid with custom paging and sorting in ASP.NET MVC
WebGrid with custom paging and sorting: An Overview
WebGrid is simply used to display the data with paging and sorting. If you want to control the default behavior of the webgrid, you need to do it manually. In this article, I would like to explore how can we do custom paging and sorting. Refer to the article Enhancing WebGrid with Ajax in MVC4 for more help. In this MVC Tutorial, we will explore more about MVC which will include webgrid in mvc3 with custom paging and sorting, customized webgrid in mvc4 mvc3, and razor webgrid template. Consider our ASP.NET MVC Course for a better understanding of all MVC core concepts.
How to do it...
The Model
First of all, design the customer model using the Entity Framework database first approach as shown below;
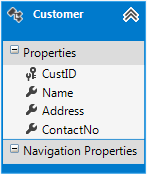
Now develop the logic for querying the data from customer table and also develop the logic for custom paging and sorting.
public class ModelServices : IDisposable
{
private readonly TestDBEntities entities = new TestDBEntities();
//For Custom Paging
public IEnumerable<Customer> GetCustomerPage(int pageNumber, int pageSize, string sort, bool Dir)
{
if (pageNumber < 1)
pageNumber = 1;
if (sort == "name")
return entities.Customers.OrderByWithDirection(x => x.Name, Dir)
.Skip((pageNumber - 1) * pageSize)
.Take(pageSize)
.ToList();
else if (sort == "address")
return entities.Customers.OrderByWithDirection(x => x.Address, Dir)
.Skip((pageNumber - 1) * pageSize)
.Take(pageSize)
.ToList();
else if (sort == "contactno")
return entities.Customers.OrderByWithDirection(x => x.ContactNo, Dir)
.Skip((pageNumber - 1) * pageSize)
.Take(pageSize)
.ToList();
else
return entities.Customers.OrderByWithDirection(x => x.CustID, Dir)
.Skip((pageNumber - 1) * pageSize)
.Take(pageSize)
.ToList();
}
public int CountCustomer()
{
return entities.Customers.Count();
}
public void Dispose()
{
entities.Dispose();
}
}
public class PagedCustomerModel
{
public int TotalRows { get; set; }
public IEnumerable<Customer> Customer { get; set; }
public int PageSize { get; set; }
}
Extension Method for OrderByWithDirection
public static class SortExtension
{
public static IOrderedEnumerable OrderByWithDirection
(this IEnumerable source,Func keySelector,bool descending)
{
return descending ? source.OrderByDescending(keySelector)
: source.OrderBy(keySelector);
}
public static IOrderedQueryable OrderByWithDirection
(this IQueryable source,Expression> keySelector,bool descending)
{
return descending ? source.OrderByDescending(keySelector)
: source.OrderBy(keySelector);
}
}
The View
Now design the view based on the above-developed model as shown below
@model Mvc4_CustomWebGrid.Models.PagedCustomerModel
@{
ViewBag.Title = "WebGrid with Custom Paging, Sorting";
WebGrid grid = new WebGrid(rowsPerPage: Model.PageSize);
grid.Bind(Model.Customer,
autoSortAndPage: false,
rowCount: Model.TotalRows);
}
@grid.GetHtml(
fillEmptyRows: false,
alternatingRowStyle: "alternate-row",
headerStyle: "grid-header",
footerStyle: "grid-footer",
mode: WebGridPagerModes.All,
firstText: "<< First",
previousText: "< Prev",
nextText: "Next >",
lastText: "Last >>",
columns: new[] {
grid.Column("CustID",header: "ID", canSort: false),
grid.Column("Name"),
grid.Column("Address"),
grid.Column("ContactNo",header: "Contact No")
}
)
The Controller
Now, let's see how to write the code for implementing the webgrid functionality using model class and methods.
public class HomeController : Controller
{
ModelServices mobjModel = new ModelServices();
public ActionResult WebGridCustomPaging(int page = 1, string sort = "custid", string sortDir = "ASC")
{
const int pageSize = 5;
var totalRows = mobjModel.CountCustomer();
bool Dir = sortDir.Equals("desc", StringComparison.CurrentCultureIgnoreCase) ? true : false;
var customer = mobjModel.GetCustomerPage(page, pageSize, sort, Dir);
var data = new PagedCustomerModel()
{
TotalRows = totalRows,
PageSize = pageSize,
Customer = customer
};
return View(data);
}
}
How it works..
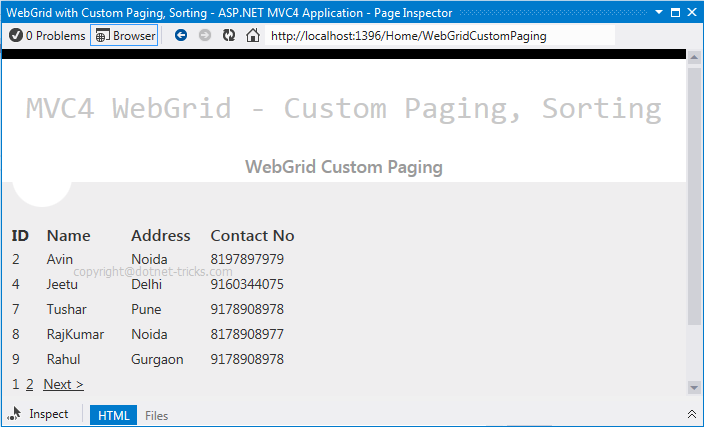
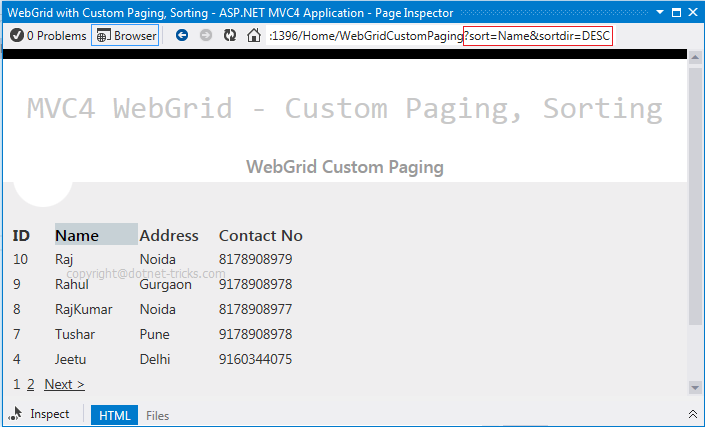